5 Common Mistakes of Performance Testing
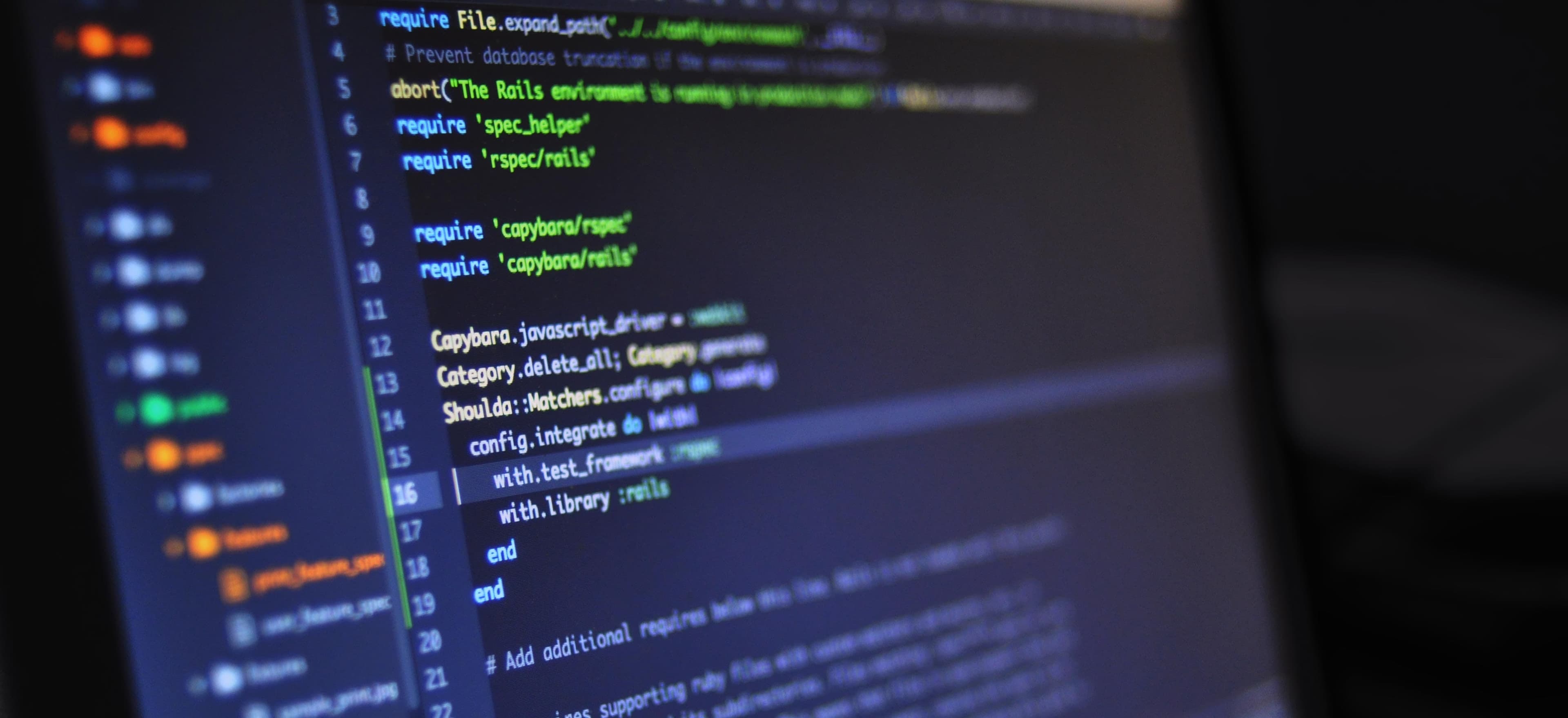
- Published on
5 Common Mistakes to Avoid in Performance Testing
Performance testing is an integral part of software development that helps ensure the reliability, scalability, and speed of an application. However, even experienced developers and testers can make common mistakes that can compromise the effectiveness of performance testing. Here are five common mistakes to avoid in performance testing.
1. Testing in an Unrealistic Environment
One of the most common mistakes in performance testing is conducting tests in an unrealistic environment. This could involve testing on a local machine with limited resources or in a testing environment that does not accurately replicate the production environment. It's essential to simulate a realistic user load and network conditions to obtain accurate performance metrics.
// Example of setting up a realistic environment for performance testing
public void setUp(){
// Use tools like JMeter to simulate real user load
// Deploy the application in a test environment that mirrors the production environment
// Emulate network conditions to mimic real-world usage
}
2. Neglecting to Set Clear Goals
Another common mistake is the failure to establish clear performance goals before conducting tests. Without clear objectives, it becomes challenging to determine whether the application meets performance expectations. It's crucial to define specific performance metrics such as response time, throughput, and resource utilization to assess the application's performance effectively.
// Example of setting clear performance goals for testing
public void setPerformanceGoals(){
// Define acceptable response time for different operations
// Determine the expected throughput under specific user loads
// Set limits for resource consumption such as memory and CPU usage
}
3. Overlooking Real User Scenarios
Performance tests often focus solely on the technical aspects of an application, overlooking real user scenarios. To truly gauge an application's performance, it's essential to incorporate real user scenarios into the testing process. This involves considering factors such as peak user load, user behavior patterns, and data variability, which can significantly impact the application's performance in a production environment.
// Example of incorporating real user scenarios into performance testing
public void considerRealUserScenarios(){
// Analyze peak user load based on historical data and business forecasts
// Replicate user behavior patterns such as simultaneous logins or database queries
// Introduce data variability to assess the application's robustness
}
4. Failing to Monitor System Resources
Inadequate monitoring of system resources during performance testing can lead to inaccuracies in identifying performance bottlenecks. It's crucial to closely monitor key system resources such as CPU, memory, disk I/O, and network usage to pinpoint potential performance issues. Utilizing performance monitoring tools and establishing baseline measurements can aid in detecting deviations from expected resource utilization.
// Example of monitoring system resources during performance testing
public void monitorSystemResources(){
// Use tools like VisualVM or JConsole to monitor JVM performance
// Implement system monitoring scripts to track CPU, memory, and disk usage
// Establish baseline measurements for comparison during performance testing
}
5. Neglecting Regular Performance Testing
A common mistake is treating performance testing as a one-time activity rather than an ongoing process. Application performance can degrade over time due to changes in code, increased user load, or infrastructure modifications. It's essential to integrate performance testing into regular testing cycles and adopt continuous performance testing practices to identify and address performance degradation proactively.
// Example of integrating regular performance testing into the development lifecycle
public void regularPerformanceTesting(){
// Include performance tests in continuous integration pipelines
// Incorporate performance testing as part of regression testing efforts
// Monitor performance metrics in production to detect gradual degradation
}
In conclusion, avoiding these common mistakes in performance testing is crucial for ensuring the accuracy and reliability of performance metrics. By testing in realistic environments, setting clear goals, incorporating real user scenarios, monitoring system resources, and integrating regular performance testing, development teams can effectively identify and address performance issues, ultimately delivering a high-performing application to end-users.
To delve deeper into performance testing best practices and tools, consider exploring resources such as Gatling and JMeter for comprehensive performance testing solutions.