Managing Files and Directories in NIO 2
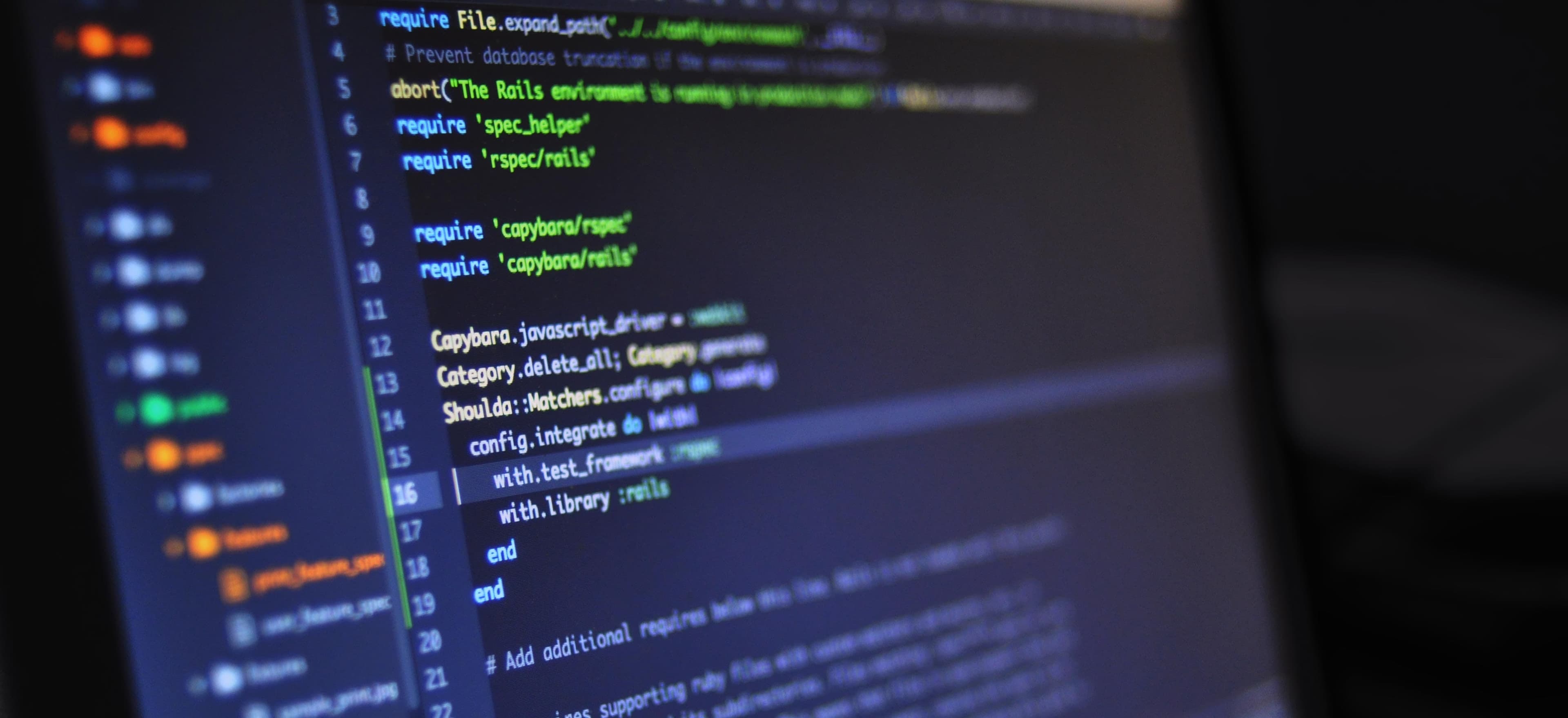
- Published on
In today's digital world, managing files and directories is a crucial part of any software application. Java, as a versatile and widely-used programming language, offers a powerful solution for file and directory management through its NIO (New I/O) 2 API. In this blog post, we'll delve into the world of NIO 2 and explore how to efficiently handle files and directories in Java applications.
What is NIO 2?
NIO 2, introduced in Java 7, provides a more extensive filesystem interface compared to the original NIO API. It offers improved support for file and directory manipulation, allowing developers to achieve tasks such as file copying, deletion, and attribute manipulation in a more efficient and streamlined manner.
Creating a File or Directory
Let's kick things off by looking at how to create a new file or directory using NIO 2. The Files
class provides various static methods for these operations. To create a directory, we can use the Files.createDirectory(Path path, FileAttribute<?>... attrs)
method. Here's a simple example:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class CreateDirectoryExample {
public static void main(String[] args) {
Path newDirectory = Paths.get("path/to/newDirectory");
try {
Files.createDirectory(newDirectory);
System.out.println("Directory created successfully!");
} catch (IOException e) {
System.err.println("Failed to create directory: " + e.getMessage());
}
}
}
In this example, we utilize the Paths
class to obtain a Path
object representing the directory we want to create. Then, we use Files.createDirectory()
to actually create the directory. If the directory creation fails, an IOException
is caught and an error message is printed.
Similarly, to create a new file, we can use the Files.createFile(Path path, FileAttribute<?>... attrs)
method.
Copying a File or Directory
NIO 2 provides a convenient way to copy files and directories using the Files.copy(Path source, Path target, CopyOption... options)
method. This method allows us to specify various options such as how to handle file attributes and how to handle file collisions.
Here's an example demonstrating how to copy a file using NIO 2:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardCopyOption;
import java.io.IOException;
public class CopyFileExample {
public static void main(String[] args) {
Path sourceFile = Paths.get("path/to/sourceFile.txt");
Path targetFile = Paths.get("path/to/targetFile.txt");
try {
Files.copy(sourceFile, targetFile, StandardCopyOption.REPLACE_EXISTING);
System.out.println("File copied successfully!");
} catch (IOException e) {
System.err.println("Failed to copy file: " + e.getMessage());
}
}
}
In this example, we use Paths.get()
to obtain Path
objects representing the source and target files. We then use Files.copy()
to perform the actual file copy operation. The StandardCopyOption.REPLACE_EXISTING
option ensures that if the target file already exists, it will be replaced.
Deleting a File or Directory
Deleting files or directories is another common operation in file management. NIO 2 provides the Files.delete(Path path)
method for this purpose. This method deletes the specified file or directory.
Let's take a look at an example of deleting a file using NIO 2:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class DeleteFileExample {
public static void main(String[] args) {
Path fileToDelete = Paths.get("path/to/fileToDelete.txt");
try {
Files.delete(fileToDelete);
System.out.println("File deleted successfully!");
} catch (IOException e) {
System.err.println("Failed to delete file: " + e.getMessage());
}
}
}
In this example, we use Paths.get()
to obtain a Path
object representing the file to delete. Then, we use Files.delete()
to delete the file. If the deletion fails, an IOException
is caught and an error message is printed.
Reading and Writing File Contents
Beyond basic file and directory manipulation, NIO 2 also provides facilities for reading and writing file contents. The Files.readAllBytes(Path path)
method can be used to read all the bytes from a file into a byte array, while the Files.write(Path path, byte[] bytes, OpenOption... options)
method can be used to write bytes to a file.
Here's an example demonstrating how to read the contents of a file using NIO 2:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.Arrays;
public class ReadFileExample {
public static void main(String[] args) {
Path fileToRead = Paths.get("path/to/fileToRead.txt");
try {
byte[] fileContents = Files.readAllBytes(fileToRead);
System.out.println("File contents: " + Arrays.toString(fileContents));
} catch (IOException e) {
System.err.println("Failed to read file: " + e.getMessage());
}
}
}
In this example, we use Paths.get()
to obtain a Path
object representing the file to read. We then use Files.readAllBytes()
to read the file contents into a byte array. If the reading operation fails, an IOException
is caught and an error message is printed.
The Closing Argument
In this blog post, we've covered various aspects of managing files and directories using NIO 2 in Java. From creating and copying files to deleting them and reading their contents, NIO 2 provides a comprehensive set of tools for efficient file and directory manipulation.
By leveraging the power of NIO 2, developers can build robust and reliable file-based operations in their Java applications. Whether it's handling user-uploaded files in a web application or managing configuration files in a desktop application, NIO 2 equips developers with the necessary tools to tackle file and directory management with ease.
NIO 2 is an essential part of modern Java development, and mastering it can significantly enhance a developer's ability to create efficient and scalable file-based operations. So, next time you find yourself dealing with file and directory management in Java, remember the power of NIO 2 at your fingertips.
To dive deeper into NIO 2 and its capabilities, you can explore the official documentation and experiment with its various methods and options. Happy coding!