Efficient Configuration of Spring and Hibernate
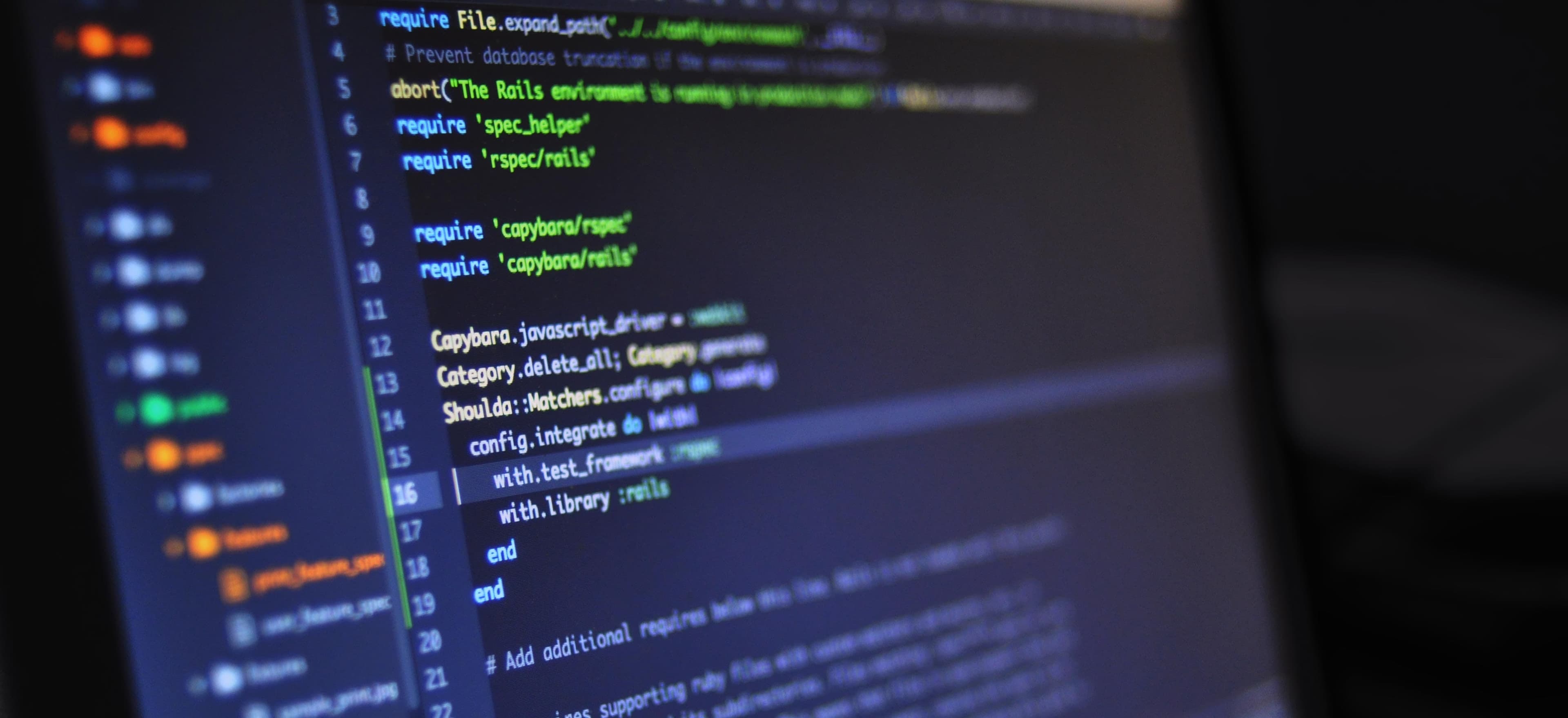
- Published on
Optimizing Spring and Hibernate Configuration for High Performance
When working with Java applications, it's crucial to ensure that the configuration of frameworks such as Spring and Hibernate is optimized for high performance. In this article, we'll explore some best practices for configuring Spring and Hibernate to achieve optimal efficiency, resulting in a more responsive and scalable application.
Efficient Configuration of Spring
1. Use Annotation-Based Configuration
Why: Annotation-based configuration reduces the verbosity of XML configuration and provides a more concise and readable way to configure Spring beans, components, and dependencies.
@Configuration
@ComponentScan("com.example")
public class AppConfig {
// Bean configurations
}
2. Implement Lazy Loading
Why: By utilizing lazy loading, beans are only created when they are actually needed, reducing startup time and memory consumption.
@Bean
@Lazy
public SomeBean someBean() {
return new SomeBean();
}
3. Leverage Spring Profiles
Why: Spring profiles allow you to have different bean configurations for different environments, enabling seamless switching between development, testing, and production configurations.
@Configuration
@Profile("dev")
public class DevDataSourceConfig {
// Development datasource configuration
}
@Configuration
@Profile("prod")
public class ProdDataSourceConfig {
// Production datasource configuration
}
4. Utilize Caching
Why: Introducing caching mechanisms such as Spring's @Cacheable
annotation can significantly improve performance by caching the results of expensive operations.
@Cacheable("products")
public Product getProductById(Long id) {
// Expensive database operation
}
Efficient Configuration of Hibernate
1. Batch Processing for Inserts and Updates
Why: Batch processing reduces the overhead of database communication by batching multiple insert or update statements into a single transaction.
<property name="hibernate.jdbc.batch_size" value="25" />
<property name="hibernate.order_inserts" value="true" />
<property name="hibernate.order_updates" value="true" />
2. Enable Second-Level Caching
Why: Second-level caching reduces the number of database hits by caching entity data, resulting in improved read performance.
@Cacheable
@Entity
public class Product {
// Entity mapping
}
3. Configure Connection Pooling
Why: Proper connection pool configuration ensures efficient management of database connections, preventing resource exhaustion and minimizing connection creation overhead.
<property name="hibernate.connection.pool_size" value="20" />
<property name="hibernate.c3p0.min_size" value="5" />
<property name="hibernate.c3p0.max_size" value="20" />
4. Fine-Tune Fetching Strategies
Why: Optimizing fetching strategies helps control the amount of data loaded from the database, preventing the retrieval of unnecessary data and reducing network overhead.
@OneToMany(fetch = FetchType.LAZY)
public List<Order> orders;
Closing Remarks
By following these best practices for configuring Spring and Hibernate, you can ensure that your Java application operates with optimal efficiency and performance. Leveraging annotation-based configuration, lazy loading, caching, batch processing, and connection pooling, among other techniques, will contribute to a more responsive and scalable system.
Implementing these strategies not only enhances the performance of your application but also contributes to a more maintainable and robust codebase, providing a solid foundation for future development and expansion.
By focusing on efficient configuration, you pave the way for a high-performing Java application that meets the demands of modern, resource-intensive use cases.
For further exploration of these topics, consider reviewing the official Spring documentation and the Hibernate User Guide.