Effective Logging Practices for Debugging
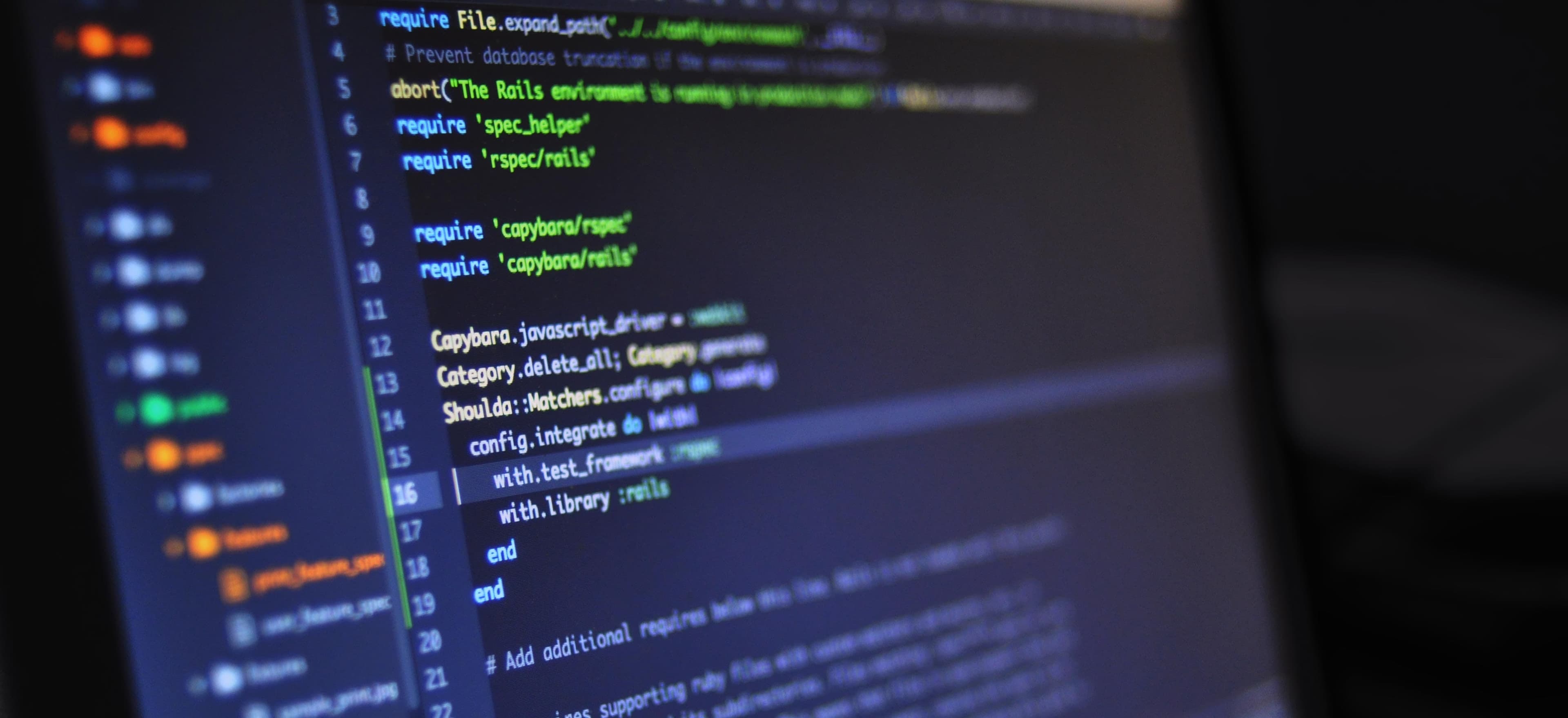
- Published on
Effective Logging Practices for Debugging
In the world of Java development, logging is an essential tool for understanding the behavior of applications, especially when troubleshooting issues. Proper logging practices not only provide visibility into the application's internal workings but also aid in debugging, monitoring, and performance analysis. In this article, we'll explore the importance of logging, best practices for logging in Java, and how to effectively utilize logging for debugging purposes.
Importance of Logging
Logging is the process of recording events, actions, and state information within an application. It serves as a crucial tool for developers, as it provides a detailed trail of an application's execution, enabling better understanding and troubleshooting of issues. Whether it's a simple informational message, a warning, or an error, logging allows developers to track the flow of the application and identify potential problems.
In Java, the most commonly used logging framework is Log4j. Log4j provides a flexible logging framework and is widely adopted in the industry due to its ease of use and customization options.
Best Practices for Logging in Java
1. Use Descriptive Log Messages
When logging, it's essential to use clear and descriptive messages. This ensures that when reviewing log files, developers can easily understand the purpose and context of each logged event. For example:
// Bad practice - Unclear log message
logger.info("Data saved");
// Good practice - Descriptive log message
logger.info("User data successfully saved to the database");
2. Utilize Log Levels Appropriately
Log messages should be categorized based on their severity. Log levels such as DEBUG, INFO, WARN, ERROR, and FATAL help in prioritizing and filtering log messages. It's crucial to use log levels appropriately to ensure that the right level of detail is captured without cluttering the logs.
3. Include Exception Information
When handling exceptions, it's important to log the stack trace along with a meaningful message. This provides valuable information for debugging and understanding the cause of an error. For example:
try {
// Some code that may throw an exception
} catch (Exception e) {
logger.error("An error occurred: " + e.getMessage(), e);
}
4. Implement Log Rolling and Retention Policies
As log files can grow rapidly, it's essential to implement log rolling to manage log file size and retention policies to control the lifespan of log files. This prevents log files from consuming excessive disk space and helps maintain a manageable log history.
5. Contextual Logging
In complex applications, it's beneficial to include contextual information in log messages. This may include session IDs, user IDs, transaction IDs, or any other relevant context that aids in tracing the flow of a specific operation or request.
Using Logging for Debugging
1. Identify the Problem Area
When debugging an issue, start by identifying the specific area of the code where the problem is believed to be occurring. Logging can be instrumental in this initial phase, as strategically placed log statements can provide insights into the execution flow and the values of variables.
2. Add Debug Log Statements
Utilize DEBUG log level statements to output detailed information during the execution of the problematic code. This could include variable values, method entry and exit points, conditional checks, and any other relevant details that can help in understanding the behavior of the code.
3. Analyze Log Outputs
Once the debug log statements are in place, execute the application and monitor the log outputs. Pay close attention to the sequence of events, variable values, and any anomalies or unexpected behaviors that are logged.
4. Iterative Refinement
Based on the analysis of the log outputs, make iterative changes to the debug log statements to gather more specific or relevant information. Refine the log messages to capture the necessary details that can aid in pinpointing the root cause of the issue.
5. Utilize Log Analysis Tools
In addition to manual log analysis, consider using log analysis tools and frameworks such as ELK Stack or Splunk. These tools offer advanced log aggregation, search, and visualization capabilities, enabling efficient analysis of log data for debugging and monitoring purposes.
In Conclusion, Here is What Matters
In conclusion, effective logging practices are paramount for debugging and understanding the behavior of Java applications. By adhering to best practices such as using descriptive log messages, appropriate log levels, and contextual logging, developers can leverage logging as a powerful tool for troubleshooting and gaining insights into application execution. When used in conjunction with deliberate debugging techniques, logging enhances the ability to identify, analyze, and resolve issues within Java applications.
When used correctly, logs can be an invaluable source of information, guiding developers through the intricacies of their applications and providing clarity in times of uncertainty.
Logging is not just about recording events; it's about shedding light on the behavior of the application, unveiling its inner workings, and paving the way for effective debugging and maintenance.
So, log wisely and debug efficiently!