Choosing the Right Method: save vs persist in Hibernate
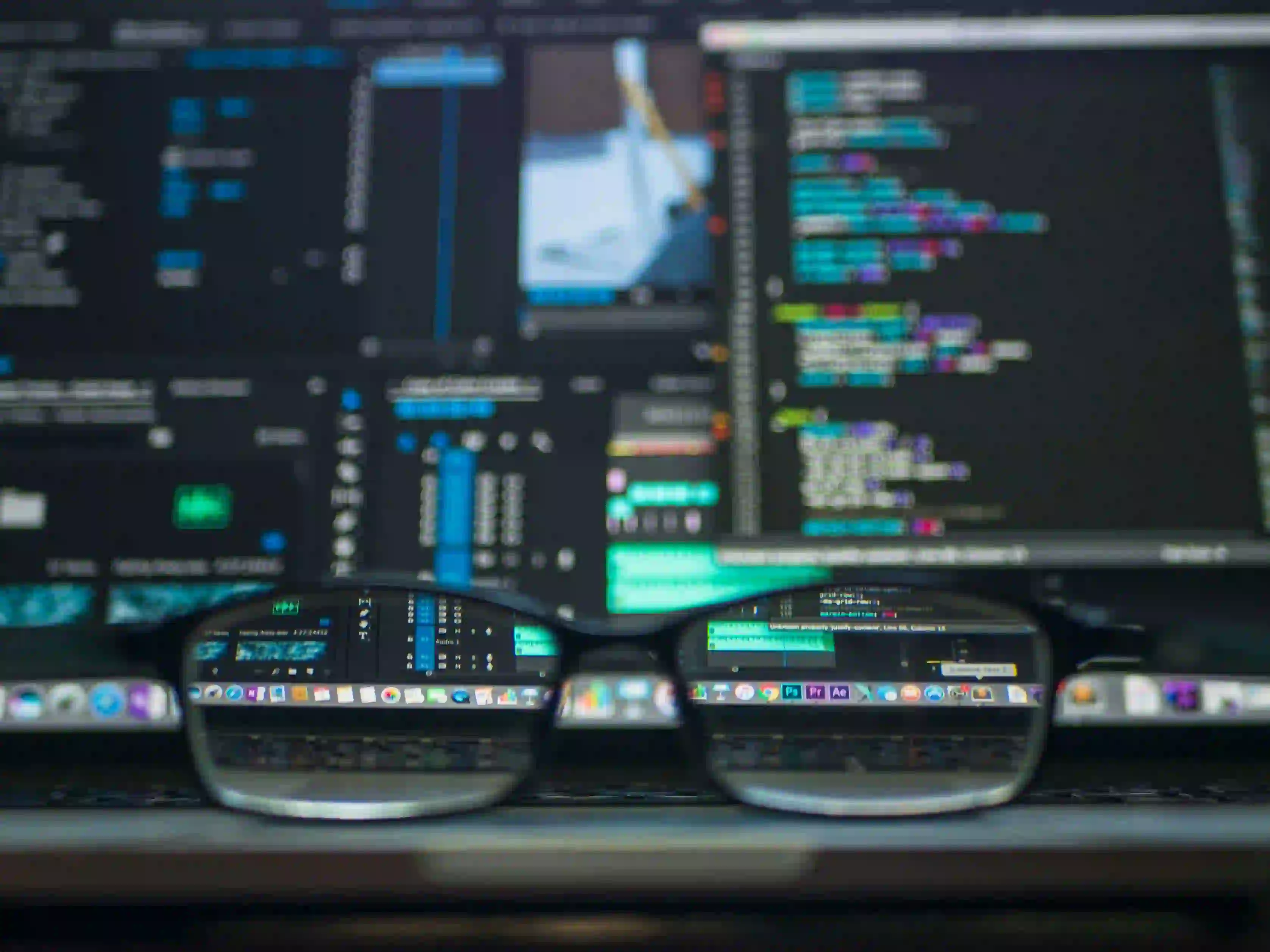
Choosing the Right Method: save vs persist in Hibernate
When working with Hibernate, a popular object-relational mapping (ORM) tool for Java, there are multiple ways to save an object to the database. Two commonly used methods are save
and persist
. While both achieve the same goal of persisting an object, there are subtle differences between the two approaches that are worth exploring to ensure you are using the right method for your specific use case.
Understanding save
and persist
save()
The save
method in Hibernate is used to persist an entity object into the database. It returns the generated identifier of the entity, allowing you to retrieve the key of the saved entity. The save
method also assigns an identifier to the object if it doesn't have one, hence making it persistent.
persist()
On the other hand, the persist
method is also used to make an entity persistent. However, unlike save
, it does not return the generated identifier immediately. The persist
method is void and its main purpose is to make a transient instance persistent. It's worth noting that it is not executed immediately, as it may be postponed till the transaction is committed.
Key Differences
Return Type
The most significant difference lies in the return type of these methods. While save
returns the generated identifier of the entity, persist
does not return anything.
Impact of Return Type
Understanding the return type of these methods can influence your choice depending on whether you need the generated identifier immediately (save
) or if the process can be deferred (persist
).
Transactional Considerations
The persist
method binds the entity instance to the persistence context, making it managed, whereas the save
method does not immediately associate the object with the persistence context. This has implications on the order of database operations within a transaction.
Code Examples
// Using save()
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
Employee employee = new Employee("John Doe", "Engineering");
Long employeeId = (Long) session.save(employee);
tx.commit();
session.close();
In the example above, the save
method is used to persist the Employee
object, and the generated identifier is retrieved immediately for further use within the transaction.
// Using persist()
Session session = sessionFactory.openSession();
Transaction tx = session.beginTransaction();
Employee employee = new Employee("Jane Smith", "Marketing");
session.persist(employee);
tx.commit();
session.close();
In this example, the persist
method is used to make the Employee
object persistent within the transaction. The generated identifier is not returned immediately.
When to Use Each Method
Using save()
Use the save
method when you need the generated identifier immediately for further processing within the same transaction. This is particularly useful when you want to perform additional operations that depend on the identifier of the saved entity within the same session and transaction.
Using persist()
The persist
method is suitable when you want to make an object persistent without immediately needing the generated identifier. It allows Hibernate to decide the best time to execute the SQL INSERT operation, which can be beneficial in terms of database optimizations.
The Last Word
In conclusion, both the save
and persist
methods in Hibernate serve the purpose of persisting entity objects, but their subtle differences make them suited for different scenarios. Understanding these differences is crucial for choosing the right method based on the specific requirements of your application.
By considering the impact of the return type, transactional implications, and the immediate need for the generated identifier, you can make an informed decision on whether to use save
or persist
in your Hibernate-based application. Remember, the right choice can lead to more optimized and efficient database operations.
To delve deeper into Hibernate methods, transactions, and best practices, continue exploring the Hibernate documentation and the vast array of resources available in the Java ecosystem.