Implementing Avatar in Java EE: Overcoming Limitations
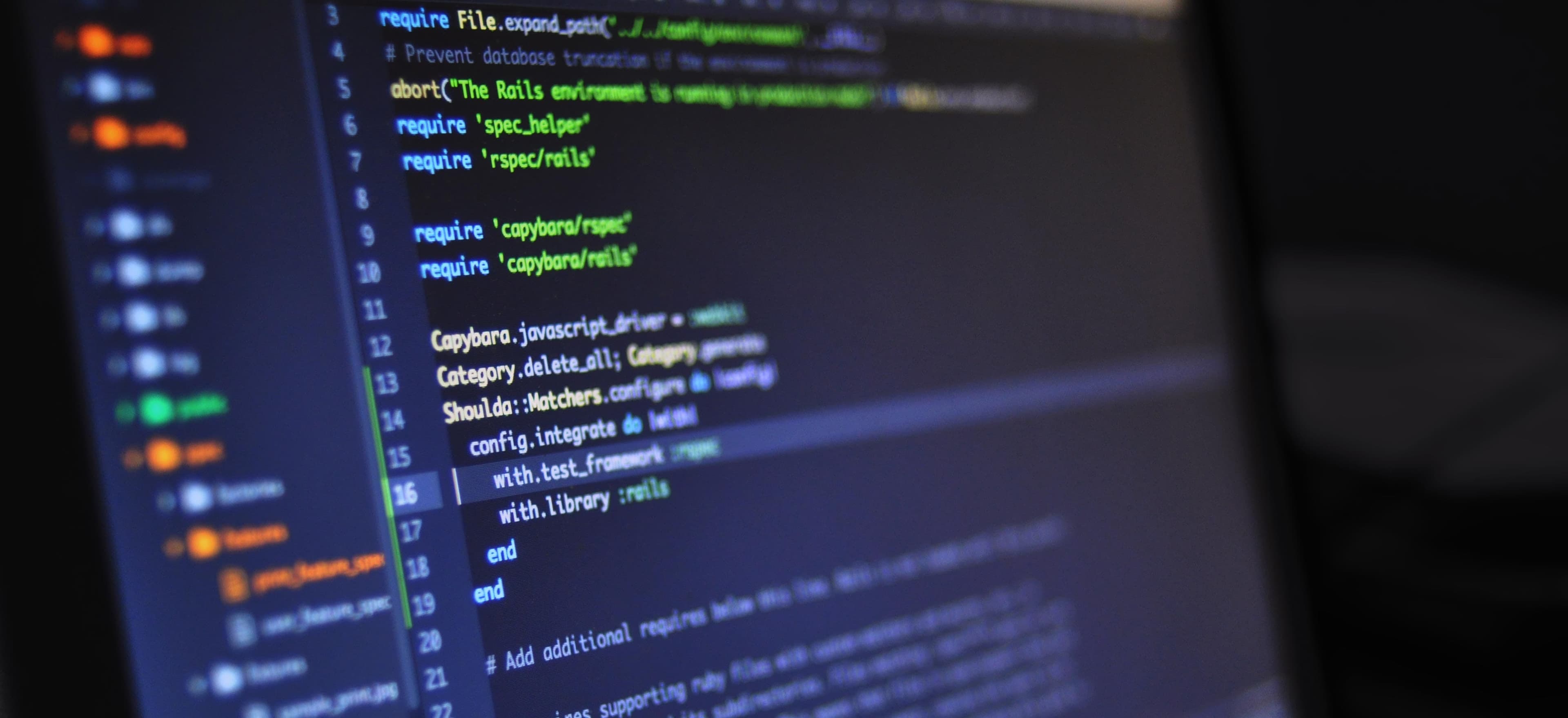
- Published on
Implementing Avatar in Java EE: Overcoming Limitations
A Quick Look
Java Enterprise Edition, commonly known as Java EE, is a robust platform for building large-scale, scalable, and secure enterprise applications. However, as technology evolves, so do the requirements for modern applications. With the advent of microservices and cloud-native architecture, developers are increasingly looking for ways to overcome the limitations of Java EE and adopt more lightweight and flexible frameworks.
Enter Project Avatar, a modern, lightweight, and modular framework designed to address the limitations of Java EE by providing a more flexible and scalable architecture for building cloud-native applications. In this blog post, we’ll explore how to implement Avatar in Java EE and overcome its limitations.
Understanding the Limitations of Java EE
Before delving into the implementation of Avatar in Java EE, it’s crucial to understand the limitations that developers often face when working with traditional Java EE applications. Some of the key limitations include:
-
Monolithic Architecture: Java EE applications are often built as monolithic applications, making it challenging to adopt a microservices architecture.
-
Resource Intensiveness: Traditional Java EE applications are resource-intensive, which can be a bottleneck when aiming for high scalability and performance.
-
Limited Flexibility: Java EE’s traditional approach lacks the flexibility needed for rapid development and easy integration with modern technologies like containers and serverless computing.
Introducing Project Avatar
Project Avatar, an open-source project led by the Oracle Corporation, is designed to address the limitations of traditional Java EE applications. Built on top of the Node.js platform, Avatar provides a lightweight and modular framework for building cloud-native applications with Java EE.
Avatar allows developers to use JavaScript and Node.js modules alongside Java EE components, enabling a more flexible and scalable architecture. By leveraging Avatar, developers can break away from the constraints of traditional Java EE and embrace modern technologies, such as microservices and serverless computing.
Implementing Avatar in Java EE
Now, let’s dive into the implementation of Avatar in a Java EE application. We’ll discuss the key steps and considerations for integrating Avatar into an existing Java EE project.
Step 1: Setting Up the Project
To get started with Avatar in Java EE, we need to set up a new project or integrate Avatar into an existing Java EE project. We can use Maven or Gradle as build tools for managing dependencies and building the project.
Here's an example pom.xml
snippet for configuring Avatar dependencies in a Maven project:
<dependencies>
<dependency>
<groupId>io.helidon.microprofile</groupId>
<artifactId>helidon-microprofile</artifactId>
<version>2.1.2</version>
</dependency>
</dependencies>
In this example, we’re adding the helidon-microprofile
dependency, which provides the necessary components for integrating Avatar into a Java EE application.
Step 2: Creating Avatar Components
Once the project is set up with Avatar dependencies, we can start creating Avatar components. Avatar components are typically written in JavaScript and can be used alongside Java EE components to build modern, cloud-native applications.
Here’s an example of creating an Avatar component to handle HTTP requests:
// avatar-component.js
var avatar = require('avatar');
avatar.http()
.get('/hello', function (req, res) {
res.send('Hello, Avatar!');
})
.listen(8080);
In this example, we’re creating an Avatar component that handles HTTP GET requests to the /hello
endpoint and responds with a simple message. This demonstrates how Avatar components can be used to handle web requests in a Java EE application.
Step 3: Integrating Java EE and Avatar Components
One of the key advantages of Avatar is its ability to seamlessly integrate Java EE components with Avatar components. This allows developers to leverage existing Java EE functionality while incorporating modern, lightweight Avatar components.
Here’s an example of integrating a Java EE CDI bean with an Avatar component:
@RequestScoped
public class GreetingService {
public String createGreeting(String name) {
return "Hello, " + name;
}
}
We can then call the GreetingService
from an Avatar component and use its functionality within the Avatar component, effectively combining Java EE and Avatar features.
Step 4: Deploying the Avatar-Enabled Application
Once the Avatar components are integrated into the Java EE application, the next step is to deploy the application. This can be done using traditional Java EE deployment methods, such as packaging the application into a WAR file and deploying it to a Java EE application server like Payara or WildFly.
Overcoming Java EE Limitations with Avatar
By implementing Avatar in Java EE, developers can effectively overcome the limitations of traditional Java EE applications. Avatar’s lightweight and modular architecture allows for greater flexibility, scalability, and integration with modern technologies, enabling developers to build cloud-native applications with ease.
While Java EE continues to be a robust platform for enterprise applications, the integration of Avatar opens up new possibilities for building modern, lightweight, and scalable applications that are well-suited for microservices and cloud-native architecture.
In conclusion, the implementation of Avatar in Java EE serves as a stepping stone towards modernizing and embracing the evolving landscape of cloud-native application development. By combining the strengths of Java EE with the flexibility of Avatar, developers can build robust, scalable, and future-ready applications that meet the demands of modern software architecture.
To explore more about Avatar and its features, you can refer to the official Project Avatar documentation.
The Closing Argument
In this blog post, we’ve discussed the limitations of traditional Java EE applications and introduced Project Avatar as a solution to overcome these limitations. We’ve explored the key steps for implementing Avatar in a Java EE application, including setting up the project, creating Avatar components, integrating Java EE and Avatar components, and deploying the Avatar-enabled application.
Through the integration of Avatar, developers can achieve a more flexible, scalable, and modern architecture for building cloud-native applications within the Java EE ecosystem. As technology continues to evolve, it’s essential for developers to embrace frameworks like Avatar to stay ahead in the rapidly changing landscape of enterprise application development.