Updating Java 7 Substring Behavior
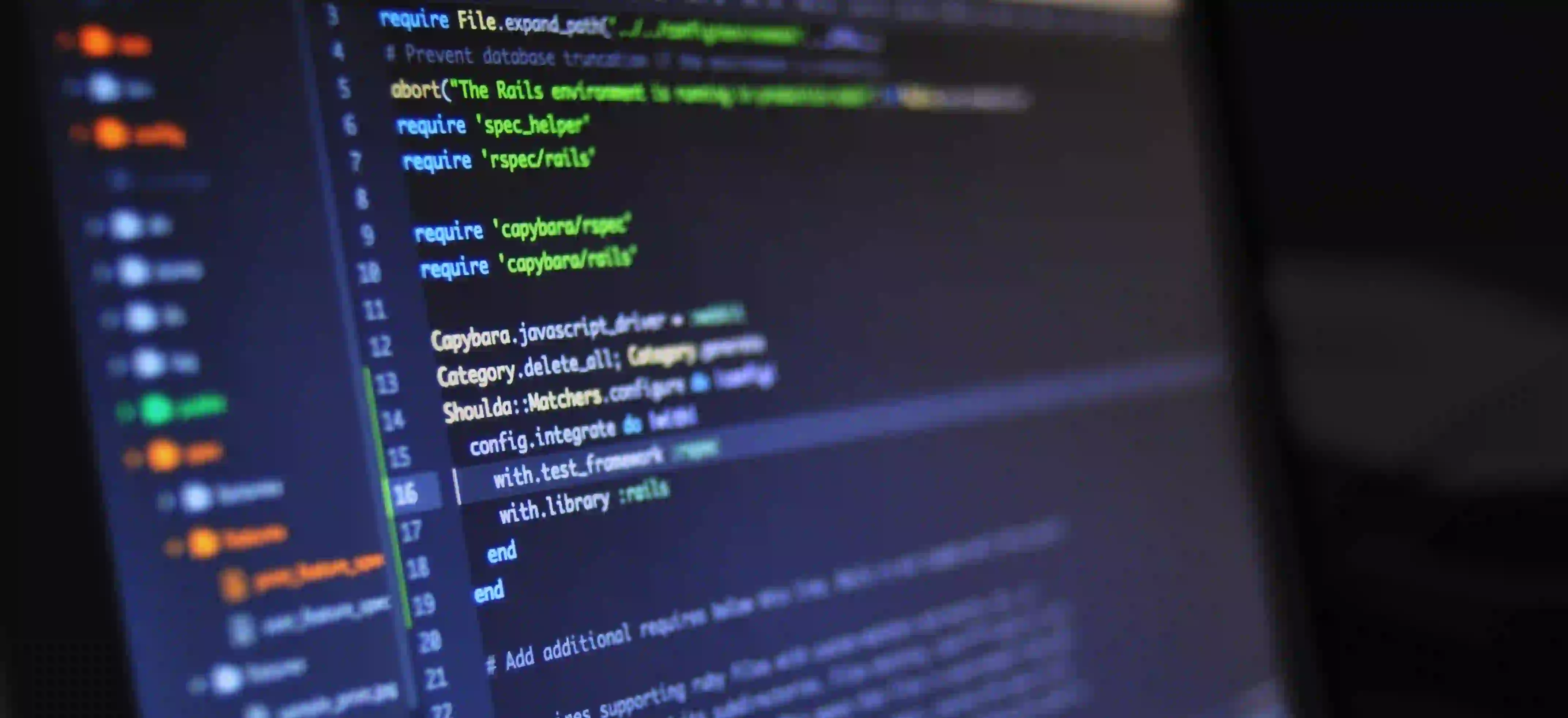
Understanding Java 7 Substring Behavior Updates
In Java 7, the String
class's substring
method had its internal implementation slightly modified, which resulted in a performance impact for certain use cases. This blog post delves into the changes made to the substring
method, the rationale behind them, and implications for developers using Java 7.
The Previous Behavior
Before Java 7, the substring
method in the String
class returned a new string comprised of the characters from the original string's specified range. This entailed creating a new character array and copying the relevant characters into it.
The Update in Java 7
With the update in Java 7, the substring
method no longer creates a new character array. Instead, it retains a reference to the original character array and the offset and count values that mark the subrange of interest.
Rationale Behind the Update
The modification aimed at improving performance and reducing memory overhead, especially when dealing with large strings. By eliminating the need to create a new character array for each substring
call, the update aimed to enhance the efficiency of string manipulations.
Implications for Developers
While the update intended to provide performance benefits, it inadvertently introduced a scenario where substrings held references to the entire original character array, preventing it from being garbage collected. Consequently, retaining a reference to a relatively small substring could unintentionally hold a large character array in memory, impacting memory usage and potentially leading to memory leaks.
Code Example
String longString = "This is a long string containing various characters.";
String substring = longString.substring(8, 15);
In the above code, the substring
retains a reference to the original character array, encompassing the entire longString
. This behavior may lead to unexpected memory retention and should be considered when dealing with large strings.
Mitigating the Issue
To mitigate the unintended memory overhead and potential memory leaks introduced by the updated substring
behavior, developers needed to explicitly create a new string from a substring when the original large string was no longer required.
Code Example
String newSubstring = new String(longString.substring(8, 15));
By creating a new string from the substring, developers ensured that only the necessary characters from the original string were retained, reducing memory usage and preventing unintentional memory retention.
Closing Remarks
The update to the substring
method in Java 7 aimed to enhance performance and decrease memory overhead but also introduced unintended consequences related to memory management. Careful consideration and explicit handling of substring creation were necessary to prevent potential memory leaks and excessive memory usage with large strings.
Understanding these nuances is crucial for Java developers when dealing with string manipulations in Java 7 and serves as a reminder to consider the broader implications of seemingly minor updates to foundational classes.
To sum up, while the Java 7 substring
behavior update aimed to optimize performance, it inadvertently introduced memory management complexities that developers needed to be aware of and proactively address in their code.
For more detailed insights and updates on Java language features, you can explore the official Java documentation and the Java SE platform.
Stay tuned for more Java-related discussions and insights!