Effective Memory Management for Preventing Crashes
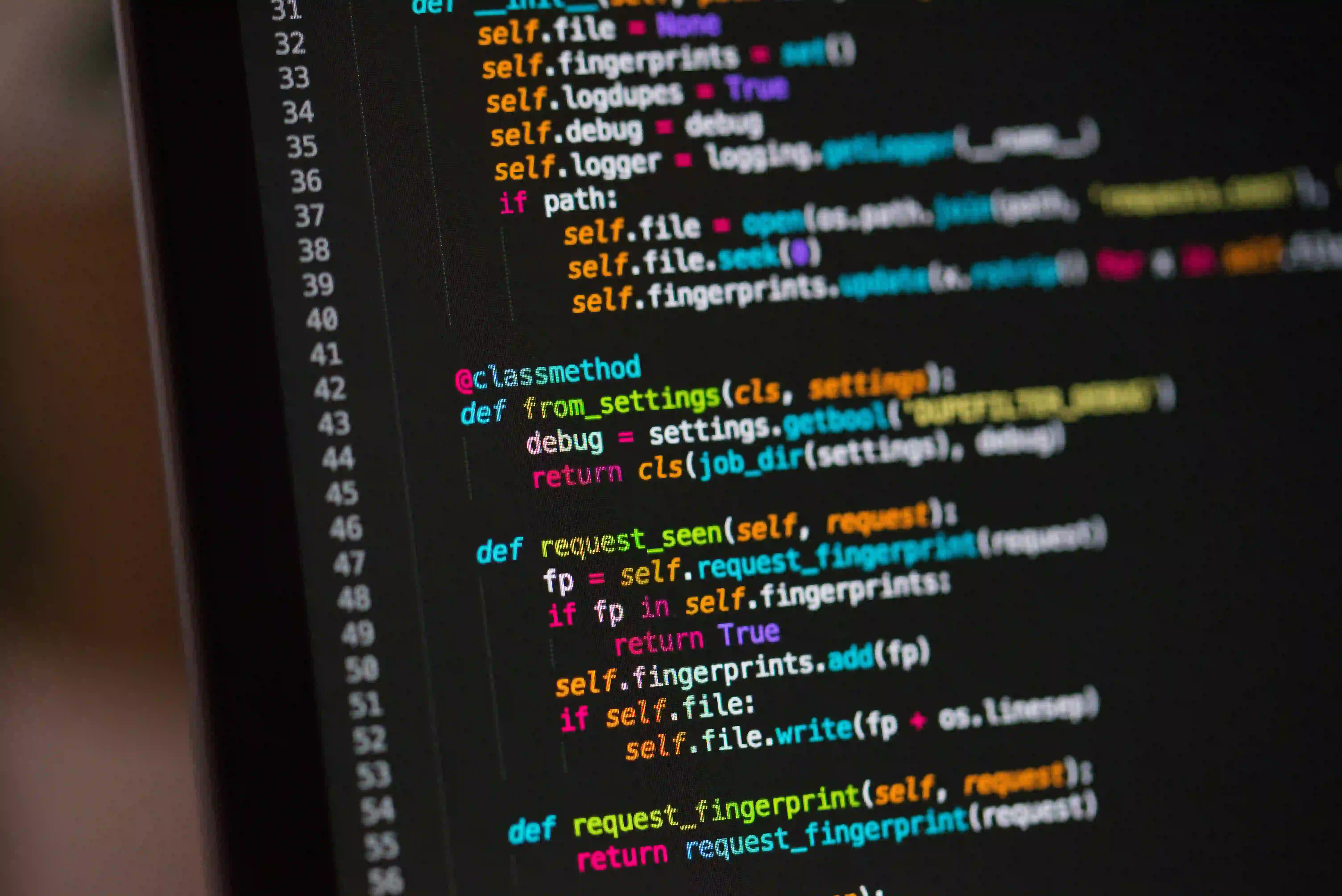
The Importance of Memory Management in Java
In the world of programming, memory management plays a pivotal role in ensuring the smooth running and stability of applications. Java, being a high-level language, abstracts most memory management tasks from the developer. However, this doesn't mean that memory issues are completely eradicated. In fact, improper memory management in Java can result in memory leaks, which can lead to application crashes and performance degradation.
In this blog post, we'll delve into the importance of effective memory management in Java and explore some best practices to prevent crashes and optimize memory usage.
Understanding Memory Management in Java
Java employs an automatic memory management system through its built-in garbage collector. This means that developers are relieved from manually allocating and deallocating memory for objects, unlike in languages such as C or C++. The garbage collector automatically reclaims memory from objects that are no longer in use, thus preventing memory leaks and fragmentation.
While this automated memory management system is undoubtedly convenient, it doesn't absolve developers from the responsibility of writing efficient code and being mindful of memory usage. Inefficient code can lead to excessive memory consumption, longer garbage collection pauses, and ultimately, crashes.
Memory Management Best Practices in Java
1. Use Primitive Types Where Possible
// Bad practice
Integer count = new Integer(5);
// Good practice
int count = 5;
By using primitive types such as int
, float
, double
, etc., instead of their corresponding wrapper classes (Integer
, Float
, Double
), unnecessary overhead can be avoided. Wrapper classes consume more memory and can lead to inefficient memory usage, especially in scenarios where a large number of instances are created.
2. Be Cautious with String Manipulation
// Bad practice
String result = "";
for (int i = 0; i < 10000; i++) {
result += "a";
}
// Good practice
StringBuilder result = new StringBuilder();
for (int i = 0; i < 10000; i++) {
result.append("a");
}
Strings in Java are immutable, meaning every time a String is concatenated or modified, a new String object is created. This can lead to excessive memory consumption and degraded performance. Using StringBuilder
for string manipulation minimizes the creation of unnecessary String objects, resulting in more efficient memory usage.
3. Release Resources in a Timely Manner
// Bad practice
InputStream is = null;
try {
is = new FileInputStream("example.txt");
// Processing the input stream
} catch (IOException e) {
e.printStackTrace();
} finally {
// Resource not closed
}
// Good practice
try (InputStream is = new FileInputStream("example.txt")) {
// Processing the input stream
} catch (IOException e) {
e.printStackTrace();
}
Failing to release resources such as file handles, database connections, or network connections can lead to resource leaks, which in turn can cause memory exhaustion and application crashes. Using try-with-resources (introduced in Java 7) ensures that the associated resources are closed when they are no longer needed, preventing memory leaks and improving overall resource management.
Avoiding Memory Leaks
Memory leaks occur when objects that are no longer needed are not properly released, causing the memory they occupy to be unavailable for other tasks. In Java, some common causes of memory leaks include retaining references to objects longer than necessary, improper use of static collections, and not deregistering listeners or callbacks.
To prevent memory leaks, it's crucial to:
- Be cautious with the use of static variables and collections, as they can cause objects to live longer than necessary.
- Ensure that listeners and callbacks are properly deregistered when they are no longer needed.
- Use memory profiling tools such as YourKit, VisualVM, or Java Mission Control to identify memory leaks and analyze memory usage patterns in your application.
Closing the Chapter
Effective memory management is critical for preventing crashes and optimizing the performance of Java applications. By following best practices such as using primitive types, being mindful of string manipulation, releasing resources in a timely manner, and avoiding memory leaks, developers can ensure that their applications run smoothly and efficiently.
Remember, while Java's automatic memory management alleviates the burden of manual memory allocation and deallocation, it's still essential to write efficient code and be conscious of memory usage to prevent crashes and maintain optimal performance.