Optimizing Java Micro Benchmarks
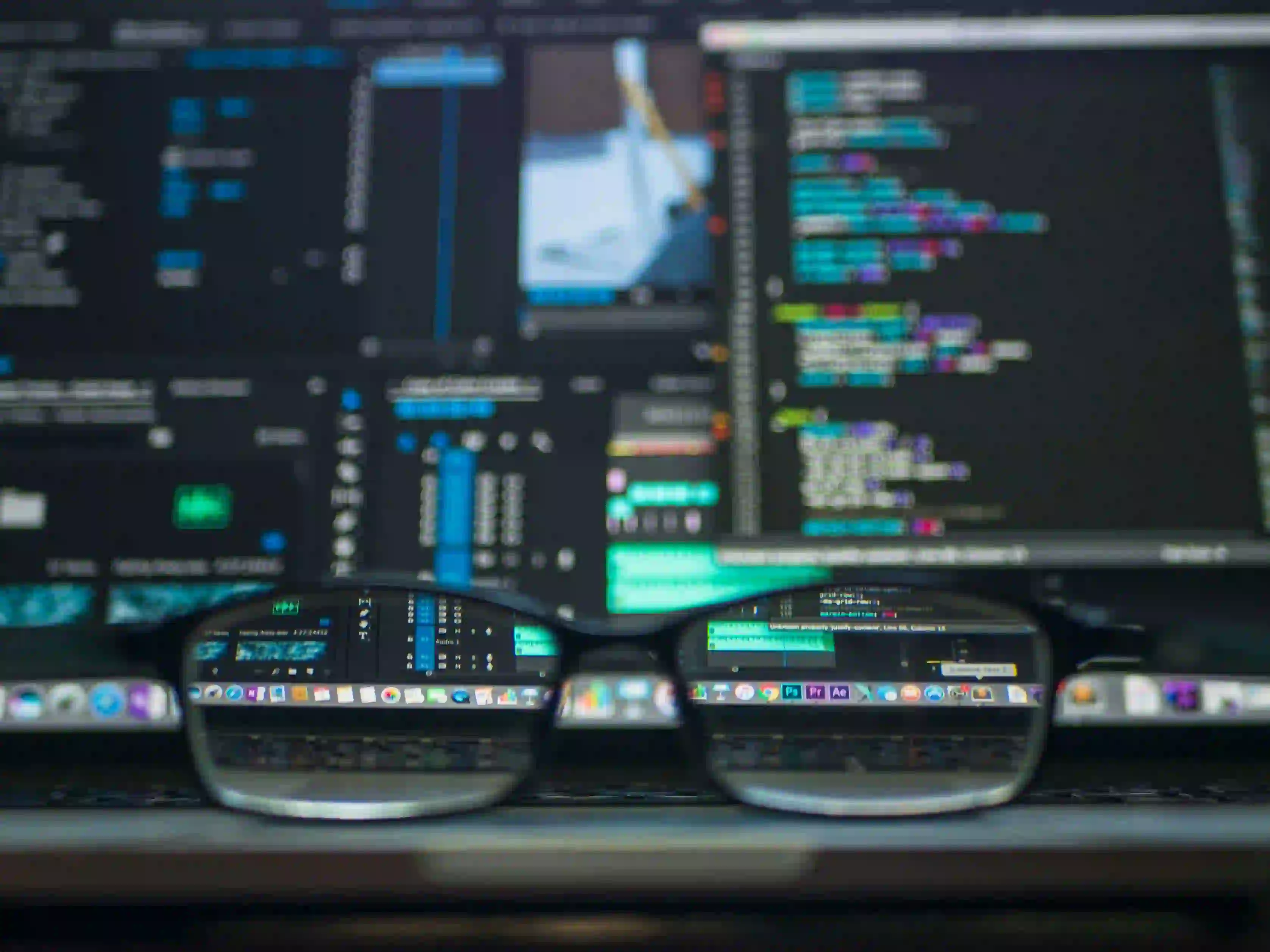
Understanding the Importance of Micro Benchmarks in Java
In Java programming, performance is a critical aspect that can greatly impact the efficiency and effectiveness of an application. micro benchmarks serve as essential tools for measuring the performance of small code snippets or methods within a program. Sufficiently optimized micro benchmarks can aid in identifying potential performance bottlenecks, allowing developers to make informed decisions about code optimization and improvement.
Setting up JMH for Micro Benchmarking
Java Microbenchmarking Harness (JMH) is a widely used tool for micro benchmarking in the Java ecosystem. It provides an easy and reliable way to benchmark Java code, taking into account various factors such as warmup iterations, measurement iterations, and benchmark mode selection.
To integrate JMH into a Java project, the following dependencies need to be included in the project's build configuration:
<dependency>
<groupId>org.openjdk.jmh</groupId>
<artifactId>jmh-core</artifactId>
<version>1.32</version>
</dependency>
<dependency>
<groupId>org.openjdk.jmh</groupId>
<artifactId>jmh-generator-annprocess</artifactId>
<version>1.32</version>
<scope>provided</scope>
</dependency>
Writing Micro Benchmarks Using JMH
Creating a micro benchmark with JMH involves defining a benchmark class and annotating the methods to be benchmarked. Let's consider an example where we want to compare the performance of two different approaches to concatenate strings.
@State(Scope.Benchmark)
@BenchmarkMode(Mode.Throughput)
@Fork(value = 1)
@Warmup(iterations = 3)
@Measurement(iterations = 5)
public class StringConcatenationBenchmark {
private String firstString = "Hello";
private String secondString = "World";
@Benchmark
public String testStringConcatenationOperator() {
return firstString + secondString;
}
@Benchmark
public String testStringBuilderAppend() {
StringBuilder sb = new StringBuilder();
sb.append(firstString);
sb.append(secondString);
return sb.toString();
}
}
In this example, we've annotated the class with various JMH annotations. The @State
annotation indicates that the benchmark state should be shared across all the threads, and the @BenchmarkMode
specifies that the benchmark should measure throughput. The @Fork
, @Warmup
, and @Measurement
annotations control the benchmark execution and iteration settings.
Running and Analyzing Micro Benchmarks
After defining the micro benchmark, we can run it using JMH's command-line runner, Maven plugin, or programmatically within a Java program. The results will include metrics such as throughput, average time, and various statistical values, providing insights into the performance of the benchmarked methods.
Analyzing the results of micro benchmarks is crucial for making data-driven optimizations. By identifying the most time-consuming operations and bottlenecks, developers can focus their efforts on the areas that will yield the greatest performance improvements.
Best Practices for Optimizing Java Micro Benchmarks
While conducting micro benchmarks, it's essential to adhere to best practices to ensure the accuracy and reliability of the benchmark results.
1. Warmup Iterations
Warmup iterations are essential for allowing the Just-In-Time (JIT) compiler to optimize the code before the actual measurement takes place. Without proper warmup, the benchmark results might be skewed due to the initial overhead of JIT compilation.
2. Benchmark Mode Selection
Choosing the appropriate benchmark mode based on the nature of the code being benchmarked is crucial. For instance, the Mode.Throughput
mode is suitable for measuring the throughput of a method, while the Mode.AverageTime
mode is more appropriate for determining the average time per operation.
3. Avoiding Dead Code Elimination
The JIT compiler might optimize away seemingly redundant operations in the benchmarked code. To prevent this, using the Blackhole
class provided by JMH to consume the results of the benchmarked methods can help in ensuring that the measured code is not eliminated.
4. Properly Managing State
Careful management of benchmark state is essential to avoid any unintended side effects during the benchmarking process. Utilizing JMH's state management annotations such as @State
and @Setup
can help in maintaining the required state for accurate benchmarking.
5. Understanding JIT Compilation Impact
It's crucial to understand the influence of JIT compilation on the benchmark results. Factors such as method inlining, loop unrolling, and other optimization techniques performed by the JIT compiler can significantly impact the measured performance.
Final Thoughts
Micro benchmarking plays a pivotal role in identifying performance bottlenecks and fine-tuning the efficiency of Java code. By utilizing Java Microbenchmarking Harness (JMH) and following best practices for micro benchmarking, developers can gain valuable insights into the performance characteristics of their code, enabling them to make informed optimization decisions.
With the ability to accurately measure the performance of specific code segments, developers can focus their optimization efforts where they will have the greatest impact, ultimately leading to more efficient and responsive Java applications.
In conclusion, micro benchmarks are indispensable tools for achieving optimal performance in Java applications, and leveraging JMH along with best practices can significantly contribute to the overall performance enhancement efforts.
To learn more about JMH and advanced micro benchmarking techniques, the official OpenJDK JMH documentation provides comprehensive insights and guidance for proficient micro benchmarking in Java.