Overcoming Classroom Anxiety
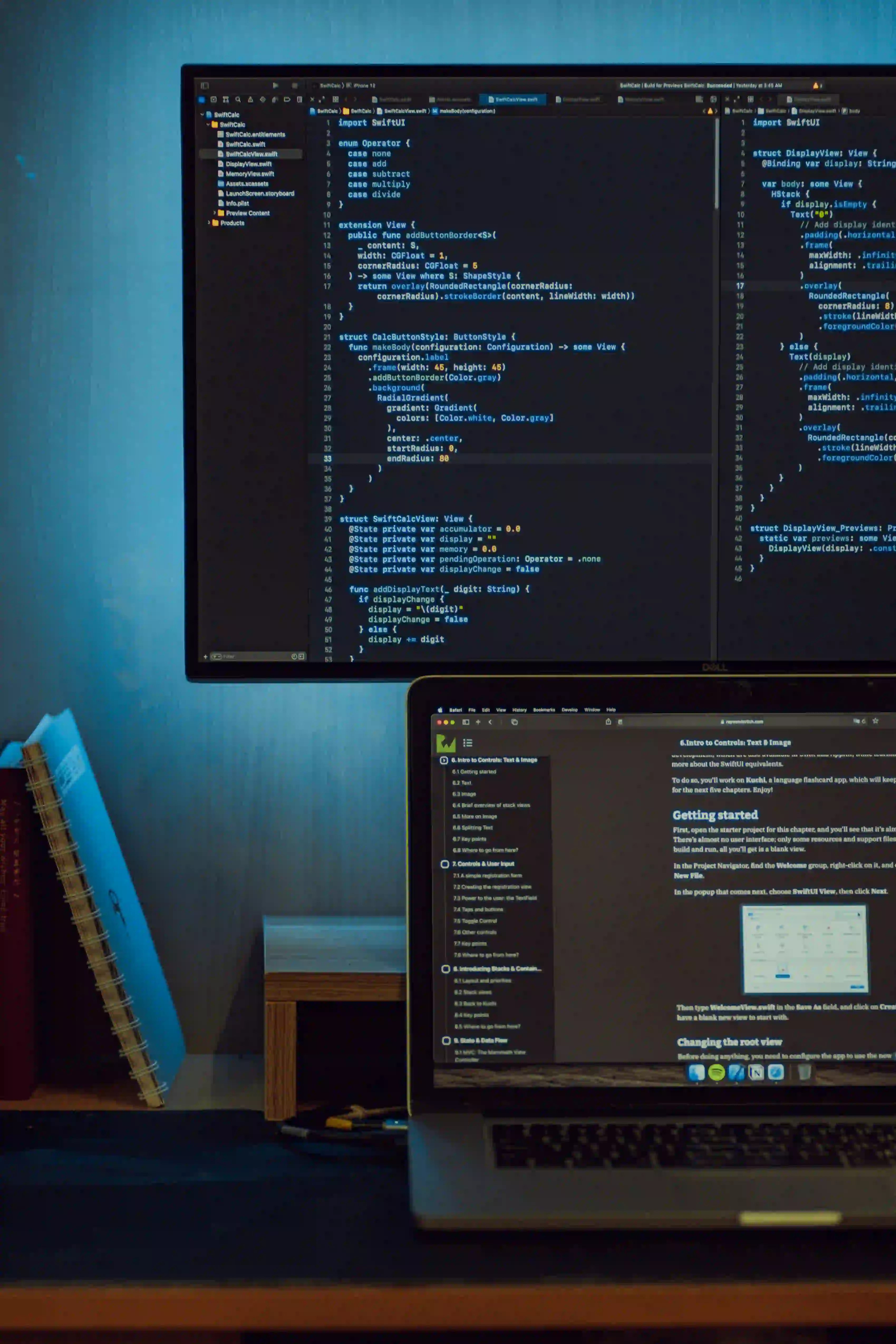
Understanding Java Reflection for Dynamic Class Loading
In the world of Java programming, reflection is a powerful tool that allows you to inspect and manipulate classes, interfaces, fields, and methods at runtime. Reflection enables you to perform operations such as examining class capabilities, instantiating objects, and calling methods dynamically, which may not be known at compile time. This blog post will delve into Java reflection and explore how it can be used for dynamic class loading.
What is Java Reflection?
Java reflection is a feature that enables you to inspect and modify classes, interfaces, fields, and methods at runtime. It provides a way to obtain information about the structure of classes and to manipulate their members, all without knowing the exact types at compile time. Reflection allows you to perform operations such as examining class capabilities, instantiating objects, and calling methods dynamically, which may not be known at compile time.
Dynamic Class Loading
Dynamic class loading refers to the ability to load classes dynamically at runtime. With reflection, you can load classes dynamically by their fully qualified names, create instances of the loaded classes, and invoke their methods without having prior knowledge of the classes at compile time. This dynamic loading of classes provides flexibility in developing applications where the classes to be used are determined at runtime.
Let's consider an example to understand the concept of dynamic class loading using Java reflection.
Example: Dynamic Class Loading
Suppose we have an interface Shape
and two classes Circle
and Rectangle
that implement the Shape
interface. Our goal is to dynamically load these classes at runtime and invoke their methods without knowing their exact types at compile time.
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing Circle");
}
}
public class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing Rectangle");
}
}
To achieve dynamic class loading and invocation of methods, we can use Java reflection as shown in the following code snippet:
public class DynamicClassLoadingExample {
public static void main(String[] args) throws ClassNotFoundException, IllegalAccessException, InstantiationException {
// Dynamically load the class by its fully qualified name
Class<?> circleClass = Class.forName("com.example.Circle");
Class<?> rectangleClass = Class.forName("com.example.Rectangle");
// Create instances of the loaded classes
Shape circle = (Shape) circleClass.newInstance();
Shape rectangle = (Shape) rectangleClass.newInstance();
// Call the draw method of each instance
circle.draw();
rectangle.draw();
}
}
In the above example, we use the Class.forName
method to dynamically load the classes by their fully qualified names. We then create instances of the loaded classes using the newInstance
method and call the draw
method on each instance. This demonstrates how reflection can be used for dynamic class loading and invocation of methods at runtime.
Why Use Dynamic Class Loading?
Dynamic class loading and reflection are powerful features that provide flexibility and extensibility to Java applications. They are commonly used in scenarios such as:
-
Plugin Systems: In applications that support plugins or dynamically loadable modules, dynamic class loading allows the application to discover and load new functionality at runtime without recompiling the code.
-
Dependency Injection: Frameworks like Spring and Guice use reflection and dynamic class loading to inject dependencies into objects at runtime based on configuration or annotations.
-
Configuration-based Behavior: Reflection can be used to load classes and configure their behavior based on external configuration, such as properties files or database settings.
-
Serialization and Deserialization: Reflection is used in serialization frameworks to dynamically inspect and manipulate object structures during the serialization and deserialization process.
Best Practices for Using Reflection and Dynamic Class Loading
While reflection and dynamic class loading provide powerful capabilities, they also come with some considerations and best practices to ensure safe and efficient usage:
-
Security: Reflection allows access to private members and can bypass visibility checks performed by the compiler. It is important to use reflection judiciously and follow security best practices to avoid unintended access to sensitive data and methods.
-
Performance: Reflection operations are generally slower than direct method calls and field accesses. It is essential to consider the performance implications of using reflection and to utilize caching for improved performance in critical sections of the code.
-
Error Handling: When working with reflection, it is crucial to handle exceptions such as
ClassNotFoundException
,NoSuchMethodException
, andIllegalAccessException
to prevent runtime failures and provide graceful error handling. -
Type Safety: Reflection bypasses compile-time type checking, which can lead to runtime type-related errors. It is advisable to use generics and type checks wherever possible to maintain type safety when working with reflection.
The Bottom Line
Java reflection and dynamic class loading open up a world of possibilities for building flexible and extensible applications. By dynamically inspecting and manipulating classes, interfaces, fields, and methods at runtime, developers can create highly adaptable systems that can evolve and accommodate new functionality without requiring extensive code changes. However, it is crucial to exercise caution and follow best practices when using reflection to ensure security, performance, and type safety in Java applications.
In this blog post, we've explored the concept of dynamic class loading using Java reflection, along with an example illustrating the dynamic loading and invocation of classes at runtime. We've also highlighted the benefits and best practices for using reflection and dynamic class loading in Java applications.
By leveraging the power of reflection and dynamic class loading, Java developers can design robust and flexible systems that can adapt to changing requirements and seamlessly integrate new functionality, making reflection a valuable tool in the developer's arsenal.
For further reading on Java reflection and dynamic class loading, check out Oracle's official documentation and the Java Reflection Tutorial.