Handling Command Line Arguments in Java
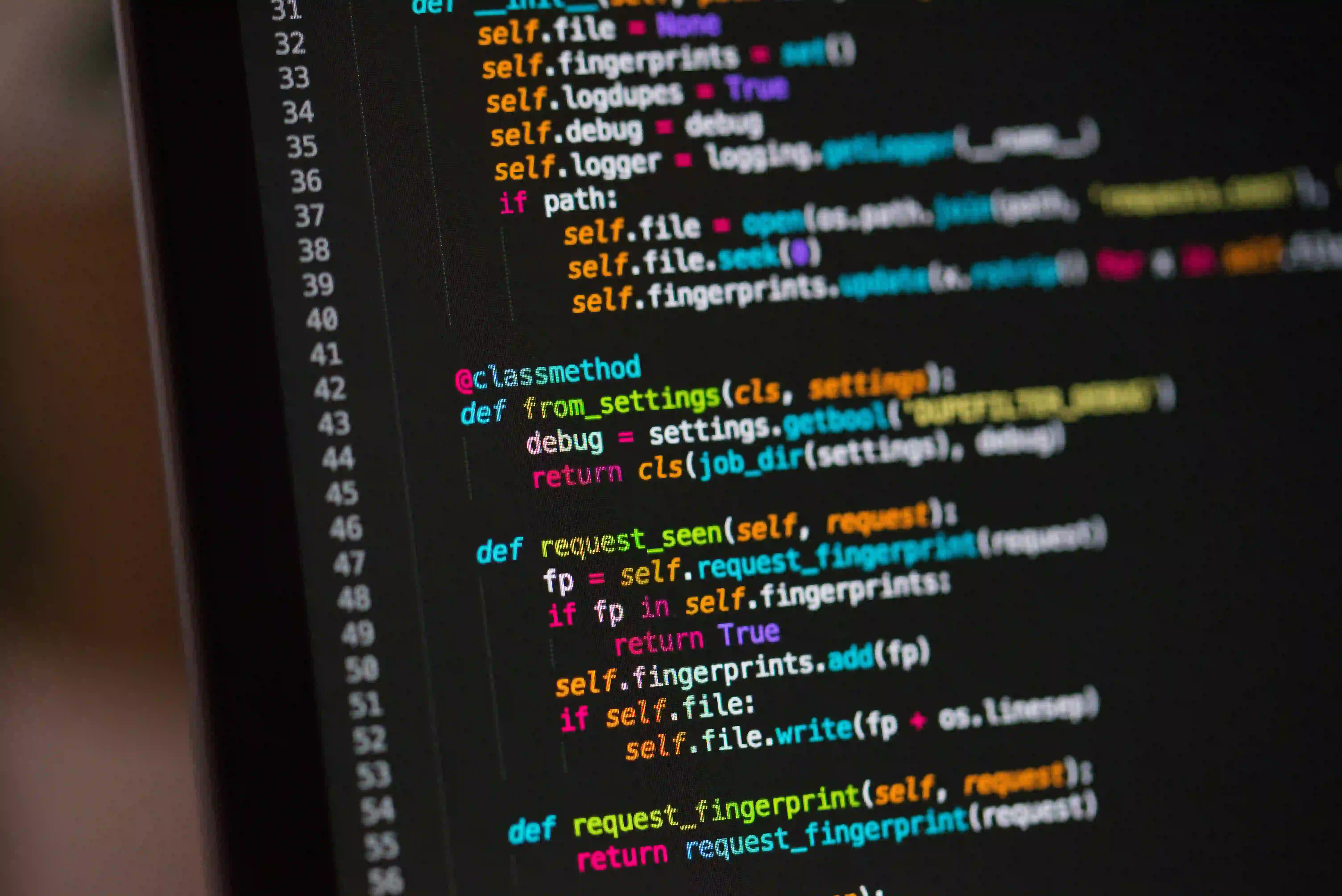
Command line arguments are a fundamental aspect of many Java applications, enabling developers to pass parameters to a program when it is executed. By leveraging command line arguments, developers can enhance the versatility and customizability of their software. In this article, we will explore the fundamentals of working with command line arguments in Java, discussing how to parse and utilize them effectively in your applications.
What are Command Line Arguments?
Command line arguments are values provided to a program when it is invoked from the command line or terminal. They allow users to customize the behavior of an application without requiring modifications to the source code. In Java, command line arguments are passed to the main
method of a class when the program is launched.
Accessing Command Line Arguments in Java
To access command line arguments in a Java program, we can use the args
parameter of the main
method. This parameter is an array of strings that contains the arguments passed to the program. Let's take a look at a simple example of a Java program that accesses and utilizes command line arguments:
public class CommandLineArgumentsExample {
public static void main(String[] args) {
System.out.println("Number of arguments: " + args.length);
for (String arg : args) {
System.out.println("Argument: " + arg);
}
}
}
In this example, the main
method accepts an array of strings args
. We then print the number of arguments passed and iterate through the array to display each argument.
Parsing Command Line Arguments
While accessing command line arguments is straightforward, parsing and interpreting them based on the requirements of the application necessitates additional consideration. This is where libraries such as Apache Commons CLI can be valuable, as they provide a robust set of tools for parsing and managing command line arguments in a structured and maintainable manner.
Let's consider a scenario where a Java application needs to accept command line arguments for configuring connection properties, such as the server address, port, username, and password. Here's how we can achieve this using Apache Commons CLI:
Options options = new Options();
options.addOption(Option.builder("s").longOpt("server").hasArg().desc("Server address").build());
options.addOption(Option.builder("p").longOpt("port").hasArg().desc("Port number").type(Number.class).build());
options.addOption(Option.builder("u").longOpt("username").hasArg().desc("Username").build());
options.addOption(Option.builder("w").longOpt("password").hasArg().desc("Password").build());
CommandLineParser parser = new DefaultParser();
try {
CommandLine cmd = parser.parse(options, args);
String server = cmd.getOptionValue("s");
int port = (Integer) cmd.getParsedOptionValue("p");
String username = cmd.getOptionValue("u");
String password = cmd.getOptionValue("w");
// Use the parsed values to establish a connection
// ...
} catch (ParseException e) {
System.err.println("Error parsing command line arguments: " + e.getMessage());
// Handle the error or display usage instructions
}
In this example, we define the options our program can accept using the Options
class, and then utilize the OptionBuilder
to create individual options with specific characteristics such as short and long identifiers, whether they require arguments, and the description. We then use the CommandLineParser
to parse the command line arguments based on the defined options. If successful, we retrieve the values and proceed with establishing the connection.
Best Practices for Handling Command Line Arguments
When working with command line arguments in Java, it's essential to adhere to best practices to ensure robustness, maintainability, and user-friendliness of the application:
-
Provide Clear Usage Instructions: Make sure your program provides clear and concise usage instructions to guide users on how to utilize command line arguments effectively. This includes displaying information on the available options and their usage.
-
Validation and Error Handling: Validate and handle errors gracefully when parsing and utilizing command line arguments. This involves checking for required arguments, ensuring the correctness of the provided values, and providing informative error messages.
-
Use Libraries for Complex Parsing: For applications that require complex or advanced command line argument parsing, consider leveraging libraries such as Apache Commons CLI or picocli, which provide comprehensive tools for defining, parsing, and managing command line options.
-
Avoid Reinventing the Wheel: Instead of building custom parsing and validation mechanisms for command line arguments, leverage established libraries and frameworks to streamline the process and benefit from their robust features and ongoing support.
Final Considerations
In conclusion, working with command line arguments in Java is an essential skill for developers, enabling them to create versatile and configurable applications. By understanding how to access, parse, and utilize command line arguments effectively, developers can enhance the usability and flexibility of their software. Additionally, leveraging libraries such as Apache Commons CLI can simplify and standardize the process of handling command line arguments, leading to more maintainable and user-friendly applications.
By incorporating best practices and utilizing powerful libraries, Java developers can ensure that their applications handle command line arguments in a robust and efficient manner, ultimately enhancing the overall user experience and maintainability of the software.