Preventing Data Loss: Best Practices for Using MongoDB GridFS Remove Method
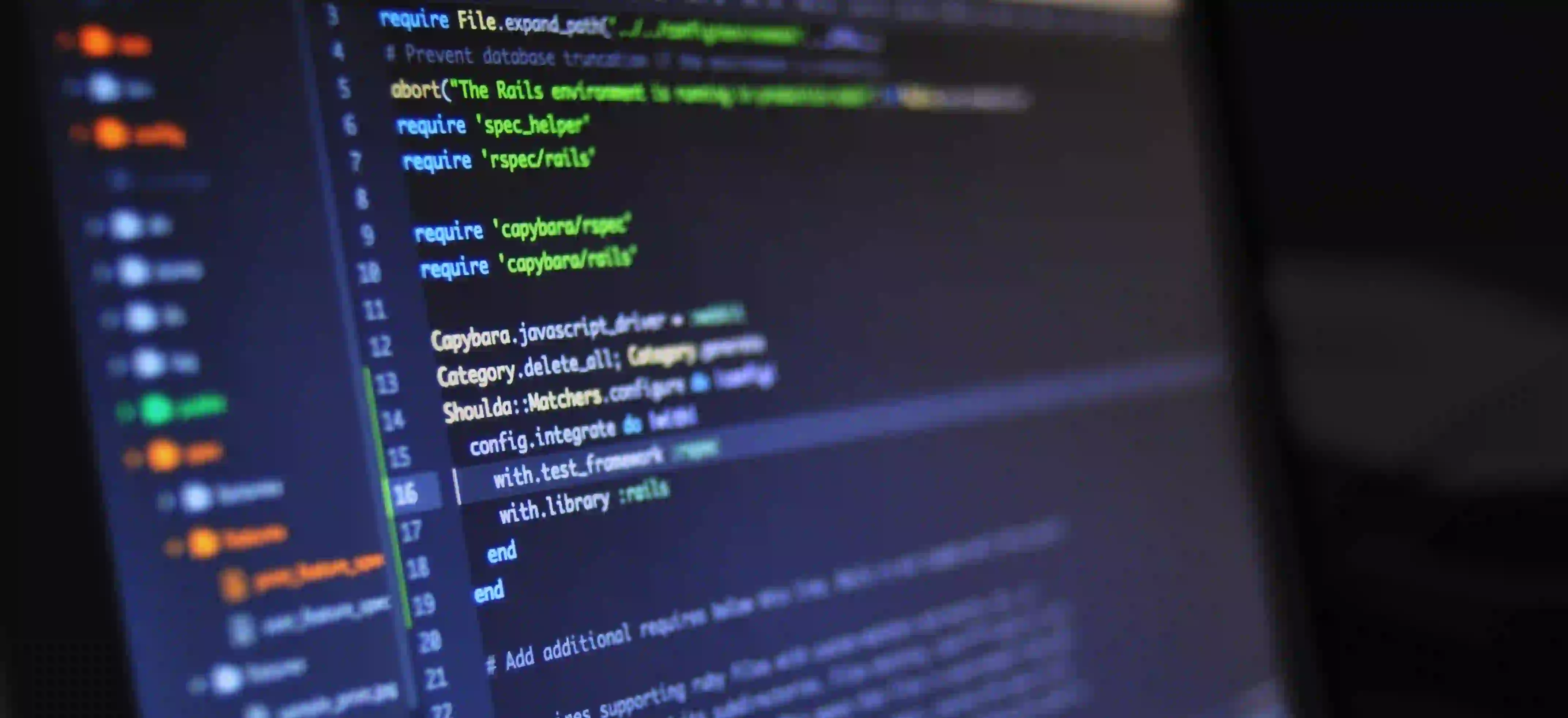
Preventing Data Loss: Best Practices for Using MongoDB GridFS Remove Method
Data loss is a significant concern in any software application, and as a Java developer, it's crucial to ensure that the code you write is robust and resilient to potential data loss scenarios. In this article, we'll explore best practices for using the MongoDB GridFS remove method to prevent inadvertent data loss.
Understanding MongoDB GridFS
MongoDB GridFS is a specification for storing and retrieving large files, such as images, videos, and audio files, in MongoDB. It divides a file into smaller chunks known as "chunks," which are then stored as individual documents in a collections. Each file is represented by a pair of collections: the files collection, which stores file metadata, and the chunks collection, which stores the binary data.
The remove
method in GridFS is used to delete files stored in the database. While this method is essential for managing data, it also carries the risk of unintentional data loss if not used carefully.
Best Practices for Using the GridFS Remove Method
1. Always Verify File Existence Before Deletion
Before invoking the remove
method, it's essential to verify the existence of the file to be deleted. Without this check, there's a risk of deleting non-existent files, potentially leading to data loss.
String fileId = "file_id_to_delete";
GridFSFile fileToDelete = gridFsTemplate.findOne(new Query(Criteria.where("_id").is(fileId)));
if (fileToDelete != null) {
gridFsTemplate.delete(new Query(Criteria.where("_id").is(fileId)));
} else {
// Handle the case where the file does not exist
}
By checking the existence of the file before deletion, you can prevent accidental removal of non-existent files and ensure data integrity.
2. Limit Access to the Deletion Operation
Restrict access to the remove
method to only authorized users or roles. This can be achieved through authentication and authorization mechanisms provided by MongoDB or your application. By controlling access, you mitigate the risk of unauthorized data deletion.
3. Implement Soft Deletion if Required
Instead of physically removing files from the database, consider implementing a soft deletion mechanism where files are marked as "deleted" but not removed from the database. This approach provides an opportunity to recover accidentally deleted files and offers an extra layer of protection against data loss.
String fileId = "file_id_to_delete";
Update update = new Update().set("deleted", true);
gridFsTemplate.updateFirst(new Query(Criteria.where("_id").is(fileId)), update, GridFsFile.class);
Soft deletion can be particularly useful in scenarios where regulatory compliance or audit requirements mandate retaining deleted files for a certain period.
4. Regularly Back Up the Database
Regular database backups are critical for safeguarding against irreversible data loss. By implementing a robust backup strategy, you can mitigate the impact of accidental file deletions and restore lost data if needed.
Consider using MongoDB's built-in backup and restore mechanisms or third-party backup solutions that integrate seamlessly with MongoDB.
5. Logging and Monitoring
Implement logging and monitoring for all operations that involve file deletions. By maintaining comprehensive logs and monitoring deletion activities, you can quickly identify and respond to any unauthorized or erroneous deletion attempts.
Leverage MongoDB's logging features and integrate them with your application's logging infrastructure for centralized monitoring and analysis of deletion-related activities.
Final Thoughts
Preventing data loss when using the MongoDB GridFS remove method requires a combination of careful validation, access control, backup strategies, and monitoring. By following these best practices and integrating them into your Java application, you can reduce the risk of inadvertent data loss and ensure the integrity and resilience of your file storage operations.
In conclusion, approaching the remove
method with caution and implementing a comprehensive data loss prevention strategy is crucial for maintaining the reliability and security of your MongoDB GridFS-based file storage system.
For more in-depth information on MongoDB GridFS and the remove method, refer to the official MongoDB documentation.
Remember, the key to effective data loss prevention is a proactive and multi-faceted approach that addresses potential vulnerabilities from various angles. By incorporating these best practices into your Java application, you can strengthen its resilience and minimize the risk of data loss when using MongoDB GridFS.