Common Java 12 Issues in Eclipse: Quick Fixes You Need
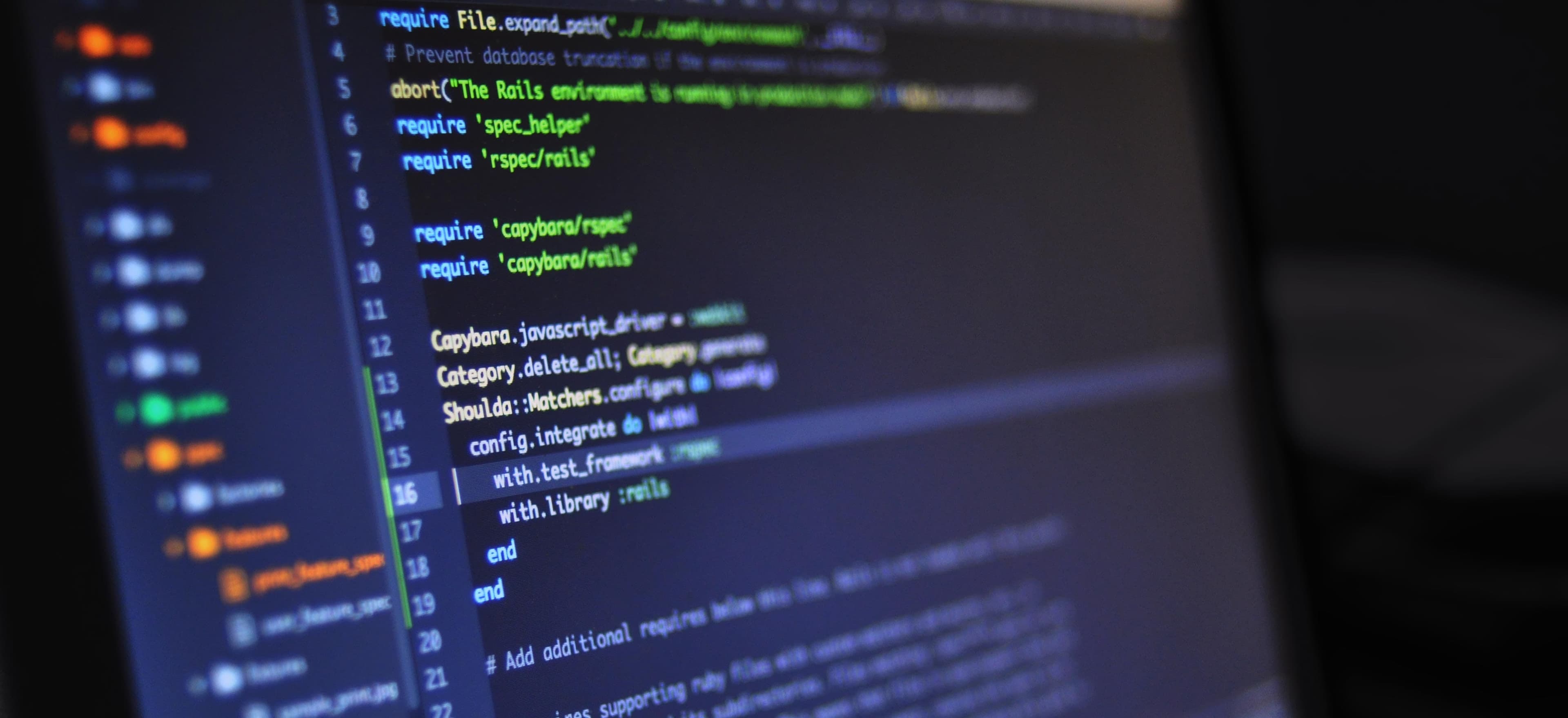
- Published on
Common Java 12 Issues in Eclipse: Quick Fixes You Need
Java 12 brought a host of exciting features and enhancements to the Java programming language, including new utilities and performance improvements. However, with every new release, developers sometimes face specific issues, particularly when using popular Integrated Development Environments (IDEs) like Eclipse.
In this blog post, we will explore common Java 12 issues encountered in Eclipse and provide quick fixes to help you streamline your Java development process. We'll ensure that our discussions are engaging and informative, with clear code examples that demonstrate the solutions effectively.
1. Compatibility Issues
As Java evolves, some environments and libraries may not initially support newer versions, leading to compatibility issues. When using Java 12, some Eclipse users may encounter errors indicating that the Java Development Kit (JDK) is not correctly set up.
Solution
-
Install JDK 12: Ensure you have JDK 12 installed on your machine. You can download it from the official Oracle JDK page or use OpenJDK.
-
Configure Eclipse:
- Open Eclipse.
- Go to
Window > Preferences
. - Expand
Java
, then click onInstalled JREs
. - Add the JDK 12 installation location as a new JRE, check the box to set it as the default JRE.
// Sample code to check Java version
public class VersionCheck {
public static void main(String[] args) {
System.out.println("Java Version: " + System.getProperty("java.version"));
}
}
This code will print the Java version currently being used. If it's anything other than 12.x, your setup might be incorrect.
2. Error in the Build Path
After upgrading to Java 12, you may find that your project cannot build due to a "build path error." This can often occur when the Java Build Path does not point to the correct JDK version.
Solution
- Check Build Path:
- Right-click your project in Eclipse.
- Select
Properties
. - Under
Java Build Path
, make sure the Libraries tab lists JDK 12. If it does not, remove the existing JRE system library and add the JDK 12 library.
// Ensure correct imports
import java.util.List;
public class BuildPathCheck {
public static void main(String[] args) {
List<String> list = List.of("Java", "Eclipse", "12");
System.out.println(list);
}
}
In this example, we utilize the new List.of()
method introduced in Java 9, verifying that our build path is set correctly to use these modern features.
3. Lack of New Features
Developers upgrading to Java 12 might expect to use newly introduced features but might face issues accessing them due to Eclipse settings. Common features such as the switch
expression or text blocks
might not work seamlessly.
Solution
-
Eclipse Update: Ensure that your Eclipse IDE is updated. Recent editions (Eclipse 2019-03 and later) provide better support for Java 12 features.
-
Install Compiler Compliance Level:
- Replace the project’s compiler compliance settings:
- Right-click the project.
- Go to
Properties > Java Compiler
. - Set the
Compiler compliance level
to12
.
- Replace the project’s compiler compliance settings:
Here’s a simple example of a switch expression:
public class SwitchExpressionDemo {
public static void main(String[] args) {
String dayType = "Monday";
String type = switch (dayType) {
case "Monday", "Tuesday", "Wednesday", "Thursday", "Friday" -> "Weekday";
case "Saturday", "Sunday" -> "Weekend";
default -> throw new IllegalArgumentException("Invalid day: " + dayType);
};
System.out.println("It's a: " + type);
}
}
In the above code, we have employed the enhanced switch
expression, which makes the code cleaner and more readable.
4. Problems with Maven or Gradle
If your project uses a build system like Maven or Gradle, you might encounter issues if the configuration doesn't specify Java 12 correctly. Your dependencies might fail to resolve due to version mismatches.
Solution
- Updating Maven:
- Open the
pom.xml
file and ensure that the Java version is set to 12:
- Open the
<properties>
<maven.compiler.source>12</maven.compiler.source>
<maven.compiler.target>12</maven.compiler.target>
</properties>
- Updating Gradle:
- In your
build.gradle
, ensure the source and target compatibility is set to 12:
- In your
sourceCompatibility = '12'
targetCompatibility = '12'
Using the right version in the build tool helps avoid compatibility or runtime errors.
5. IDE Performance Issues
Eclipse users may experience sluggish performance due to memory allocation or garbage collection issues after upgrading to Java 12.
Solution
- Increase Memory Allocation:
- Modify the Eclipse executable (
eclipse.ini
) to set higher memory limits.
- Modify the Eclipse executable (
For example:
-Xms512m
-Xmx2048m
This tells Eclipse to use between 512 MB and 2048 MB of memory, reducing the likelihood of performance degradation.
The Closing Argument
Java 12 has a lot to offer, and using it within Eclipse should enhance your development experience. You may encounter some bumps along the way, but with the solutions provided in this post, many common issues can be resolved quickly.
Stay up to date by regularly checking for updates in Eclipse, and don’t forget to review the recently introduced features in Java 12. For more in-depth information about the new features, refer to the official Java Documentation.
With these tips, you should be well-equipped to handle most of the common problems faced while using Java 12 in Eclipse. Happy coding!
By following the insights highlighted above, developers can enhance their productivity and tackle integration challenges efficiently. Should you require further assistance, feel free to explore Java forums or community discussions tailored to the current developments.