Common Mistakes: When to Avoid Microservices
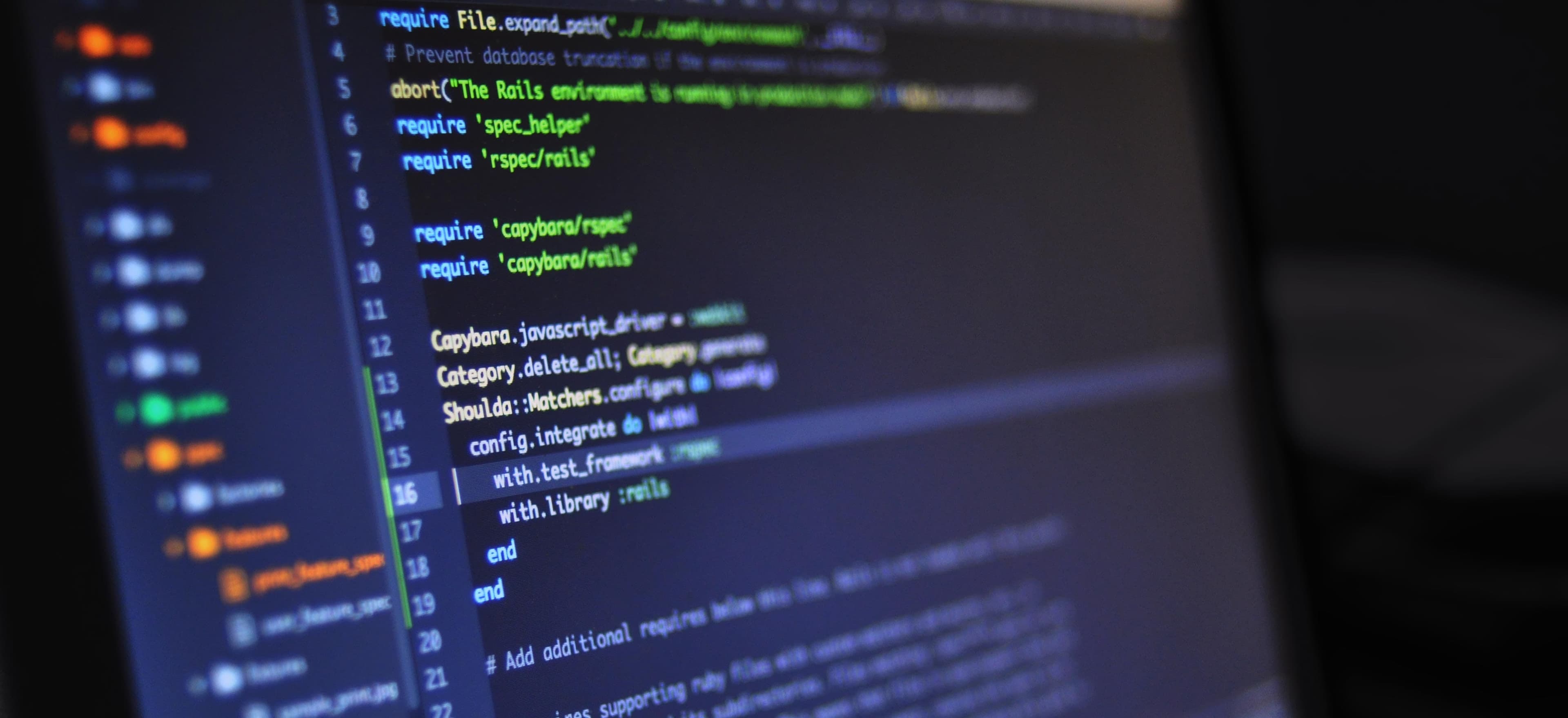
- Published on
Common Mistakes: When to Avoid Microservices
Microservices architecture has gained popularity in the software development world for its flexibility, scalability, and ability to enable continuous delivery. However, it's crucial to note that not all projects are suitable for microservices. In some cases, opting for a monolithic architecture might be a more practical choice.
In this post, we will break down some common mistakes developers make when deciding to adopt microservices, as well as scenarios in which microservices might not be the best fit. It's essential to understand these factors to make an informed decision about when to avoid the microservices approach.
1. Premature Optimization
One of the most common mistakes is jumping into microservices too early in the project lifecycle. It's crucial to remember that microservices come with added complexity in terms of deployment, monitoring, and network communication. If your project is still in its early stages and the exact boundaries of each service are not yet clear, starting with a monolithic architecture might be more beneficial.
Exemplary Code Snippet:
// Prematurely implementing microservices without clear service boundaries
public class PrematureMicroserviceImplementation {
// Service 1 code
public void service1Functionality() {
// logic
}
// Service 2 code
public void service2Functionality() {
// logic
}
}
In the above code snippet, prematurely dividing functionalities into separate microservices without a clear boundary can lead to unnecessary complexity.
Why:
Prematurely structuring code into separate microservices without well-defined boundaries can result in increased development overhead and hinder overall project progress.
2. Small, Simple Projects
Microservices are designed to tackle complex and scalable systems. If you are working on a small, straightforward project with a clear scope and minimal integration points, opting for microservices might introduce unnecessary overhead. In such cases, a monolithic architecture would be more straightforward and manageable.
Exemplary Code Snippet:
// Simple functionality within a monolithic architecture
public class MonolithicSimpleFunctionality {
public void simpleFunctionality() {
// logic
}
}
In this scenario, implementing microservices would overcomplicate the development and maintenance of the project.
Why:
For small, straightforward projects, the introduction of microservices can lead to an unnecessary increase in complexity and deployment overhead.
3. Tight Budgets and Deadlines
Microservices come with added operational overhead, as each service needs to be deployed, monitored, and maintained independently. In cases where there are budget constraints or tight deadlines, opting for a monolithic architecture can streamline the development process. Microservices, while beneficial in the long run, require additional effort in setting up infrastructure, continuous integration, and deployment pipelines.
Exemplary Code Snippet:
// Monolithic approach for rapid development
public class MonolithicRapidDevelopment {
// All-in-one functionality for quick deployment
public void allInOneFunctionality() {
// logic
}
}
In fast-paced development environments, a monolithic architecture can provide a more agile approach to meeting deadlines and budget constraints.
Why:
Microservices, due to their distributed nature, demand additional effort in setting up infrastructure, which can conflict with tight project timelines and budgets.
4. Limited DevOps and Infrastructure Capabilities
Deploying and managing microservices requires robust DevOps practices and infrastructure capabilities. If your team lacks experience in containerization, orchestration, and managing distributed systems, transitioning to a microservices architecture can become daunting. In such scenarios, focusing on building expertise in these areas or utilizing the existing strengths through a monolithic architecture might be the more practical choice.
Exemplary Code Snippet:
// Monolithic approach due to limited DevOps capabilities
public class MonolithicLimitedDevOps {
// Combined functionality for simplified deployment
public void combinedFunctionality() {
// logic
}
}
In this case, leveraging a monolithic architecture can help the team focus on strengthening their DevOps capabilities before embracing microservices.
Why:
Limited experience and expertise in DevOps and managing distributed systems can make microservices challenging to implement and maintain effectively.
Final Thoughts
In conclusion, microservices are not a one-size-fits-all solution. Understanding when to avoid microservices is crucial in ensuring the success of your project. By recognizing the common mistakes and evaluating the project requirements, teams can make informed decisions about whether to opt for a microservices architecture or stick with a monolithic approach.
While microservices offer numerous benefits, including independent scalability and easier maintenance, it's essential to consider the specific needs and constraints of each project before diving into a microservices architecture. Only by carefully evaluating these factors can teams determine the most suitable architecture for their software projects.
By being mindful of when to avoid microservices, developers can save time, reduce unnecessary complexity, and ultimately deliver more effective software solutions.
For further reading on microservices architecture, check out this comprehensive guide to microservices and learn about best practices and implementation strategies.
Remember, the goal is not just to adopt the latest trend, but to choose the architecture that best serves the needs and goals of the project at hand.