Resolving Infinity Bad Default Timeout Issues
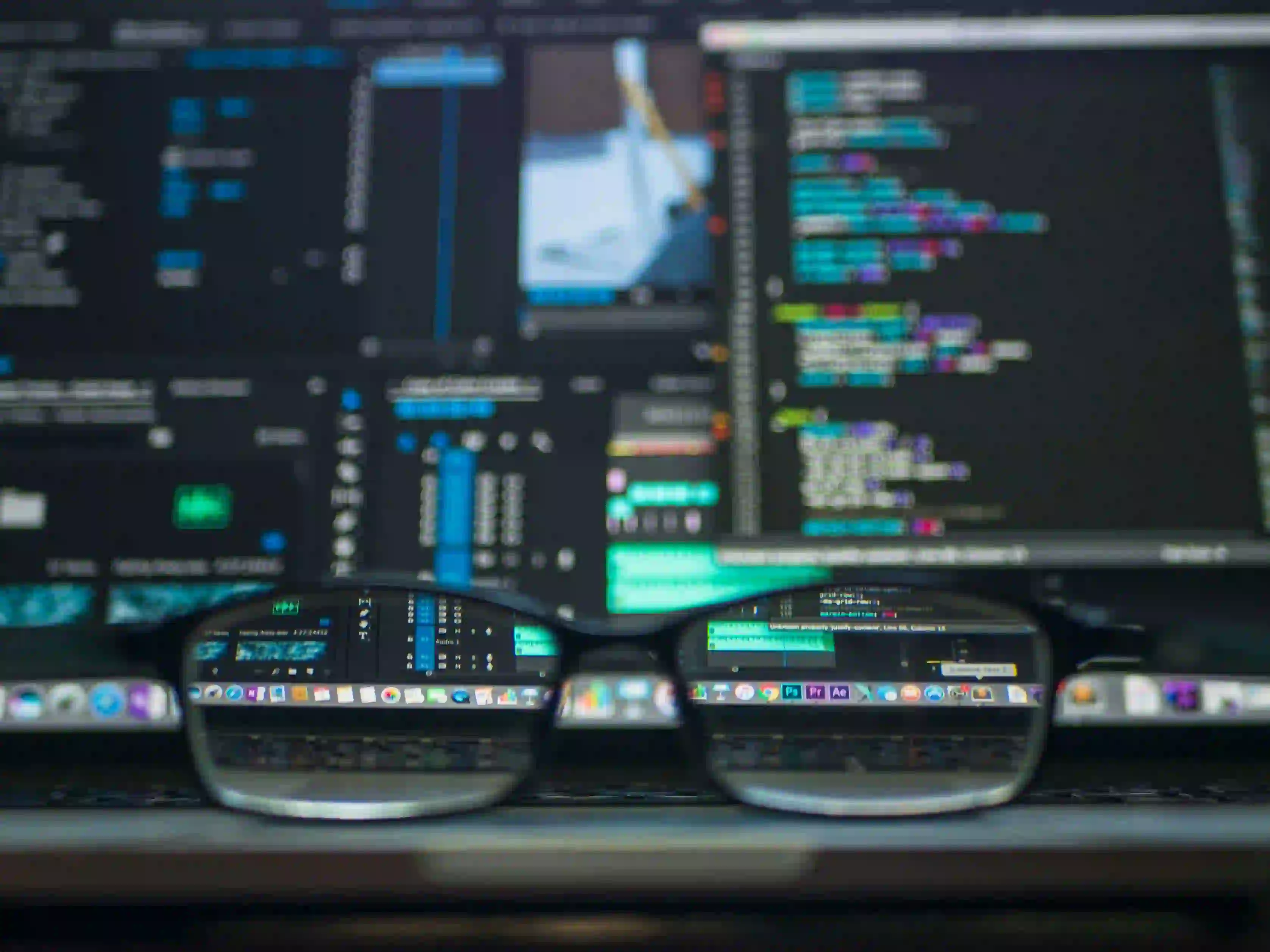
Resolving Infinity Bad Default Timeout Issues
In Java applications, managing timeouts is essential to ensure optimal performance and prevent potential issues such as indefinite waiting or resource wastage. However, the default timeout settings can sometimes lead to problems, especially when dealing with network requests or external services. One common issue is the "Infinity Bad Default Timeout," where the application stalls indefinitely due to improper or missing timeout configurations.
In this article, we'll explore the Infinity Bad Default Timeout issue, its impact, and how to resolve it effectively in Java applications.
Understanding the Infinity Bad Default Timeout Issue
When a Java application makes network requests or communicates with external services, it typically includes timeout configurations to define the maximum time it will wait for a response. If these timeouts are not explicitly set or are set to an excessively large value (often denoted by "Infinity"), the application can experience the Infinity Bad Default Timeout issue.
This issue manifests as the application waiting indefinitely for a response, leading to potential resource exhaustion, degraded performance, and unresponsiveness.
Impact of the Infinity Bad Default Timeout Issue
The Infinity Bad Default Timeout issue can have significant repercussions on the reliability and stability of Java applications, particularly those relying on network operations. The impact includes:
-
Resource Exhaustion: Indefinite waiting can lead to the exhaustion of system resources, such as threads and connection pools, hindering the application's ability to handle additional requests.
-
Degraded Performance: The prolonged waiting time can degrade overall system performance, causing delays in processing subsequent operations and negatively impacting user experience.
-
Unresponsiveness: With the application locked in infinite waiting, it becomes unresponsive and fails to fulfill its intended functions, adversely affecting end-users and downstream services.
Resolving the Infinity Bad Default Timeout Issue
To address the Infinity Bad Default Timeout issue in Java applications, it's crucial to implement appropriate timeout configurations for network requests and external service interactions. Let's explore some effective approaches to resolve this issue.
1. Setting Connection and Read Timeouts
When utilizing classes like HttpURLConnection
or HttpClient
for making HTTP requests, it's essential to set connection and read timeouts explicitly. Failure to do so can result in the default timeout values being used, potentially leading to the Infinity Bad Default Timeout issue.
Example: Setting Connection and Read Timeouts in HttpURLConnection
HttpURLConnection connection = (HttpURLConnection) new URL("https://example.com/api").openConnection();
connection.setConnectTimeout(5000); // 5 seconds
connection.setReadTimeout(10000); // 10 seconds
In this example, we set the connection timeout to 5 seconds and the read timeout to 10 seconds, ensuring that the application does not wait indefinitely for a response.
2. Using Timeouts with External Service Clients
When integrating with external services or APIs, leveraging timeout configurations provided by client libraries is vital. For instance, popular libraries like Retrofit for RESTful API consumption in Java offer configurable timeout settings that should be utilized appropriately.
Example: Setting Timeout in Retrofit Client
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.client(new OkHttpClient.Builder()
.connectTimeout(5, TimeUnit.SECONDS)
.readTimeout(10, TimeUnit.SECONDS)
.build())
.build();
By specifying the connect and read timeouts within the OkHttpClient instance, the Infinity Bad Default Timeout issue is mitigated, enhancing the robustness of the application's interactions with external services.
3. Applying Circuit Breaker Patterns
Implementing circuit breaker patterns, such as those offered by resilience4j or Hystrix, can effectively prevent the propagation of Infinity Bad Default Timeout issues. These patterns enable the application to handle failures and timeouts gracefully, thereby maintaining resilience in the face of network-related challenges.
Example: Using Resilience4j CircuitBreaker
CircuitBreaker circuitBreaker = CircuitBreaker.ofDefaults("externalService");
CheckedFunction0<String> supplier = CircuitBreaker.decorateCheckedSupplier(callingExternalService::performOperation);
Try<String> result = Try.of(CircuitBreaker.decorateCheckedSupplier(callingExternalService::performOperation))
.recover(throwable -> "Fallback response");
By incorporating a circuit breaker with appropriate fallback mechanisms, the application can proactively manage timeouts and failures, reducing the risk of prolonged waiting periods.
Key Takeaways
In Java applications, addressing the Infinity Bad Default Timeout issue is pivotal for maintaining robustness and responsiveness, especially in scenarios involving external communications. By applying explicit timeout configurations, leveraging client library features, and employing resilience patterns, the potential repercussions of indefinite waiting can be effectively mitigated.
Implementing these strategies not only enhances the reliability and performance of the application but also contributes to a more resilient and responsive ecosystem for end-users and downstream dependencies.
Resolving the Infinity Bad Default Timeout issue in Java applications is a proactive step towards ensuring optimal operational integrity and mitigating the impact of network-related challenges.
To delve deeper into this topic, you can refer to the official documentation of java.net.HttpURLConnection for comprehensive insights into managing timeouts for HTTP connections in Java.
In addition, exploring prominent libraries such as Retrofit and resilience4j can provide valuable guidance on incorporating advanced timeout management and resilience patterns in Java applications.