Effective Strategies for Restful API Integration Testing
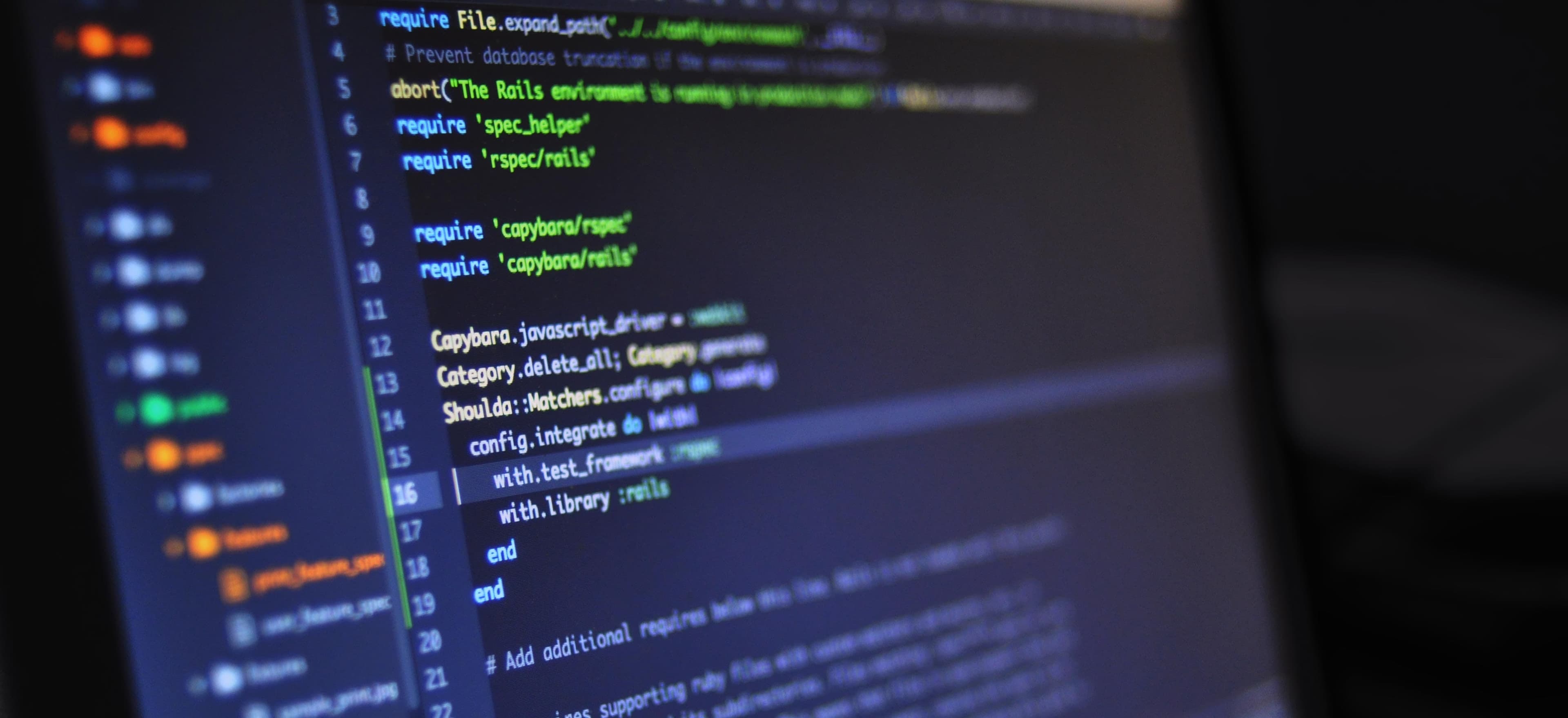
- Published on
Strategies and Best Practices for Restful API Integration Testing
When it comes to Restful API integration testing, there are various challenges and complexities involved. In this blog post, we will discuss effective strategies and best practices to ensure seamless integration testing for Restful APIs using Java.
A Brief Overview to Restful API Integration Testing
Restful APIs have become the backbone of modern web and mobile applications. As the number of APIs being developed and consumed continues to grow, the need for thorough integration testing becomes increasingly important. Integration testing ensures that APIs work as expected when interacting with other systems and services.
Choosing the Right Testing Framework
Choosing the right testing framework is crucial for effective Restful API integration testing in Java. Among the most popular choices are JUnit and TestNG. Both frameworks provide robust support for writing and executing integration tests. When choosing a testing framework, consider factors such as ease of use, reporting capabilities, and integration with other tools.
@Test
public void testAPICall() {
// Implement API call and assertions here
// Make assertions using JUnit or TestNG assertions
}
Mocking Dependencies and External Services
One key challenge in Restful API integration testing is dealing with dependencies and external services. Mocking these dependencies can help create a controlled environment for testing. Libraries such as Mockito and WireMock are powerful tools for creating mock objects and simulating external services' behavior.
@Test
public void testAPICallWithMockedDependency() {
SomeDependency mockDependency = Mockito.mock(SomeDependency.class);
// Set up mock behavior
// Inject mockDependency into the API client
// Make assertions based on the mock behavior
}
Generating and Managing Test Data
Generating and managing test data is an essential aspect of integration testing. Tools like Faker and RandomizedTesting can be used to create diverse and realistic test data. Additionally, using in-memory databases or dedicated test databases can help isolate test data from production environments.
Running Tests in Isolation
Running integration tests in isolation is crucial to ensure that each test case is independent and does not interfere with others. This can be achieved by resetting the database state before each test or utilizing containerization technologies like Docker to create isolated test environments.
Handling Authentication and Authorization
Restful APIs often involve authentication and authorization mechanisms. When testing such APIs, it's essential to handle authentication tokens, user roles, and permissions. Libraries like Spring Security provide utilities for simulating authentication and authorization in integration tests.
Continuous Integration and Deployment (CI/CD) Pipelines
Integrating Restful API integration tests into CI/CD pipelines is essential for ensuring the stability and reliability of the API. Tools such as Jenkins, GitLab CI, or Travis CI can be used to automate test execution upon code commits and deployments. This ensures that any code changes do not break existing API integrations.
Monitoring and Reporting
Effective monitoring and reporting are fundamental to understanding the health and performance of Restful APIs. Integration tests should be integrated with monitoring tools like Prometheus or New Relic to capture performance metrics during test execution. Additionally, generating comprehensive test reports using frameworks like ExtentReports or Allure can provide valuable insights into test results.
In Conclusion, Here is What Matters
In conclusion, Restful API integration testing in Java requires a robust strategy and adherence to best practices. Choosing the right testing framework, mocking dependencies, generating realistic test data, running tests in isolation, handling authentication and authorization, integrating with CI/CD pipelines, and implementing monitoring and reporting are all critical components of a successful integration testing strategy.
By following these strategies and best practices, development teams can ensure the reliability, security, and performance of their Restful APIs, leading to a seamless experience for end users and integration partners.
For further reading on Restful API integration testing, consider exploring this insightful article on Effective Strategies for API Testing.