Overcoming Tool Dependence
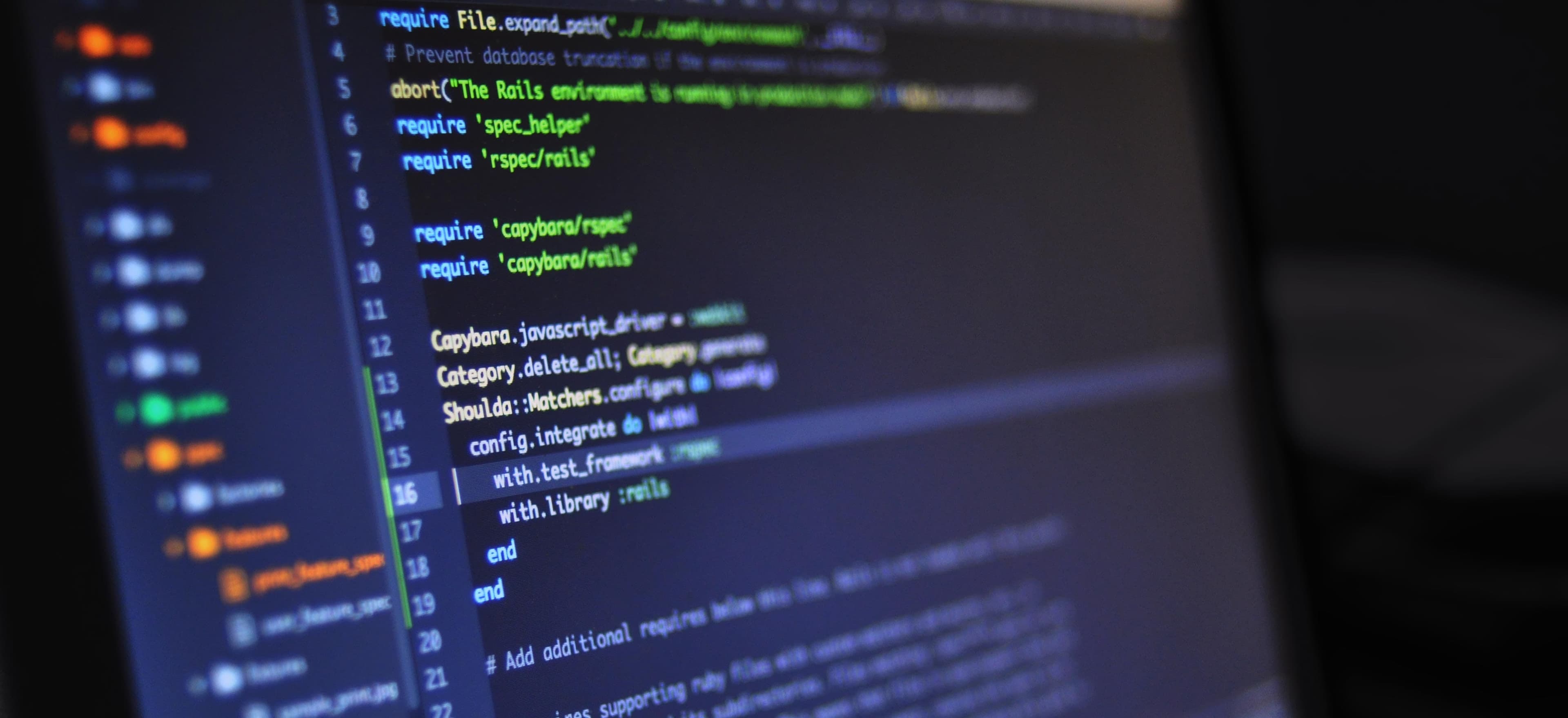
- Published on
In the world of Java development, it's common to rely heavily on various tools and libraries to streamline the coding process. While these tools undoubtedly offer efficiency and ease, developers can often become overly dependent on them, leading to a lack of understanding of the underlying principles. In this blog post, we'll discuss the ramifications of tool dependence in Java development and explore strategies to overcome it.
The Pitfalls of Tool Dependence
Loss of Core Skill Development
Java developers often turn to frameworks, libraries, and IDEs to simplify their workflow. While these tools undeniably enhance productivity, they can also lead to a stagnation of core Java skills. Relying solely on auto-generated code or pre-built functions can hinder the developer's ability to write clean, efficient code from scratch.
Hidden Complexity
Many Java tools abstract away the underlying complexity, providing convenient shortcuts for common tasks. While this can be advantageous in the short term, it may obscure the intricacies of the language and platform, leading to a lack of understanding of what's happening beneath the surface.
Limited Problem-Solving Abilities
Tool-dependent developers may struggle when faced with unique or unconventional challenges that fall outside the scope of their familiar tools. Over-reliance on specific frameworks or libraries can hinder the ability to think creatively and solve problems independently.
Strategies to Overcome Tool Dependence
Emphasize Fundamental Concepts
To combat tool dependence, it's essential to place a renewed emphasis on mastering fundamental Java concepts. Understanding core principles such as object-oriented programming, data structures, and algorithms can empower developers to write robust, efficient code without being overly reliant on external tools.
Regular Code Reviews and Pair Programming
Engaging in regular code reviews and pair programming sessions can provide valuable opportunities to collaborate and learn from peers. By actively discussing and dissecting code, developers can gain deeper insights into Java best practices and design patterns, reducing the temptation to rely solely on automated tools.
Build Projects From Scratch
Taking on projects that require building functionality from the ground up can be immensely beneficial in overcoming tool dependence. By starting with a clean slate, developers are compelled to write more code manually, reinforcing their understanding of Java fundamentals and reducing reliance on automated generators.
Leverage Documentation and Tutorials
When utilizing tools and libraries, it's crucial to refer to their official documentation and delve into tutorials that explore their internal workings. Understanding the rationale behind the design and implementation of these tools can enhance knowledge and mitigate the risk of over-reliance.
Continuous Learning and Exploration
Encouraging a culture of continuous learning and exploration within the development team can foster a proactive approach to mastering Java. This includes staying abreast of language updates, exploring new features, and delving into advanced topics such as concurrency, performance optimization, and security.
Balancing Efficiency with Understanding
While it's important to acknowledge the potential drawbacks of tool dependence, it's equally crucial to recognize the value that these tools bring to Java development. Frameworks like Spring and libraries such as Apache Commons offer immense productivity benefits and have become integral parts of the Java ecosystem. The objective is not to eliminate tool usage but to strike a balance between efficiency and a deep understanding of the underlying language and platform.
Exemplifying Balancing Act: Dependency Injection
Consider the prevalent practice of dependency injection in Java, commonly facilitated by frameworks like Spring. While it's tempting to solely rely on Spring's injection mechanisms, understanding the principles of inversion of control and dependency inversion can enable developers to implement manual dependency injection when the situation demands it, providing a clear example of striking the balance.
// Manual Dependency Injection
public class MyApp {
private DataService dataService;
public MyApp(DataService dataService) {
this.dataService = dataService;
}
public void performAction() {
// Use dataService here
}
}
In the above example, the MyApp
class performs manual dependency injection by accepting a DataService
instance through its constructor, bypassing the reliance on a framework for this particular aspect, thereby exemplifying a balanced approach.
Closing Remarks
Overcoming tool dependence in Java development is a multifaceted endeavor that requires a conscious effort to strike a balance between leveraging modern tools and honing core Java skills. By emphasizing fundamental concepts, engaging in collaborative learning, and exploring new avenues of knowledge, developers can transcend tool dependence and cultivate a deeper understanding of the language. Ultimately, this balanced approach equips developers with the versatility and expertise to navigate the dynamic landscape of Java development with confidence and proficiency. Embracing the fundamentals while leveraging tools harmoniously is the key to a robust and sustainable Java development career.