Optimizing Garbage Collection for Low CPU Overhead
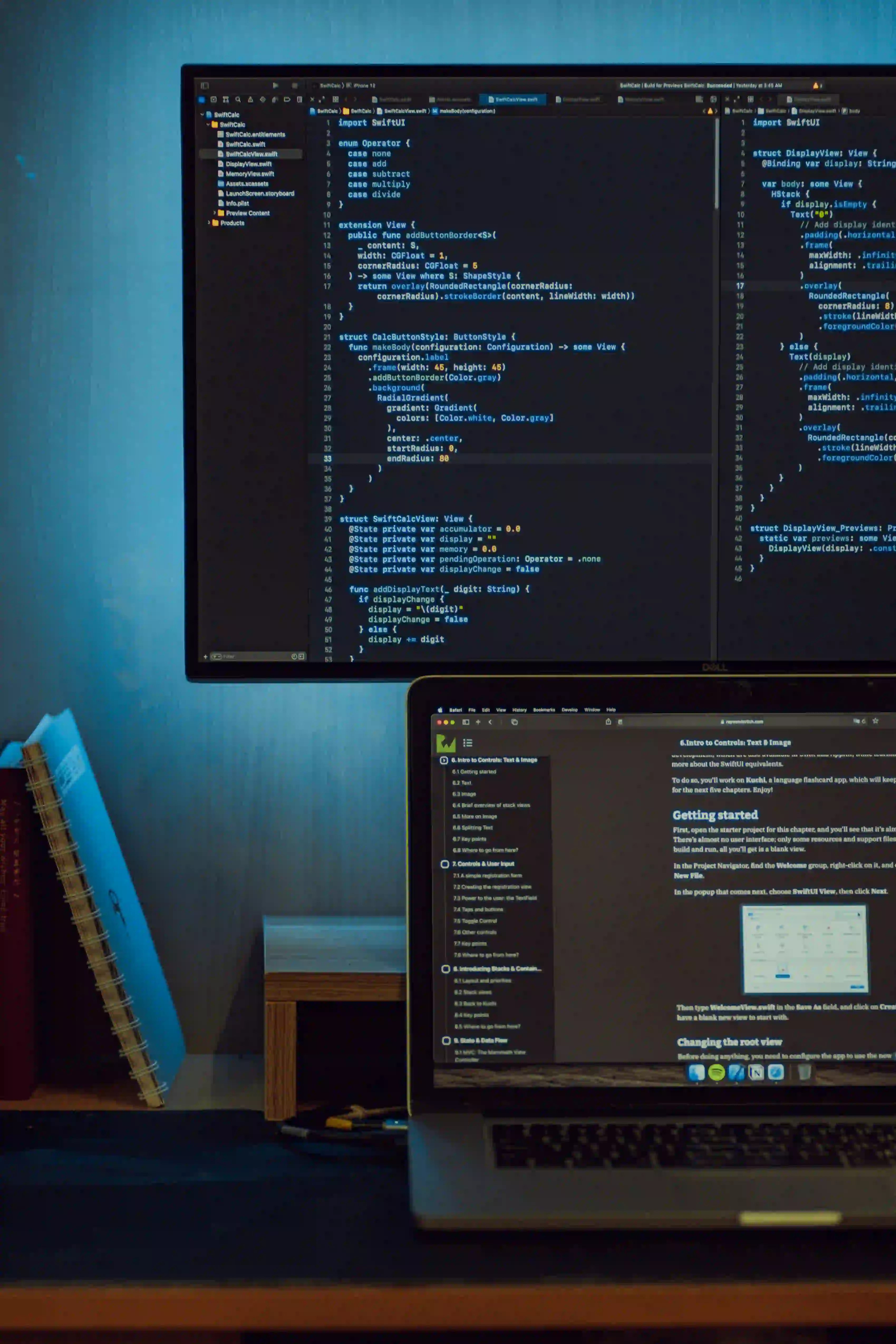
Optimizing Garbage Collection for Low CPU Overhead
When it comes to optimizing the performance of a Java application, one of the key areas to focus on is garbage collection. Garbage collection is essential for managing memory in Java but can also introduce CPU overhead if not optimized properly. In this post, we'll explore some strategies for optimizing garbage collection to minimize CPU overhead and improve the overall performance of your Java application.
Understanding Garbage Collection
Before we delve into optimization techniques, it's important to have a basic understanding of how garbage collection works in Java. When objects are created in Java, they reside in the heap memory. Over time, some of these objects may no longer be needed and become eligible for garbage collection.
The garbage collector identifies and removes these unused objects, freeing up memory for new object allocations. This process is crucial for preventing memory leaks and managing memory efficiently. However, the garbage collection process itself can introduce overhead, especially in terms of CPU utilization.
Types of Garbage Collectors in Java
Java provides several garbage collection algorithms, each designed to suit different types of applications and usage scenarios. Some of the commonly used garbage collectors include:
-
Serial Garbage Collector: Suitable for single-threaded applications or small-scale applications with limited resources.
-
Parallel Garbage Collector: Also known as throughput collector, it is designed for applications with medium to large-sized heaps and multi-core systems. It performs garbage collection using multiple threads, thus reducing pause times.
-
Concurrent Mark-Sweep (CMS) Garbage Collector: This collector aims to minimize pause times by doing most of the work concurrently with the application threads.
-
G1 (Garbage-First) Garbage Collector: Intended for large heap applications, G1 aims to provide both low pause times and high throughput by dividing the heap into smaller regions.
Each garbage collector has its own set of configurations and tuning options that can be adjusted to minimize the impact of garbage collection on CPU performance.
Optimization Strategies
1. Analyze Garbage Collection Logs
Before optimizing garbage collection, it's essential to gather data on how the garbage collector is performing. Enable garbage collection logging using the -Xloggc
and -XX:+PrintGCDetails
flags to generate logs that provide valuable insights into the behavior of the garbage collector.
java -Xloggc:gc.log -XX:+PrintGCDetails MyApp
By analyzing these logs, you can identify patterns such as the frequency and duration of garbage collection pauses, which will help in choosing the appropriate optimization strategy.
2. Choose the Right Garbage Collector
Selecting the most suitable garbage collector for your application is crucial. Depending on the application characteristics, you may need to experiment with different garbage collectors to find the best fit.
For example, if your application has a large heap size and low pause time requirements, G1 collector might be a good choice. On the other hand, for small-scale applications, the Serial Garbage Collector could suffice.
3. Adjust Heap Size
The size of the heap can significantly impact garbage collection performance. If the heap size is too small, frequent garbage collection cycles may occur, leading to increased CPU overhead. On the other hand, an excessively large heap can result in longer garbage collection pauses.
By analyzing garbage collection logs, you can determine the optimal heap size for your application. Adjust the initial and maximum heap size using the -Xms
and -Xmx
flags, respectively.
java -Xms2G -Xmx2G MyApp
4. Utilize Garbage Collection Ergonomics
Java provides a feature called garbage collection ergonomics, which allows the JVM to automatically select the appropriate garbage collector and heap configuration based on the characteristics of the application and the underlying hardware.
By enabling garbage collection ergonomics (-XX:+UseAdaptiveSizePolicy
), you allow the JVM to make decisions regarding the garbage collector and heap size based on runtime behavior.
5. Tune Garbage Collection Parameters
Each garbage collector comes with a set of configurable parameters that can be fine-tuned to achieve better performance. For example, you can adjust the young and old generation size, the parallelism of the collector, the garbage collection threads, and the pause time goals.
-XX:NewRatio=3 -XX:SurvivorRatio=4 -XX:ParallelGCThreads=8
It's important to note that tuning these parameters requires a good understanding of the garbage collector being used and thorough testing to ensure the desired effects are achieved.
6. Minimize Object Allocation and Promote Reuse
Frequent object allocation contributes to increased garbage collection overhead. By minimizing unnecessary object creation and promoting object reuse, you can reduce the frequency of garbage collection cycles.
Consider using object pooling and immutable objects where applicable to avoid unnecessary allocations.
7. Use Memory Profiling Tools
Utilize memory profiling tools such as VisualVM, YourKit, or JVisualVM to identify memory hotspots, memory leaks, and inefficient memory usage patterns. By understanding memory allocation and usage patterns, you can optimize object lifecycles and reduce unnecessary memory churn.
8. Consider Application-Level Optimizations
In addition to JVM-level optimizations, consider making changes at the application level to reduce memory pressure and object churn. This includes optimizing data structures, caching frequently used objects, and implementing efficient algorithms.
My Closing Thoughts on the Matter
Optimizing garbage collection for low CPU overhead is a crucial aspect of improving the performance of Java applications, particularly those with high memory churn or large heap sizes. By analyzing garbage collection behavior, selecting the right garbage collector, adjusting heap size, utilizing ergonomics, tuning parameters, minimizing object allocation, and considering application-level optimizations, you can effectively reduce the impact of garbage collection on CPU performance, ultimately leading to a more responsive and efficient application.
Remember, optimization strategies should be based on careful analysis of garbage collection logs and thorough testing to ensure the desired performance improvements are achieved.
In conclusion, optimizing garbage collection is a continuous process that requires a combination of best practices, careful observation, and fine-tuning to strike the right balance between memory management and CPU utilization.
By implementing these optimization strategies, you can ensure that garbage collection becomes an ally rather than a bottleneck in your Java applications.
Always stay mindful of the trade-offs involved in each optimization and remember that the best approach may vary depending on the specific requirements and characteristics of your application.