Effective Strategies for Microservices Test Automation
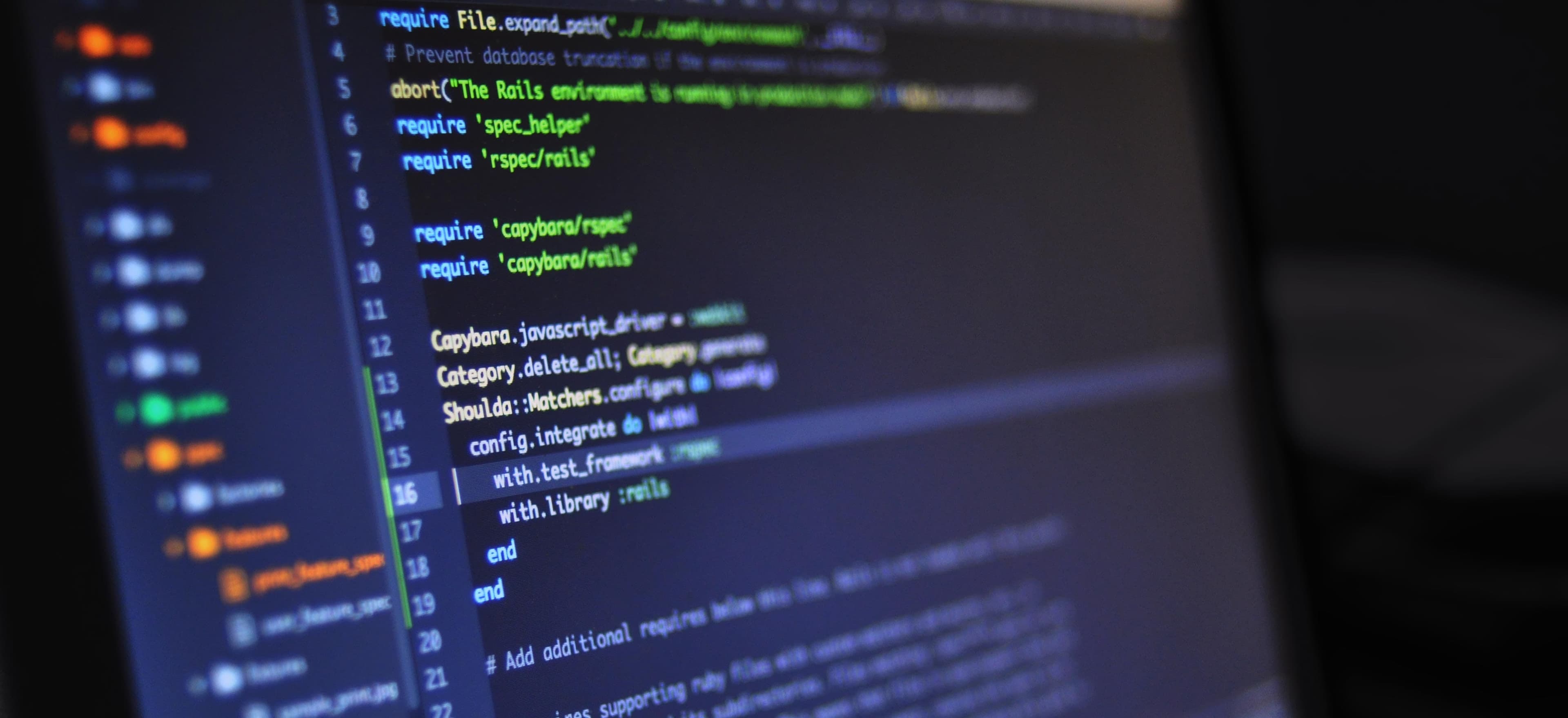
- Published on
Effective Strategies for Microservices Test Automation
In the realm of software development, microservices have become increasingly popular due to their scalability, flexibility, and reusability. With the rise of microservices architecture, the need for efficient test automation has become more critical than ever. In this article, we will explore effective strategies for test automation in the context of microservices using Java.
The Importance of Test Automation in Microservices
Microservices are typically small, independent services that work together to form an application. Each service may be developed, deployed, and scaled independently, making the overall application more resilient and easier to maintain. However, this also introduces complexities in testing, as each service needs to be thoroughly tested in isolation as well as in combination with other services.
The traditional manual testing methods are not sufficient for the intricacies of microservices architecture. Test automation allows for rapid, consistent, and reliable testing of each service and their interactions, ensuring the quality and stability of the entire system.
Choosing the Right Test Automation Framework
When it comes to test automation in Java, there are several popular frameworks to consider, such as JUnit, TestNG, and Mockito. It's crucial to choose a framework that aligns with the specific needs and challenges of testing microservices.
JUnit
JUnit is a widely used framework for unit testing in Java. It provides annotations for defining test methods and assertions for verifying the expected results. For microservices, JUnit is well-suited for writing and executing unit tests for individual service components.
@Test
public void validateUserInput() {
// Test logic here
assertNotNull(userInput);
// More assertions
}
TestNG
TestNG is another testing framework that offers more flexibility and additional features compared to JUnit. It supports parameterized and data-driven testing, making it suitable for testing various input combinations in microservices.
@Test(dataProvider = "inputData")
public void processUserData(UserData data) {
// Test logic using data
// Assertions
}
Mockito
Mockito is a mocking framework that is invaluable for isolating the component under test by simulating the behavior of its dependencies. In a microservices environment, where services often rely on other services or external resources, Mockito can be used to create mock objects for seamless and isolated testing.
@Mock
private DataService dataService;
@Test
public void fetchUserData() {
// Define mock behavior
when(dataService.getUserData("123")).thenReturn(new UserData("John Doe"));
// Test logic that uses dataService
// Assertions
}
Implementing End-to-End Testing
While unit testing is essential for validating individual service components, end-to-end testing is equally crucial for ensuring that all services work together as expected. In a microservices architecture, where services communicate over networks and APIs, end-to-end testing helps uncover issues related to inter-service communication, data consistency, and overall system behavior.
Using RestAssured for API Testing
RestAssured is a Java library that simplifies API testing by providing a fluent interface for making HTTP requests and validating responses. It can be seamlessly integrated into test automation frameworks like JUnit or TestNG to perform comprehensive API testing across microservices.
@Test
public void verifyDataConsistency() {
given()
.when()
.get("/users/{id}", 123)
.then()
.statusCode(200)
.body("name", equalTo("John Doe"));
// More assertions
}
Contract Testing with Pact
Pact is a contract testing tool that enables consumer-driven contract testing for microservices. It allows the consumer and provider services to define and verify the contracts for their interactions. Pact tests can be written in Java using the Pact JVM library to ensure that the communication between microservices adheres to the defined contracts.
@Pact(consumer = "Consumer")
public RequestResponsePact createPact(PactDslWithProvider builder) {
return builder
.uponReceiving("a request for user data")
.path("/users/123")
.method("GET")
.willRespondWith()
.status(200)
.body("{\"name\": \"John Doe\"}")
.toPact();
}
Managing Test Data for Microservices
In a microservices environment, managing test data becomes a significant challenge due to the distributed nature of services and databases. It's essential to have a strategy for provisioning and maintaining test data to support various types of testing, including unit, integration, and end-to-end testing.
Using Docker for Test Data Provisioning
Docker provides a convenient way to create and manage containers for databases, third-party services, and other dependencies required for testing microservices. By utilizing Docker Compose, test environments with preconfigured test data can be spun up on-demand, enabling consistent and reproducible testing across different environments.
version: '3'
services:
db:
image: postgres
environment:
POSTGRES_USER: testuser
POSTGRES_PASSWORD: testpassword
POSTGRES_DB: testdb
ports:
- "5432:5432"
Rolling Back Data Changes with Flyway
Flyway is a database migration tool that facilitates version control and management of database schemas and test data. In a microservices architecture, where each service may have its database, Flyway can be used to manage schema changes and ensure that test data is rolled back to a clean state after each test run, preventing data pollution and interference between tests.
@Test
public void verifyDataConsistency() {
// Test logic that modifies data
// Assertions
// Roll back data changes using Flyway
flyway.clean();
flyway.migrate();
}
Continuous Integration and Deployment (CI/CD) for Microservices Testing
CI/CD pipelines play a vital role in automating the build, test, and deployment processes for microservices. Tools like Jenkins, GitLab CI, and Travis CI provide robust capabilities for orchestrating test automation and ensuring the quality of microservices throughout the development lifecycle.
Jenkins Pipeline for Microservices Testing
Jenkins Pipeline allows the creation of complex, multi-stage pipelines for building, testing, and deploying microservices. By defining Jenkinsfile with stages for different types of tests (unit, integration, end-to-end), along with integrated tooling like Docker and Maven, comprehensive test automation can be seamlessly integrated into the CI/CD process.
pipeline {
agent any
stages {
stage('Build') {
steps {
// Maven build
}
}
stage('Unit Tests') {
steps {
// Execute unit tests
}
}
// More stages for integration tests, end-to-end tests, etc.
}
post {
always {
// Clean up resources
}
}
}
GitLab CI for Dockerized Test Environments
GitLab CI/CD integrates seamlessly with Docker, allowing the creation of disposable test environments for microservices testing. By defining .gitlab-ci.yml with service containers and test stages, GitLab CI enables parallelized and isolated test execution across multiple microservices while maintaining environmental consistency.
services:
- name: postgres:latest
alias: db
stages:
- test
unit_test:
stage: test
script:
- mvn test
Final Thoughts
Test automation is fundamental for ensuring the reliability and stability of microservices in today's software development landscape. By leveraging the right test automation frameworks, implementing end-to-end testing strategies, managing test data effectively, and integrating test automation into CI/CD pipelines, organizations can achieve comprehensive and efficient testing of their microservices. Java, with its robust ecosystem of testing tools and libraries, provides a solid foundation for building a robust test automation strategy tailored to the complexities of microservices architecture.
It's evident that an effective test automation strategy is a cornerstone for successful microservices implementation. Investing in test automation not only ensures the quality of microservices but also accelerates the development and deployment cycles, ultimately leading to better customer satisfaction and business outcomes.
In conclusion, test automation in the context of microservices is not just a technical necessity but a strategic enabler for organizations striving to thrive in today's competitive and dynamic market.
By embracing these effective strategies for microservices test automation, organizations can bolster their confidence in the reliability, performance, and scalability of their microservices, paving the way for innovation and success in the digital era.
Incorporating these strategies into your microservices testing approach will not only ensure the robustness of your applications but also streamline your development processes, enabling you to deliver high-quality software at the speed and scale demanded by modern businesses.
We have discussed a comprehensive approach to microservices test automation with a focus on utilizing Java and its ecosystem for robust and efficient testing strategies. Now, it's time to put these strategies into practice and elevate the quality and reliability of your microservices.