Building Scalable Web Services from Scratch
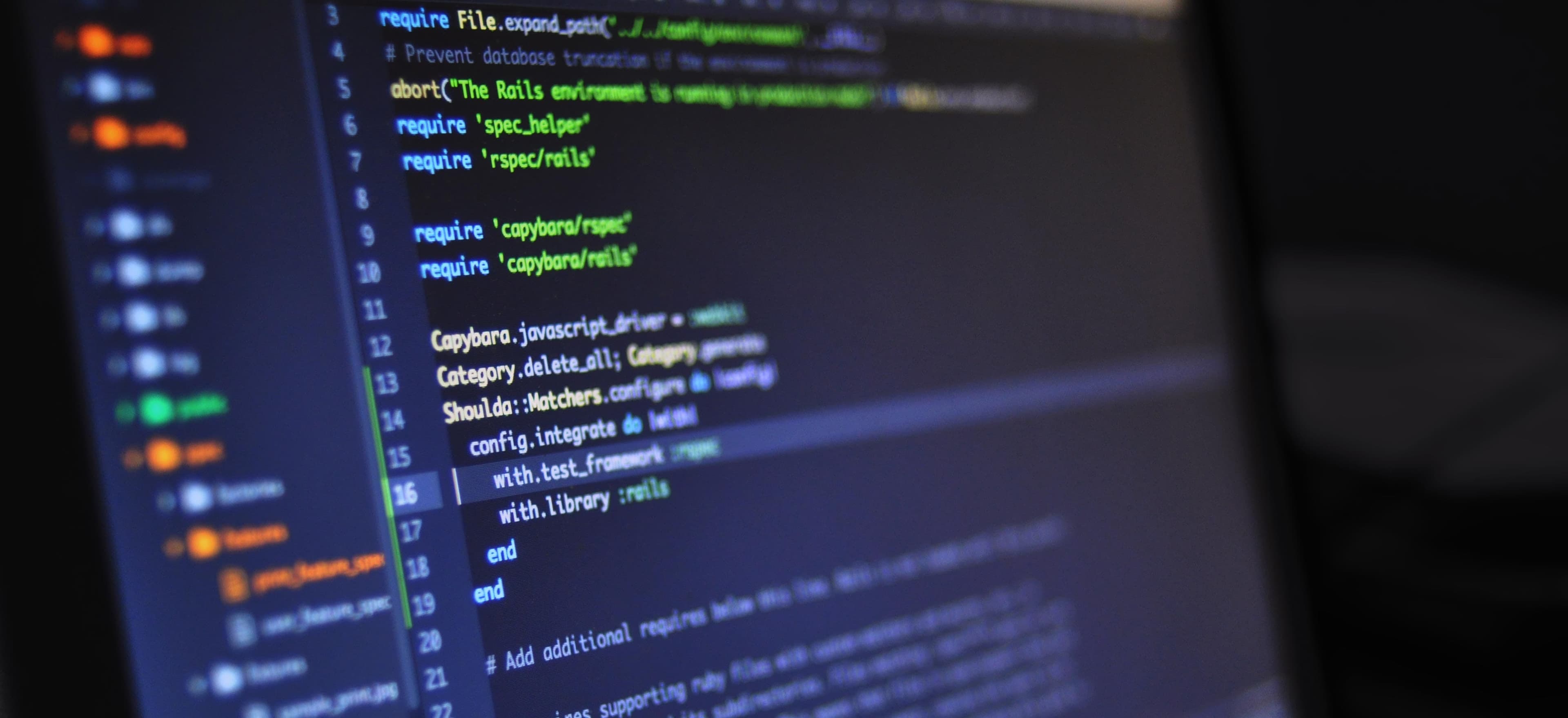
- Published on
Building Scalable Web Services from Scratch
In today's fast-paced digital world, the demand for scalable web services has skyrocketed. Whether you are a seasoned developer or just starting with web development, understanding how to build scalable web services from scratch is crucial. In this post, we will delve into the core principles and best practices for developing scalable web services using Java.
Understanding Scalability
Before we dive into the technical aspects, let's first understand what scalability means in the context of web services. Scalability refers to the ability of a system to handle an increasing amount of work by adding resources to the system. In the context of web services, this means being able to handle a growing number of users, requests, and data without compromising performance.
Choosing the Right Framework
When it comes to developing scalable web services in Java, choosing the right framework is essential. Spring Boot, with its robust features and extensive community support, stands out as one of the top choices for building scalable web services.
Example: Setting up a Simple Spring Boot Application
@SpringBootApplication
public class ScalableWebServiceApplication {
public static void main(String[] args) {
SpringApplication.run(ScalableWebServiceApplication.class, args);
}
}
In the above example, we use the @SpringBootApplication
annotation to indicate that this is the main application class. When the main
method is executed, it launches the Spring Boot application.
Database Design for Scalability
As the amount of data and user interactions grows, the database becomes a critical component of scalable web services. Proper database design is crucial for ensuring the scalability of web services. Using techniques such as database sharding, indexing, and denormalization can significantly impact the performance and scalability of the system.
Example: Implementing Database Sharding
public class UserShardStrategy implements ShardStrategy {
@Override
public int getShardKey(Object obj) {
// Implement sharding logic based on user attributes
// Return the shard key
}
}
In the above example, we define a UserShardStrategy
class that implements the ShardStrategy
interface. This allows us to define the sharding logic based on user attributes, enabling horizontal scaling of the database.
Utilizing Caching for Performance
Caching is a powerful technique for improving the performance and scalability of web services. By storing frequently accessed data in a cache, we can reduce the load on the underlying data store and improve response times. In Java, popular caching solutions like Redis and Ehcache provide robust support for implementing caching in web services.
Example: Integrating Redis for Caching
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public RedisCacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) {
return RedisCacheManager.builder(redisConnectionFactory).build();
}
}
In the above example, we configure Redis as the caching provider using the RedisCacheManager
in a Spring Boot application. This enables us to leverage Redis for efficient caching of data, thereby enhancing the scalability of the web service.
Leveraging Asynchronous Processing
Traditional web services often struggle with handling concurrent requests efficiently. Leveraging asynchronous processing can significantly improve the scalability of web services by allowing the system to handle a larger number of requests without increasing resource consumption.
Example: Implementing Asynchronous Processing with CompletableFuture
public CompletableFuture<User> getUserAsync(String userId) {
return CompletableFuture.supplyAsync(() -> userRepository.getUserById(userId));
}
In the above example, we define a method to fetch user details asynchronously using CompletableFuture
in Java. This allows the system to process other requests while waiting for the user data, thereby improving overall responsiveness and scalability.
Ensuring Fault Tolerance
Building scalable web services also involves ensuring fault tolerance to handle potential failures gracefully. Techniques such as implementing retry mechanisms, circuit breakers, and graceful degradation can help maintain the availability and performance of the web service under adverse conditions.
Example: Implementing Circuit Breaker Pattern with Resilience4j
CircuitBreakerConfig circuitBreakerConfig = CircuitBreakerConfig.custom()
.failureRateThreshold(50)
.waitDurationInOpenState(Duration.ofMillis(1000))
.build();
CircuitBreaker circuitBreaker = CircuitBreakerRegistry.of(circuitBreakerConfig).circuitBreaker("userService");
CheckedFunction0<User> userSupplier = CircuitBreaker.decorateCheckedSupplier(circuitBreaker,
() -> userService.getUserDetails(userId));
Try<User> result = Try.of(userSupplier)
.recover(throwable -> {
// Handle the fallback or recovery logic
});
In the above example, we configure a circuit breaker using Resilience4j to protect the userService
from potential failures. By defining a fallback logic, we ensure that the web service remains operational even when the underlying service encounters issues.
Continuous Monitoring and Scaling
Lastly, continuous monitoring and auto-scaling are essential for maintaining the scalability of web services. By leveraging monitoring tools and cloud platform capabilities, we can analyze the system's performance and automatically scale resources based on the demand.
Example: Using Prometheus for Monitoring
Prometheus is a popular open-source monitoring solution that provides robust support for monitoring web services. By instrumenting the web service with Prometheus metrics, we can gain valuable insights into the system's performance and resource utilization, enabling proactive scaling based on the observed patterns.
As we conclude our exploration of building scalable web services from scratch using Java, it's essential to keep in mind that scalability is not a one-time achievement but an ongoing process that requires continuous refinement and optimization.
By employing the right frameworks, database design, caching strategies, asynchronous processing, fault tolerance mechanisms, and continuous monitoring, developers can ensure that their web services remain resilient and responsive in the face of growing demands, ultimately delivering a seamless user experience.
In a nutshell, the journey to building scalable web services from scratch in Java is a combination of sound technical choices, architectural considerations, and a proactive mindset towards performance optimization and scalability.
Start building your scalable web services from scratch today and embrace the rewarding challenge of delivering high-performing, resilient, and scalable solutions in the ever-evolving digital landscape.
Remember, the key to scalability lies in thoughtful design, strategic implementation, and relentless commitment to refining and evolving your web services as the demands scale. Here's to building scalable web services that stand the test of time!