Optimizing Code Splitting for J2CL: Best Practices
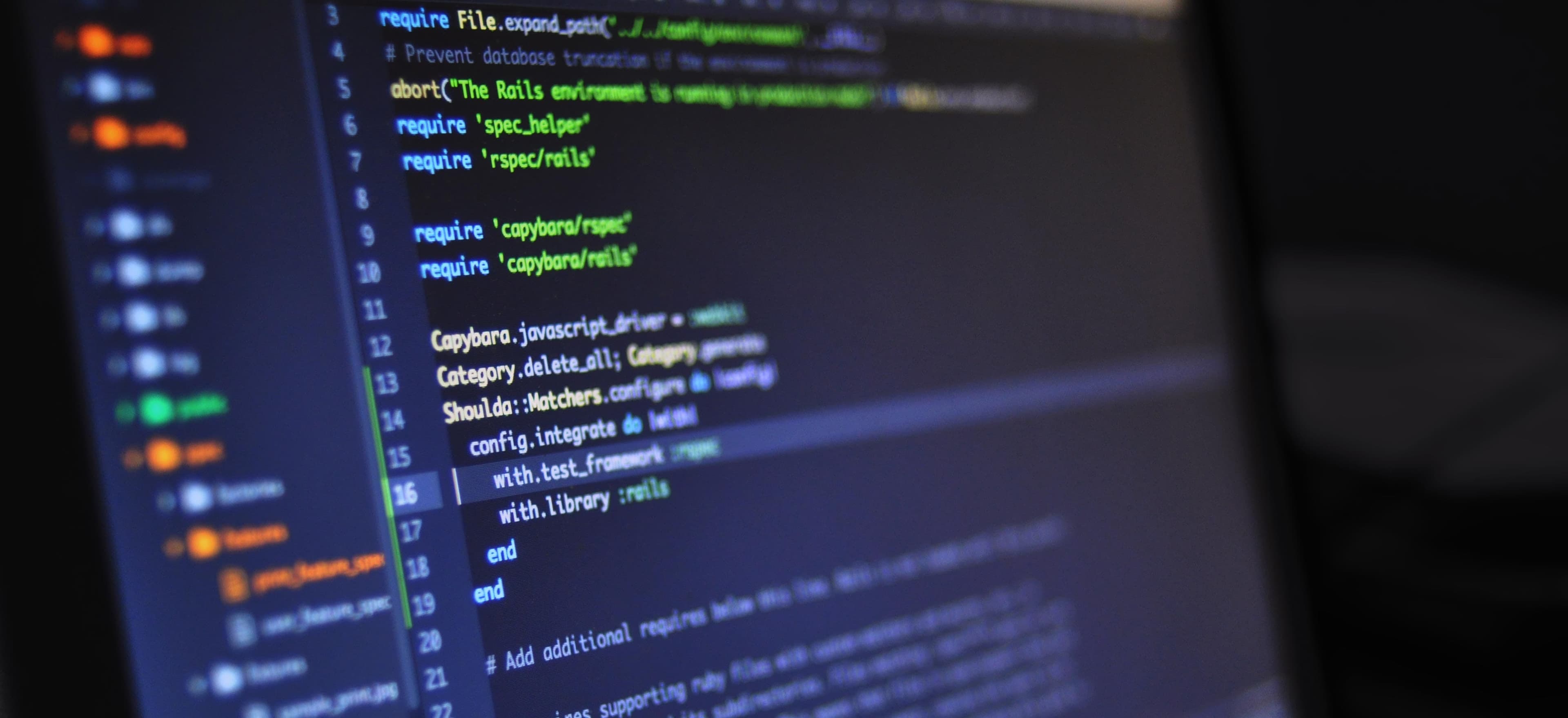
- Published on
Optimizing Code Splitting for J2CL: Best Practices
When it comes to optimizing the performance of your Java applications, code splitting plays a crucial role. Code splitting allows you to divide your codebase into smaller chunks, which can be loaded on-demand, resulting in faster initial load times and improved overall user experience. In this article, we will explore some best practices for optimizing code splitting in J2CL, a tool for transpiling Java to JavaScript.
Understanding Code Splitting in J2CL
In J2CL, code splitting is achieved through the use of @JsType
annotations and the deferred-binding
mechanism. By annotating your Java classes with @JsType(isNative = true, namespace = JsPackage.GLOBAL)
, you can indicate that a particular class should be loaded on-demand. When the transpiler encounters these annotations, it generates corresponding JavaScript code that enables code splitting.
Best Practices for Optimizing Code Splitting
1. Identify Critical Dependencies
Before applying code splitting, it's essential to identify the critical dependencies in your application. Critical dependencies are the modules or classes that are required for the initial rendering of the application. These dependencies should be loaded eagerly to ensure a smooth user experience. Use tools like Chrome DevTools' Network tab or Lighthouse to identify critical resources and optimize their loading.
2. Establish Code Splitting Boundaries
Identify natural boundaries within your application that can serve as code splitting points. For example, a complex feature within your application can be a good candidate for code splitting. By breaking down your application into logical chunks, you can create smaller bundles that are loaded on-demand, leading to faster initial load times.
3. Leverage Asynchronous Loading
J2CL provides support for asynchronous loading of code-split modules using the GWT.runAsync
method. By utilizing this feature, you can dynamically load modules when needed, reducing the initial payload size and improving the application's time-to-interactivity. Let's take a look at an example of how to use GWT.runAsync
:
GWT.runAsync(
() -> {
// Code to load the module asynchronously
}
);
In this example, the code inside the lambda function will be loaded asynchronously when GWT.runAsync
is invoked.
4. Avoid Over-Splitting
While code splitting is beneficial for optimizing loading times, over-splitting can lead to increased overhead due to the additional HTTP requests required to load the split modules. It's important to strike a balance between code splitting and minimizing the number of requests. Analyze the size and frequency of module usage to determine the optimal splitting strategy.
5. Utilize Dynamic Imports
Dynamic imports in JavaScript allow you to import modules on-demand. J2CL provides support for dynamic imports through the @JsAsync
annotation. By annotating your Java class with @JsAsync
, you can instruct the transpiler to generate dynamic import statements in the resulting JavaScript code, enabling asynchronous loading of the annotated class.
In Conclusion, Here is What Matters
Optimizing code splitting in J2CL is essential for improving the performance of your Java applications, especially when targeting web environments. By following the best practices outlined in this article, you can effectively leverage code splitting to reduce initial load times, enhance user experience, and ensure efficient resource utilization.
In conclusion, identifying critical dependencies, establishing code splitting boundaries, leveraging asynchronous loading, avoiding over-splitting, and utilizing dynamic imports are key strategies for optimizing code splitting in J2CL.
For further insights into code splitting and its impact on web performance, you can refer to the Google Developers documentation and J2CL official documentation.
Implement these best practices in your J2CL projects to harness the power of code splitting and deliver high-performing Java applications in web environments.