Java 9 Modular Services: Implementing Service Providers
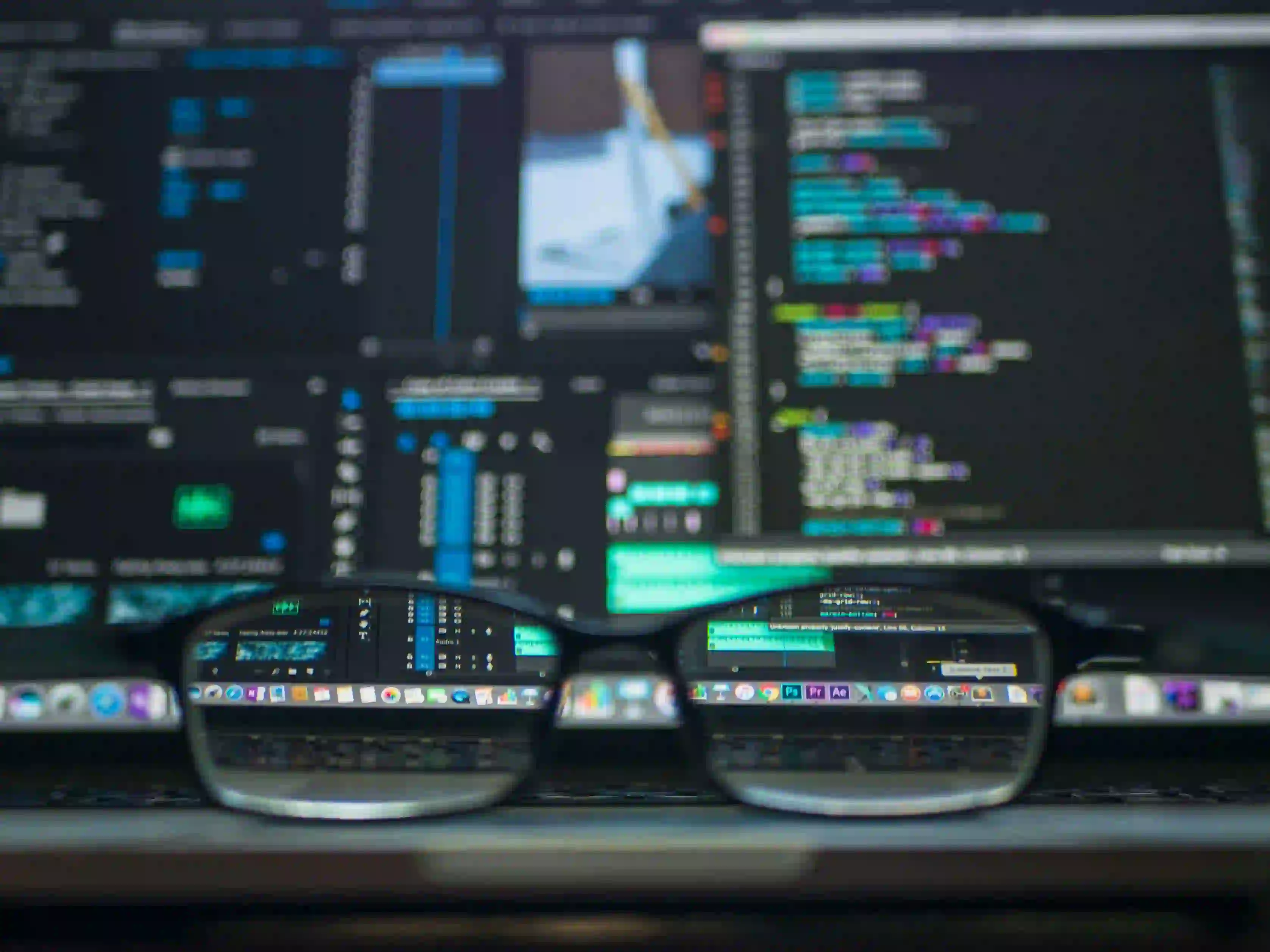
Java 9 Modular Services: Implementing Service Providers
In Java 9, the introduction of modules brought a significant shift in the way developers organize and structure their code. One key aspect of modules is the ability to define services and the providers that implement those services. This blog post will explore the implementation of service providers in Java 9 modules, providing a step-by-step guide and code examples.
Understanding Services and Service Providers
In the context of Java 9 modules, a service is defined as a well-known set of interfaces and classes. A service provider, on the other hand, is an implementation of a particular service. The goal of defining services and service providers is to decouple the service interface from its implementation, allowing for more flexible and modular code.
Creating a Service Interface
Let's begin by creating a simple service interface. In this example, we'll create an interface called NotificationServcie
that declares a method for sending notifications.
public interface NotificationService {
void sendNotification(String message);
}
The NotificationService
interface declares a method sendNotification
to send a notification message. This interface will serve as the contract for all service providers.
Implementing a Service Provider
Next, we'll create a service provider that implements the NotificationService
interface. For this example, we'll create a class called EmailNotificationService
as our service provider.
public class EmailNotificationService implements NotificationService {
@Override
public void sendNotification(String message) {
// Implement email notification logic here
System.out.println("Email notification sent: " + message);
}
}
The EmailNotificationService
class implements the NotificationService
interface and provides the implementation for the sendNotification
method, in this case, sending an email notification.
Creating a Module
In order to use services and service providers, we need to define a module. Let's create a module called notification.module
that will contain our service interface and service provider.
module notification.module {
exports com.example.notification;
provides com.example.notification.NotificationService with com.example.notification.EmailNotificationService;
}
In this module declaration, we use the provides
directive to specify that the EmailNotificationService
class is the provider of the NotificationService
interface.
Consuming the Service
Now that we have defined our service and service provider within a module, let's create a client module that consumes the service. We'll create a module called client.module
and use the uses
directive to indicate that it consumes the NotificationService
interface.
module client.module {
requires notification.module;
uses com.example.notification.NotificationService;
}
With the uses
directive, the client.module
effectively declares its dependency on the NotificationService
interface provided by the notification.module
.
Using the Service Provider
In a class within the client.module
, we can now use the ServiceLoader
to load and use the available service provider for NotificationService
.
public class Main {
public static void main(String[] args) {
ServiceLoader<NotificationService> serviceLoader = ServiceLoader.load(NotificationService.class);
for (NotificationService service : serviceLoader) {
service.sendNotification("Hello, world!");
}
}
}
The ServiceLoader
class is used to load all available service providers for a given service interface. In this example, when the Main
class is executed, it will load the EmailNotificationService
as the provider for NotificationService
and invoke the sendNotification
method.
My Closing Thoughts on the Matter
In Java 9, modular services provide a powerful way to decouple the service interface from its implementation. By defining services and service providers within modules, developers can create more flexible and modular code. This blog post has covered the basics of implementing service providers in Java 9 modules, from creating a service interface to consuming the service in a client module. By following these steps and principles, developers can harness the full potential of modular services in Java 9.
To dive deeper into Java 9 modules and modular programming, check out the official Oracle documentation for comprehensive insights.
Start leveraging Java 9 modular services today and unlock the full potential of modular programming!