Mastering Java Through Tennis Game Katas
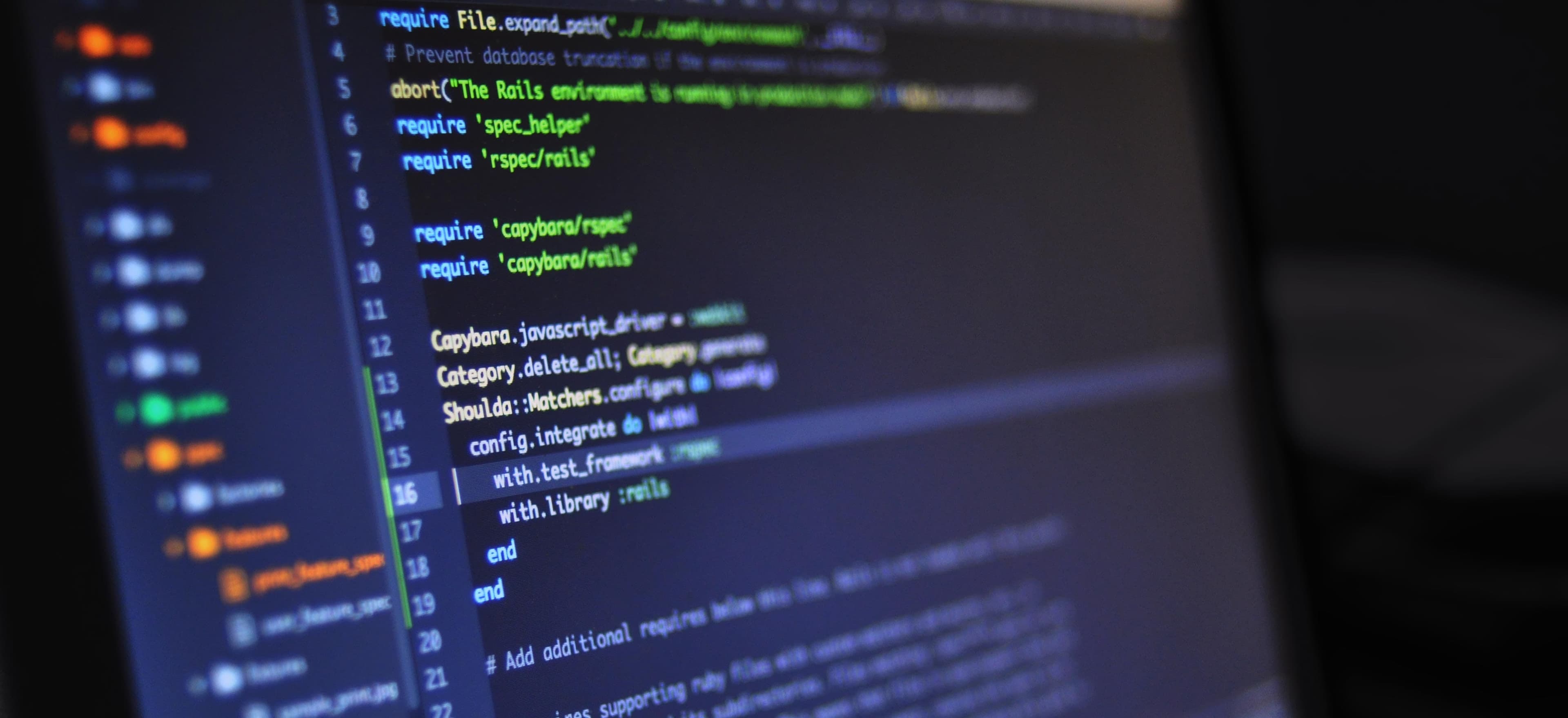
- Published on
Mastering Java Through Tennis Game Katas
If you're looking to enhance your Java programming skills while having fun, tennis game katas are an excellent way to do just that. Katas are small coding exercises that help improve your coding abilities through deliberate practice and repetition. In this blog post, we'll explore how to use tennis game katas to master Java programming.
The Tennis Game Kata
The Tennis Game Kata is a popular exercise that challenges developers to create a simple tennis scoring system. The rules are straightforward: two players compete in a tennis game, and the scoring follows the traditional tennis scoring system (Love, 15, 30, 40). The kata requires you to implement the logic for scoring and determining the winner based on the game's progress.
Setting Up Your Java Environment
Before diving into the kata, ensure that you have Java installed on your machine. You can download the latest version of Java Development Kit (JDK) from the official Java website.
Once you have Java installed, set up your preferred IDE (Integrated Development Environment) such as IntelliJ IDEA, Eclipse, or NetBeans. These IDEs provide a comfortable environment for writing, testing, and debugging Java code.
Starting the Kata
To begin the Tennis Game Kata, create a new Java project in your IDE. Within the project, start by setting up the necessary classes and tests. The Test-Driven Development (TDD) approach works well for this kata, as it encourages writing tests before implementing the actual logic.
Example Test Case
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class TennisGameTest {
@Test
public void testInitialScore() {
TennisGame tennisGame = new TennisGame();
assertEquals("Love - Love", tennisGame.getScore());
}
}
In the example above, a simple test case verifies the initial score of the tennis game. The getScore()
method is expected to return "Love - Love" at the start of the game.
Implementing the TennisGame Class
public class TennisGame {
public String getScore() {
return "Love - Love";
}
}
The initial implementation of the TennisGame
class returns a hardcoded score. As you progress through the kata, you will incrementally update the getScore()
method's logic to handle various game scenarios.
Refactoring and Improving Your Code
As you implement the Tennis Game Kata, take the opportunity to refactor and improve your code. Consider using design patterns such as the Strategy pattern to handle different scoring cases effectively. Keep your code modular and maintainable by creating separate classes or methods for specific functionalities like scoring rules, player management, and game state.
Testing Your Code
Testing is a crucial aspect of the development process. Write comprehensive unit tests to cover different game scenarios and edge cases. Consider using mocking frameworks like Mockito to simulate player actions and game states for more complex test cases.
Example Test Case for Scoring
@Test
public void testPlayer1WinsGame() {
TennisGame tennisGame = new TennisGame();
tennisGame.playerScores(1);
tennisGame.playerScores(1);
tennisGame.playerScores(1);
tennisGame.playerScores(1);
assertEquals("Player 1 wins the game", tennisGame.getScore());
}
In this test case, player 1 scores four points, winning the game. The assertEquals()
method verifies that the game score reflects the expected outcome.
Embracing Object-Oriented Principles
As you work on the kata, strive to apply object-oriented principles in your Java code. Encapsulate behaviors within classes, leverage inheritance and polymorphism where applicable, and ensure that your code is cohesive and loosely coupled. This systematic approach will not only improve your implementation of the Tennis Game Kata but also strengthen your grasp of object-oriented programming in Java.
Final Thoughts
Mastering Java through tennis game katas provides a practical and engaging way to elevate your programming skills. By tackling the kata incrementally, refactoring your code, writing comprehensive tests, and embracing object-oriented principles, you'll solidify your understanding of Java while enjoying the process.
By integrating tennis game katas into your Java programming journey, you'll cultivate a deeper understanding of the language and its applications. Stay engaged, experiment with different approaches, and continuously seek opportunities to enhance your skills. Happy coding!
Remember, the journey to mastering Java is as exhilarating and rewarding as a thrilling tennis match. So, grab your racket (or in this case, your IDE) and start swinging (coding)!