Maximizing Efficiency: Optimizing Spring's Concurrency Executors
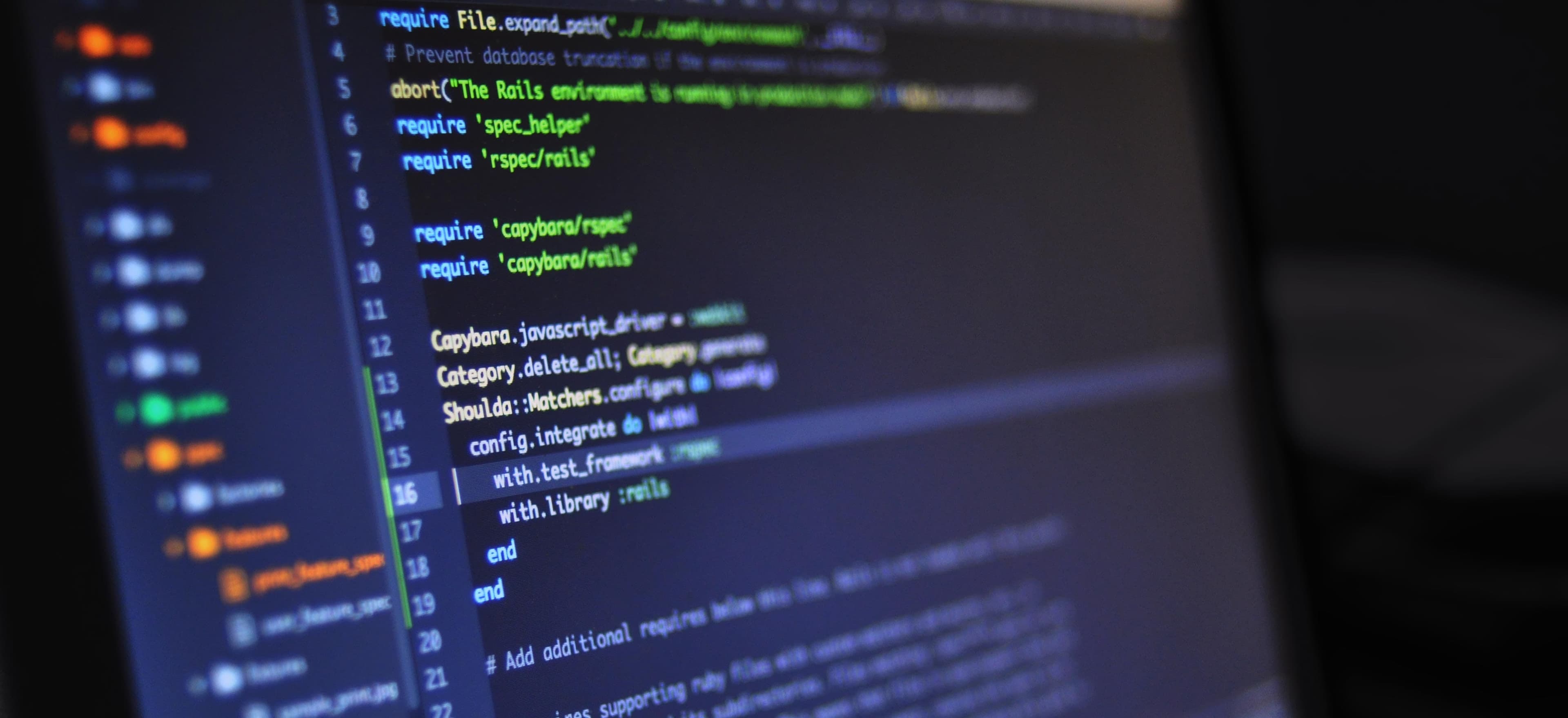
- Published on
Maximizing Efficiency: Optimizing Spring's Concurrency Executors
When working on a Java project, ensuring maximum efficiency is crucial. One way to achieve this is by optimizing the usage of Spring's concurrency executors. In this article, we'll delve into the intricacies of Spring's concurrency executors and explore techniques to maximize their efficiency.
Understanding Spring's Concurrency Executors
Spring Framework provides robust support for task execution and scheduling. It offers various executors and abstractions for concurrent programming, making it easier to manage multithreaded tasks.
One of the key components in Spring's concurrency support is the TaskExecutor
interface. This interface provides a way to decouple the execution of tasks from the main application flow, allowing for asynchronous and concurrent processing.
Leveraging ThreadPoolTaskExecutor
The ThreadPoolTaskExecutor
is a widely used implementation of the TaskExecutor
interface in Spring. It provides a configurable pool of worker threads that can asynchronously execute submitted tasks.
Configuration
Let's take a look at an example configuration of ThreadPoolTaskExecutor
in a Spring application context:
@Configuration
@EnableAsync
public class AppConfig implements AsyncConfigurer {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(5);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(25);
executor.setThreadNamePrefix("MyThread-");
executor.initialize();
return executor;
}
// Other configurations...
}
In this configuration, we define a ThreadPoolTaskExecutor
bean with a core pool size of 5, a maximum pool size of 10, a queue capacity of 25, and a thread name prefix. These parameters determine how the executor manages the execution of tasks.
Why It Matters
Configuring the ThreadPoolTaskExecutor
properly is vital for efficient task execution. The corePoolSize
and maxPoolSize
parameters, along with the queueCapacity
, directly impact the executor's behavior. By optimizing these values based on the application's requirements, we can prevent thread starvation, excessive thread creation, and queue overflow, thus enhancing overall performance.
Efficient Task Submission
While optimizing the executor itself is essential, optimizing how tasks are submitted for execution is equally important for maximizing efficiency.
Task Bundling
Instead of submitting a large number of fine-grained tasks individually, consider bundling related tasks into larger, coarse-grained tasks. This approach reduces the overhead of task submission and results in a more efficient utilization of the executor's threads.
Asynchronous Method Invocation
In Spring, methods annotated with @Async
are executed asynchronously, utilizing the configured task executor. This annotation can be applied to methods within Spring components, allowing for effortless asynchronous task execution without explicitly dealing with the executor.
@Service
public class MyService {
@Async
public void performAsyncTask() {
// Task logic
}
// Other methods...
}
Why It Matters
Optimizing task submission minimizes the overhead of task management and enhances the overall throughput of the executor. By bundling tasks and leveraging asynchronous method invocation, we can improve the efficiency of task execution and resource utilization.
Monitoring and Tuning
Efficient optimization is an ongoing process that requires monitoring and tuning based on actual performance metrics.
Monitoring with Actuator
Spring Boot Actuator provides various endpoints for monitoring and managing the application. By enabling the metrics
and health
endpoints, we can gather valuable insights into the concurrency executor's performance, thread pool metrics, and task execution statistics.
Dynamic Tuning
Based on the monitored metrics, we can dynamically adjust the executor's configuration to align with the application's runtime demands. This dynamic tuning ensures that the executor adapts to varying workloads, maximizing efficiency under different scenarios.
Final Thoughts
Optimizing Spring's concurrency executors is pivotal for achieving peak performance and resource utilization in multithreaded environments. By configuring the executors, efficiently submitting tasks, and continuously monitoring and tuning, we can ensure that our applications make the most out of concurrent processing capabilities while remaining responsive and scalable.