Preventing Dependency Hell in Software Development
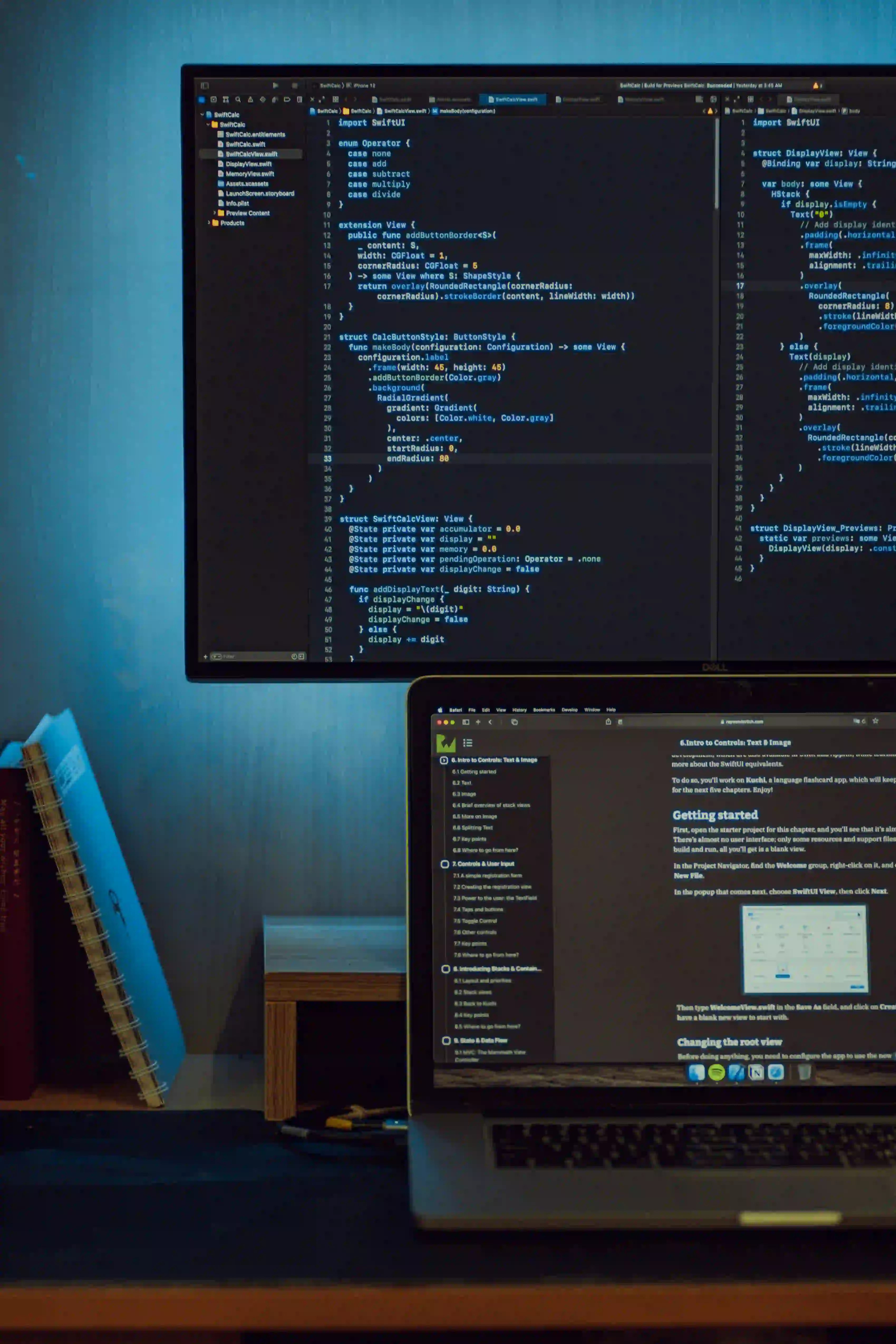
Preventing Dependency Hell in Java Software Development
Software development in Java often involves managing a multitude of dependencies. Dependencies are a necessary evil in modern software engineering, enabling developers to reuse code, add functionality, and streamline development. However, if left unchecked, dependencies can lead to a phenomenon known as “dependency hell.” This article delves into the causes of dependency hell and offers practical strategies to prevent it in Java development.
What is Dependency Hell?
Dependency hell occurs when a project’s dependencies become tangled, conflicting, or outdated, leading to a myriad of issues such as:
-
Version conflicts: Different modules or libraries within a project may depend on conflicting versions of the same dependency, leading to runtime errors and unpredictable behavior.
-
Incompatible dependencies: Dependencies may rely on incompatible third-party libraries, resulting in compilation failures or runtime crashes.
-
Transitive dependencies: As dependencies rely on other dependencies, the transitive closure can become overwhelming, making it difficult to determine the root cause of issues.
-
Security vulnerabilities: Outdated or unmaintained dependencies pose security risks, potentially exposing the software to exploits.
Strategies to Prevent Dependency Hell
1. Dependency Management Tools: Maven and Gradle
Maven and Gradle are popular build automation tools that offer robust dependency management capabilities. They allow developers to declare project dependencies, and these tools handle the retrieval, integration, and resolution of dependencies.
<!-- Maven Example -->
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>example-library</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Both Maven and Gradle use a declarative approach to specify dependencies, making it easier to manage and avoid conflicts. They also support the concept of dependency scopes, which helps in controlling the visibility and lifecycle of dependencies.
2. Dependency Locking
Dependency locking involves freezing the versions of dependencies used in a project. This ensures that the same versions are consistently used across different environments and builds, mitigating unexpected behavior due to version discrepancies.
Maven's Dependency Plugin and Gradle's Locking DSL enable developers to lock dependencies, thereby promoting reproducible and reliable builds.
3. Continuous Monitoring and Updates
Regularly monitoring dependencies for security vulnerabilities, updates, and deprecations is crucial for preventing dependency hell. Tools such as OWASP Dependency-Check and Snyk can be integrated into the build process to automatically identify known vulnerabilities in project dependencies.
4. Modularization and Microservices
Modularizing applications and adopting microservices architectures can help mitigate dependency hell by reducing the scope of interdependent components. This approach promotes encapsulation, autonomy, and clear boundaries between different parts of the system, thereby limiting the impact of dependency issues.
5. Semantic Versioning
Adhering to semantic versioning principles when managing dependencies can prevent compatibility issues. By following semantic versioning rules (MAJOR.MINOR.PATCH), developers can accurately convey the impact of changes in a dependency. This enables consumers of the dependency to make informed decisions about when to upgrade or adopt a new version.
6. Automated Testing with Mocking
Thorough testing, including unit tests and integration tests, can uncover dependency-related issues early in the development process. Using mocking frameworks such as Mockito or EasyMock allows developers to isolate dependencies and simulate their behavior, leading to more reliable and robust tests.
Final Thoughts
Dependency hell can be a daunting challenge in Java software development, but with proactive measures and best practices, it can be effectively mitigated. By embracing robust dependency management tools, version control practices, continuous monitoring, and architectural strategies, developers can navigate the complex landscape of dependencies with confidence and ensure the long-term stability and security of their software projects.
Navigating the complexities of dependencies in software development can be challenging, but with the right tools and practices in place, it's possible to prevent dependency hell and build reliable, secure, and maintainable Java applications.
For further reading on this topic, be sure to check out the official Maven documentation and Gradle's dependency management guide.