Optimizing Database Performance for Microservices
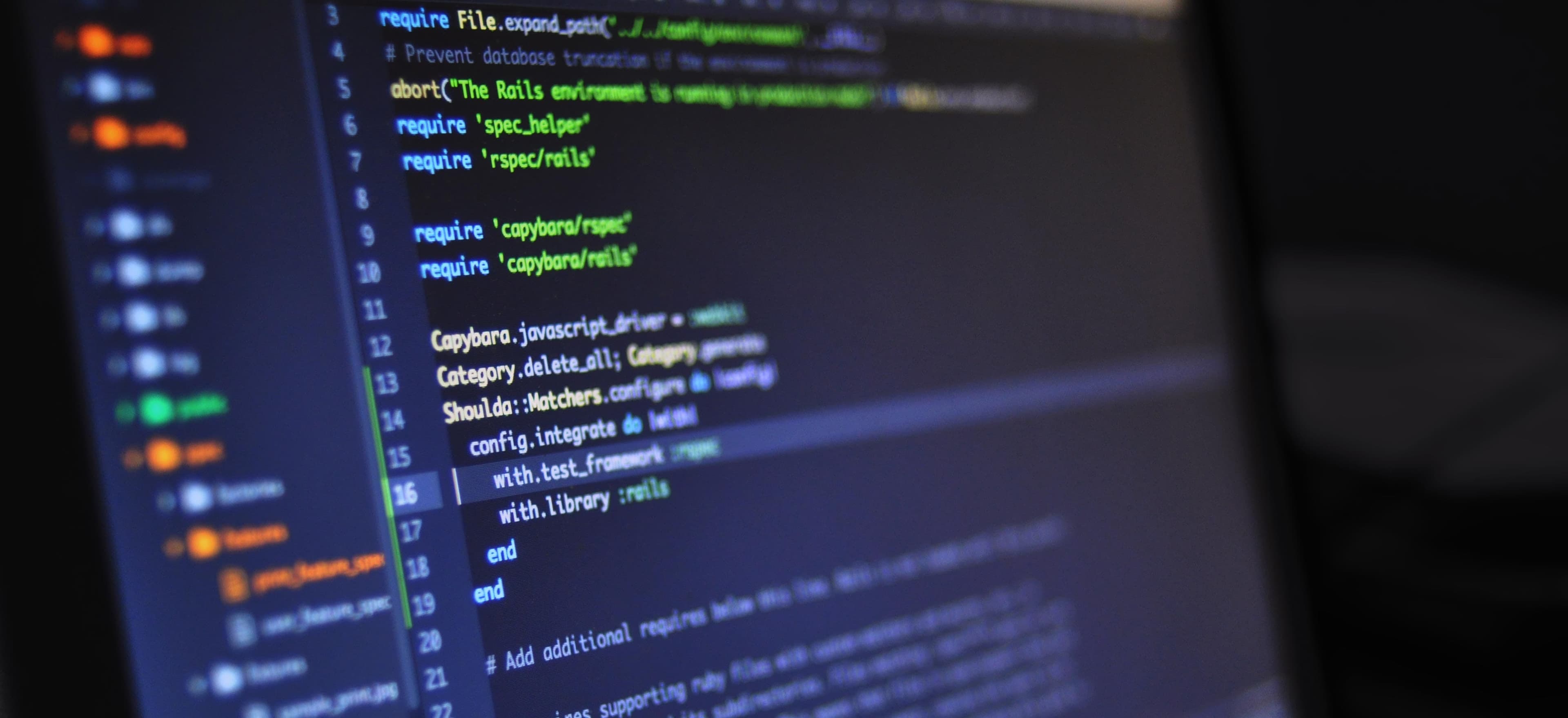
- Published on
Optimizing Database Performance for Microservices
Microservices have become increasingly popular as a way to build and scale applications. They allow developers to create small, independent services that work together to form a larger application. One of the key challenges when working with microservices is optimizing the performance of the underlying database systems. In this article, we will explore some best practices and strategies for optimizing database performance in the context of microservices.
Understanding the Challenges
When dealing with microservices, each service usually has its own database, which introduces some challenges in terms of performance optimization. The key challenges include:
-
Data Consistency: With multiple services reading and writing to different databases, ensuring data consistency becomes a challenge.
-
Data Access Patterns: Each microservice might have different data access patterns, which can lead to inefficient database queries and slow performance.
-
Scalability: As the number of microservices grows, the overall database workload increases, requiring scalable solutions.
Best Practices for Optimizing Database Performance
1. Choose the Right Database
Selecting the appropriate database for your microservices architecture is crucial. Different databases have different strengths and weaknesses, and choosing the right one can significantly impact performance. Consider factors such as data model, query requirements, scalability, and consistency when making this decision.
2. Database Sharding
Sharding involves splitting a large database into smaller, more manageable parts called shards. Each shard has its own data and can be hosted on separate servers. This can help distribute the database load and improve performance.
// Example of database sharding using Spring Data JPA
@Entity
@Table(name = "user")
@Immutable
@Subselect("select * from user where shard_key = 'shard1'")
public class Shard1User extends User {
// Entity definition
}
3. Use Caching
Implementing a caching strategy can greatly improve database performance by reducing the number of database calls. Use tools like Redis or Memcached to cache frequently accessed data.
// Example of using Redis for caching in Spring Boot
@Autowired
private RedisTemplate<String, User> redisTemplate;
public User getUser(String userId) {
User user = redisTemplate.opsForValue().get(userId);
if (user == null) {
user = userRepository.findById(userId);
redisTemplate.opsForValue().set(userId, user);
}
return user;
}
4. Asynchronous Processing
Consider using asynchronous processing for tasks that do not require immediate responses. Tools like Kafka or RabbitMQ can be used to offload non-critical database tasks and improve overall system performance.
// Example of asynchronous processing with Kafka in Spring Boot
@KafkaListener(topics = "database-tasks")
public void processDatabaseTask(String task) {
// Process the task asynchronously
}
5. Monitor and Tune Query Performance
Regularly monitor database queries and their performance. Use tools like Spring Boot Actuator and Micrometer to gather metrics and identify slow queries. Tune the queries by adding indexes, optimizing SQL, or denormalizing data where necessary.
// Example of adding an index in Spring Data JPA
@Entity
@Table(name = "user")
public class User {
// Other fields and annotations
@Index(name = "idx_username")
private String username;
// Getters and setters
}
6. Implement Circuit Breaker Pattern
To prevent cascading failures and improve resilience, implement the Circuit Breaker pattern. Tools like Resilience4j or Hystrix can be used to handle and recover from database failures gracefully.
// Example of using Resilience4j for circuit breaking in Spring Boot
@CircuitBreaker(name = "databaseService", fallbackMethod = "fallbackMethod")
public Data fetchData(String key) {
// Database call
}
public Data fallbackMethod(String key, Exception e) {
// Fallback logic
}
Final Considerations
Optimizing database performance in a microservices architecture is crucial for maintaining the overall system efficiency and scalability. By following the best practices outlined in this article, you can ensure that your microservices operate with optimal database performance, ultimately providing a better experience for your users.
By understanding the unique challenges presented by microservices and employing the right strategies and tools, you can build a robust and high-performing database layer for your microservices architecture.
Remember, database performance optimization is an ongoing process, and it requires continuous monitoring, testing, and refinement to adapt to changing requirements and usage patterns.
For further reading, check out these resources:
- Scaling Microservices with Message Queues
- Best Practices for Microservices Architecture
- Monitoring Microservices with Spring Boot Actuator
- Resilience4j Circuit Breaker
Thank you for reading! Let's continue to build high-performance microservices together.