Unraveling JavaMail's Disruptive Impact on App Servers
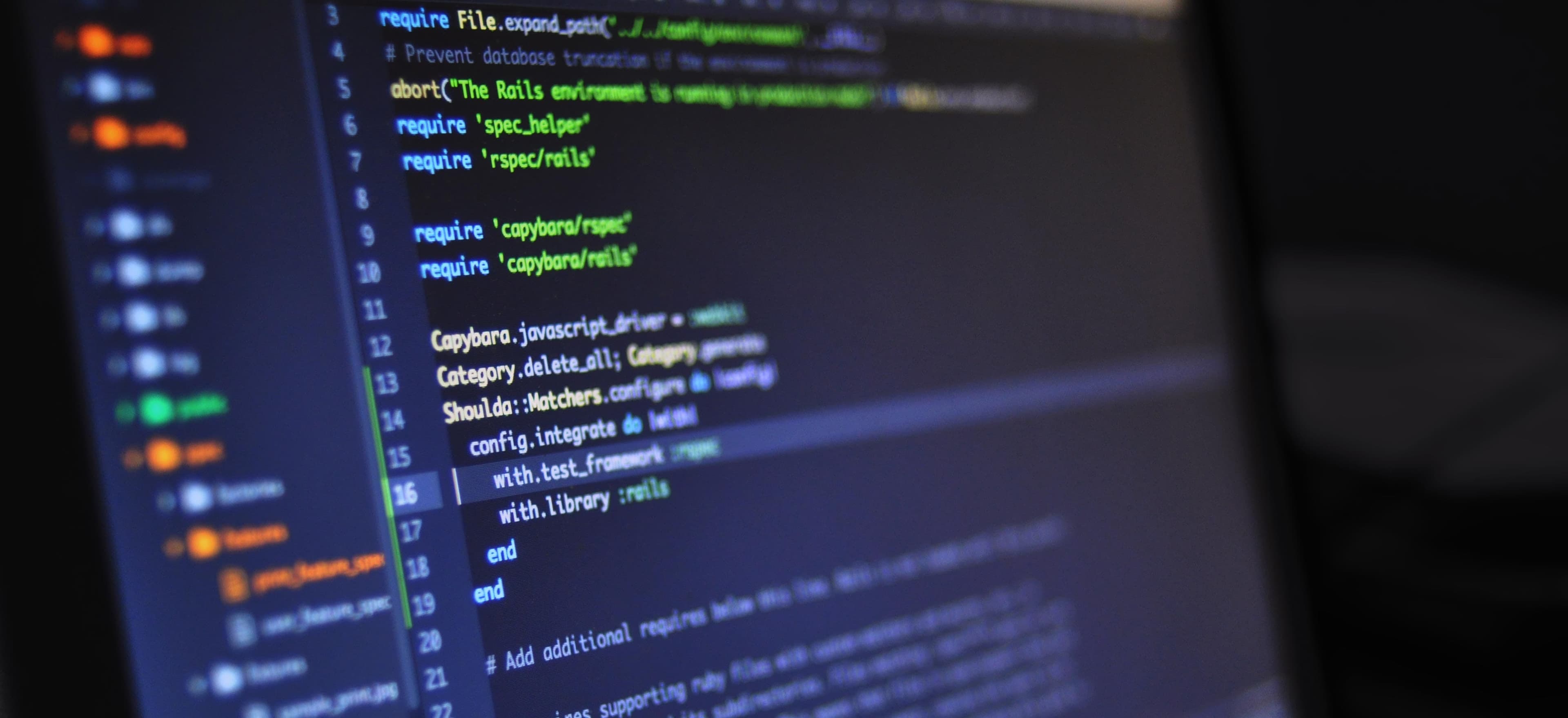
- Published on
The Disruptive Impact of JavaMail on App Servers
When it comes to enterprise Java applications, the management of emails is a crucial part of the system's functionality. JavaMail, a Java API used to compose, send, and receive email messages, has been an integral part of many applications. However, using JavaMail within an application may have a disruptive impact on the associated app servers. In this article, we will delve into the reasons behind this disruption and provide insights into how to mitigate its effects.
The Impact of JavaMail
Resource Consumption
One of the primary reasons JavaMail can disrupt app servers is its resource consumption. Sending and receiving emails can be resource-intensive operations, especially when handling large volumes of messages or attachments. This can lead to increased memory usage, CPU utilization, and I/O operations on the app server, potentially impacting the overall performance and scalability of the application.
Thread Management
Another critical factor is JavaMail's use of threads for asynchronous email processing. While this can be advantageous for handling concurrent email operations, it can also lead to thread management challenges within the app server. Improper handling of threads can result in thread contention, thread starvation, and even thread leaks, which can degrade the app server's stability and reliability.
Security Considerations
JavaMail's integration with various email protocols and servers introduces security considerations for the underlying app server. Handling authentication, encryption, and secure communication with email servers is essential for safeguarding sensitive information. Failing to address these security aspects can compromise the integrity of the app server and the confidentiality of email communications.
Mitigating the Impact
Asynchronous Execution
To mitigate the disruptive impact of JavaMail, leveraging asynchronous execution mechanisms can be beneficial. By offloading email processing to separate threads or utilizing asynchronous APIs, app servers can better manage email-related tasks without blocking the main execution flow. This approach helps in improving concurrency and responsiveness while reducing the potential for thread-related issues.
// Asynchronous email sending using CompletableFuture in Java
CompletableFuture.runAsync(() -> {
// Code for sending email
});
Resource Pooling
Resource pooling techniques, such as connection pooling for SMTP servers, can effectively manage resource consumption and optimize the utilization of email-related resources. By reusing connections and resources, app servers can minimize the overhead associated with establishing connections to email servers, ultimately enhancing performance and scalability.
// Example of using Apache Commons Pool for creating an SMTP connection pool
GenericObjectPool<Transport> pool = new GenericObjectPool<>(new TransportFactory());
Transport transport = pool.borrowObject();
try {
// Use the pooled transport for sending email
} finally {
pool.returnObject(transport);
}
Performance Tuning
Performance tuning of the app server, including memory allocation, garbage collection optimization, and thread management, is crucial for mitigating the impact of JavaMail. Fine-tuning these aspects can help ensure that the app server efficiently handles email-related operations without experiencing resource bottlenecks or performance degradation.
Security Hardening
Addressing the security considerations associated with JavaMail involves implementing secure communication protocols, enforcing authentication mechanisms, and handling sensitive data in a secure manner. Integrating encryption protocols, SSL/TLS configurations, and secure credential storage mechanisms are essential for fortifying the app server's email capabilities against potential security threats.
A Final Look
While JavaMail plays a vital role in email communication within Java applications, its disruptive impact on app servers cannot be overlooked. However, by understanding the reasons behind this impact and adopting mitigation strategies such as asynchronous execution, resource pooling, performance tuning, and security hardening, developers and system administrators can effectively manage and optimize the usage of JavaMail within their applications, ensuring a seamless and efficient email handling mechanism without compromising the stability and performance of the underlying app servers.
In conclusion, it is imperative to strike a balance between leveraging JavaMail's capabilities and mitigating its disruptive impact to uphold the overall integrity and performance of enterprise Java applications.
For further insights into JavaMail and its impact on app servers, you can refer to the Oracle's official documentation and JavaMail API documentation.