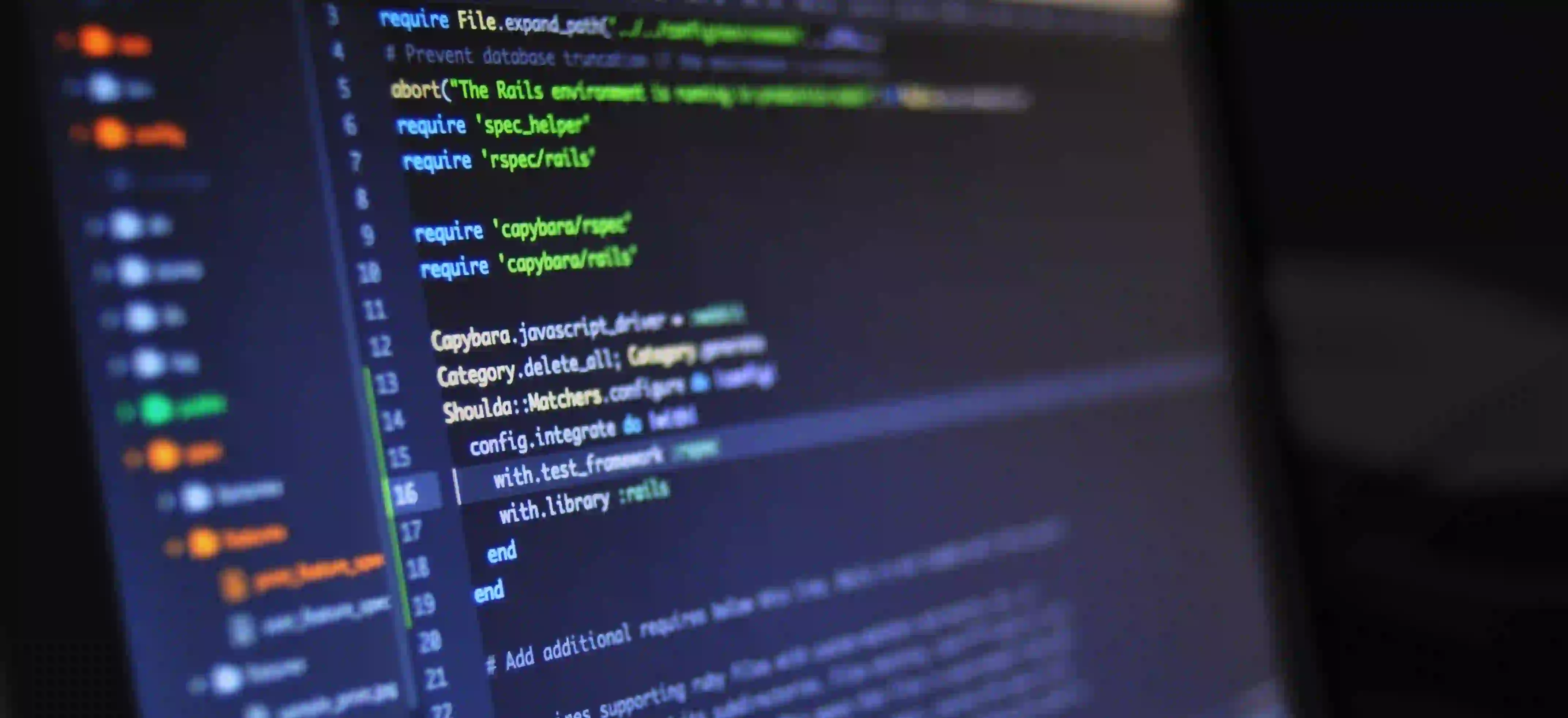
Effective Form Validation in Spring MVC
Form validation is a crucial aspect of web development as it ensures that the data entered by the user is accurate, secure, and consistent with the application's requirements. In the context of Spring MVC, form validation plays a key role in maintaining data integrity and providing a smooth user experience.
In this article, we will explore the effective implementation of form validation in Spring MVC. We will cover the use of validation annotations, custom validation logic, error handling, and the integration of validation with the front-end. Let's dive in!
1. Understanding Validation Annotations
Spring MVC provides a set of validation annotations that can be used to validate form inputs. These annotations are located in the javax.validation.constraints
package and can be applied to form fields within the model classes.
Example:
public class User {
@NotEmpty
@Size(min=2, max=30)
private String username;
@Email
private String email;
// Getters and setters
}
In this example, we have used @NotEmpty
and @Size
annotations to validate the username
field, and the @Email
annotation to validate the email
field. These annotations provide a concise and declarative way of defining validation rules.
Why: Utilizing validation annotations helps in keeping the codebase clean, readable, and maintainable. By attaching validation rules directly to the model, we ensure that the validation logic is closely tied to the data it validates.
2. Custom Validation Logic
In addition to using validation annotations, Spring MVC allows us to define custom validation logic by creating validator classes that implement the org.springframework.validation.Validator
interface.
Example:
@Component
public class UserValidator implements Validator {
@Override
public boolean supports(Class<?> clazz) {
return User.class.equals(clazz);
}
@Override
public void validate(Object target, Errors errors) {
User user = (User) target;
if (!user.getPassword().equals(user.getConfirmPassword())) {
errors.rejectValue("confirmPassword", "password.mismatch", "Passwords do not match");
}
}
}
Why: Custom validation logic is essential when the validation rules are complex and cannot be expressed solely through annotations. By creating a separate validator class, we can encapsulate the validation logic and keep the model classes focused on representing the data.
3. Integrating Validation with Controller
To integrate validation with the controller, we need to annotate the model parameter with @Valid
and the BindingResult
parameter to capture the validation errors.
Example:
@Controller
public class UserController {
@Autowired
private UserValidator userValidator;
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.addValidators(userValidator);
}
@PostMapping("/users")
public String addUser(@Valid @ModelAttribute("user") User user, BindingResult result) {
if (result.hasErrors()) {
return "registration-form";
}
// Process the valid user data
return "redirect:/success";
}
}
Why: By using @Valid
and BindingResult
, we can seamlessly integrate the validation process into the controller method. The BindingResult
object contains information about any validation errors that may have occurred during the processing of the form.
4. Displaying Validation Errors in the View
When validation errors occur, it is important to display meaningful error messages to the user. This can be achieved by adding the Errors
object to the model and handling it in the view layer.
Example (Thymeleaf Template):
<form th:object="${user}" th:action="@{/users}" method="post">
<input type="text" th:field="*{username}" />
<span th:if="${#fields.hasErrors('username')}" th:errors="*{username}"></span>
<!-- Other form fields -->
</form>
Why: Providing clear and contextual error messages enhances the user experience and helps users rectify their inputs effectively. By integrating error handling in the view layer, we maintain a cohesive user interface that accounts for validation feedback.
5. Handling Global Validation Errors
In some cases, we may also need to handle global validation errors that are not specific to a particular form field. This can be achieved by using the @ControllerAdvice
annotation and creating a global error handler.
Example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(MethodArgumentNotValidException.class)
@ResponseStatus(HttpStatus.BAD_REQUEST)
@ResponseBody
public Map<String, String> handleValidationExceptions(MethodArgumentNotValidException ex) {
Map<String, String> errors = new HashMap<>();
ex.getBindingResult().getFieldErrors().forEach(error ->
errors.put(error.getField(), error.getDefaultMessage()));
return errors;
}
}
Why: Handling global validation errors is crucial for ensuring a unified approach to error handling across the application. By using @ControllerAdvice
, we can centralize the management of validation errors and provide consistent error responses.
The Bottom Line
In this article, we have explored the effective implementation of form validation in Spring MVC. By leveraging validation annotations, custom validation logic, error handling, and integration with the front-end, we can ensure robust and user-friendly form validation.
Form validation is an integral part of building reliable web applications, and Spring MVC provides a comprehensive set of tools and techniques to address this requirement effectively.
By mastering form validation in Spring MVC, developers can enhance the integrity and usability of their applications, thereby delivering a seamless experience to their users.
Implementing robust form validation in Spring MVC not only ensures data integrity but also enhances user experience, boosting the overall quality of the application.