Efficiently Convert JDBC ResultSet to CSV in Java
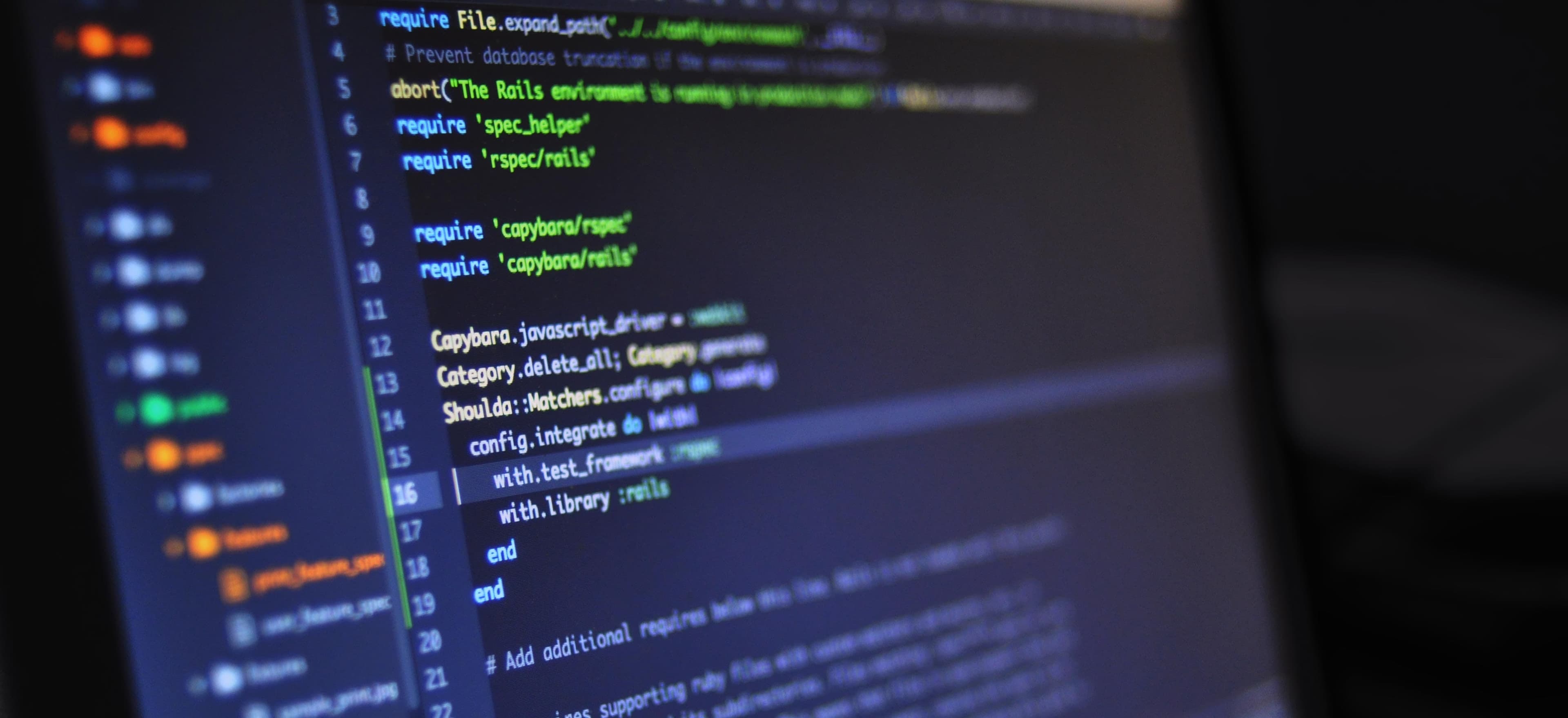
- Published on
A Comprehensive Guide to Efficiently Convert JDBC ResultSet to CSV in Java
When working with databases in Java, it's common to fetch data from the database using JDBC and then transform it into a more portable format like CSV. This guide will walk you through the process of efficiently converting a JDBC ResultSet to a CSV file in Java.
Why Convert JDBC ResultSet to CSV?
CSV (Comma-Separated Values) is a popular file format for exchanging tabular data. Converting a JDBC ResultSet to CSV allows for easy data transfer, sharing, and analysis.
Prerequisites
Before we delve into the code, make sure you have the following prerequisites in place:
- A basic understanding of Java and JDBC
- JDK installed on your machine
- A database (e.g., MySQL, PostgreSQL) with sample data
- JDBC driver for your database
Setting Up the Project
First, create a new Java project or use an existing one. Ensure your project includes the JDBC driver for the database you're working with.
Connecting to the Database
Here's a simple example of how to connect to a MySQL database using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
public static Connection connectToDatabase(String url, String user, String password) throws SQLException {
return DriverManager.getConnection(url, user, password);
}
public static void closeConnection(Connection connection) {
try {
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
// Handle the exception
}
}
}
In this example, url
is the JDBC URL of your database, and user
and password
are the credentials for accessing the database.
Executing a Query and Getting ResultSet
Assuming you have already executed a query and obtained a ResultSet object, we can now focus on converting this ResultSet to a CSV file.
import java.io.FileWriter;
import java.io.IOException;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
public class CSVConverter {
public static void resultSetToCSV(ResultSet resultSet, String filePath) throws SQLException, IOException {
ResultSetMetaData metaData = resultSet.getMetaData();
int columnCount = metaData.getColumnCount();
try (FileWriter writer = new FileWriter(filePath)) {
for (int i = 1; i <= columnCount; i++) {
writer.append(metaData.getColumnName(i));
if (i < columnCount) {
writer.append(',');
}
}
writer.append('\n');
while (resultSet.next()) {
for (int i = 1; i <= columnCount; i++) {
writer.append(resultSet.getString(i));
if (i < columnCount) {
writer.append(',');
}
}
writer.append('\n');
}
}
}
}
Let's break down the code:
- We start by obtaining the metadata of the ResultSet, which includes information about the columns and their types.
- We then iterate through the ResultSet and write each row to the CSV file, separating the column values with commas.
Using the CSVConverter
Now, let's see how we can use the CSVConverter
class to convert a ResultSet to a CSV file.
public class Main {
public static void main(String[] args) {
String jdbcUrl = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try (Connection connection = DatabaseConnector.connectToDatabase(jdbcUrl, user, password)) {
// Execute a query and obtain a ResultSet (assume resultSet is obtained here)
CSVConverter.resultSetToCSV(resultSet, "output.csv");
System.out.println("ResultSet converted to CSV successfully.");
} catch (SQLException | IOException e) {
e.printStackTrace();
}
}
}
In this example, we establish a connection to the database, execute a query to obtain a ResultSet, and then call the resultSetToCSV
method of CSVConverter
to convert the ResultSet to a CSV file.
Closing Remarks
In this guide, we explored how to efficiently convert a JDBC ResultSet to a CSV file in Java. By leveraging the JDBC API and basic file handling, we can seamlessly transform database query results into a widely-compatible format for further analysis and manipulation.
With these techniques in your toolkit, you can confidently handle data export from your Java applications and streamline the sharing and processing of tabular data.
Remember, handling exceptions and edge cases, such as null values and special characters, is crucial when working with real-world data, so always consider the specific requirements of your use case.
For further exploration:
- Download MySQL Connector/J
- PostgreSQL JDBC Driver