Reactive Programming: Avoiding Callback Hell
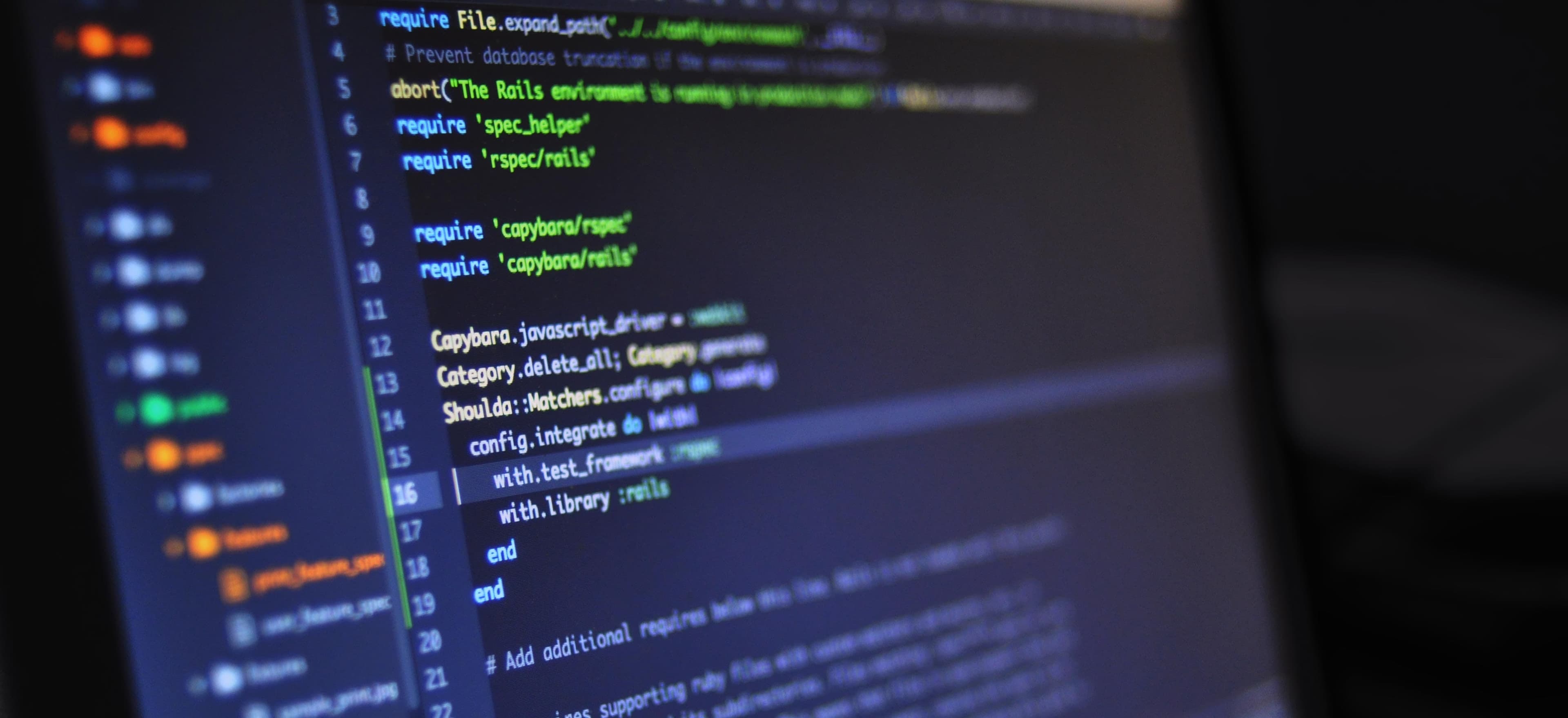
- Published on
Reactive Programming: Avoiding Callback Hell
In the world of asynchronous programming, callbacks are a fundamental concept. They allow us to define the behavior that should occur once an asynchronous operation completes. However, as our codebase grows, the use of callbacks can lead to a situation known as "callback hell". This is characterized by deeply nested callback functions, making code difficult to read, understand, and maintain. Reactive programming offers a solution to this problem by providing a more structured approach to handling asynchronous operations. In this article, we will explore how reactive programming, specifically using Java and the Reactor library, can help us avoid callback hell.
Understanding Callback Hell
Callback hell occurs when asynchronous operations are chained using nested callbacks, resulting in code that is hard to follow and reason about. Consider the following example of fetching data from an API and then performing a series of operations on the result:
getDataFromAPI(new Callback() {
@Override
public void onSuccess(Data data) {
processData(data, new Callback() {
@Override
public void onSuccess(ProcessedData processedData) {
updateUI(processedData, new Callback() {
@Override
public void onSuccess() {
showSuccessMessage();
}
@Override
public void onFailure() {
showErrorMessage();
}
});
}
@Override
public void onFailure() {
showErrorMessage();
}
});
}
@Override
public void onFailure() {
showErrorMessage();
}
});
As you can see, the code quickly becomes difficult to read and maintain due to the nested nature of the callbacks.
Introducing Reactive Programming
Reactive programming is a programming paradigm focused on asynchronous data streams. It provides a way to declaratively compose and transform streams of data, allowing us to easily handle asynchronous operations and events. In Java, the Reactor library provides a powerful set of tools for reactive programming, including the Flux
and Mono
types for handling asynchronous data flows.
Using Reactor to Avoid Callback Hell
Let's revisit the previous example and see how we can use Reactor to avoid callback hell:
getDataFromAPI()
.flatMap(this::processData)
.flatMap(this::updateUI)
.doOnSuccess(success -> showSuccessMessage())
.doOnError(error -> showErrorMessage())
.subscribe();
In this revised code, we use the flatMap
operator to chain asynchronous operations together in a more readable and maintainable way. Each operation returns a Mono
representing the asynchronous result, allowing us to easily compose the operations without deeply nested callbacks. Finally, we use the subscribe
method to initiate the asynchronous execution.
Advantages of Reactive Programming
Reactive programming not only helps us avoid callback hell, but it also offers several other advantages:
1. Declarative and Readable Code
By using operators such as flatMap
, map
, and filter
, reactive programming allows us to write code in a more declarative and readable manner. This makes it easier to understand the flow of data and operations being performed.
2. Error Handling
Reactor provides powerful error-handling mechanisms, allowing us to handle errors in a centralized and consistent manner. This simplifies error handling and makes our code more robust.
3. Asynchronous by Default
Reactor encourages the use of asynchronous operations by default, making it easier to work with asynchronous data streams without explicitly dealing with low-level concurrency constructs.
4. Backpressure Support
Reactor supports backpressure, allowing the consumer to control the rate at which data is consumed. This is essential for handling scenarios where data production is faster than consumption.
A Final Look
In conclusion, reactive programming, and specifically the Reactor library in Java, offers a powerful solution for avoiding callback hell and dealing with asynchronous operations in a more structured and manageable way. By leveraging the capabilities of Reactor, we can write code that is more readable, maintainable, and robust when dealing with asynchronous data streams. Asynchronous operations are a fundamental part of modern applications, and mastering reactive programming techniques can greatly improve the quality and maintainability of our code.
If you are interested in learning more about reactive programming and the Reactor library, I highly recommend checking out the official Reactor documentation and the Reactive Streams specification. These resources provide in-depth information and examples to help you dive deeper into the world of reactive programming.