Why Verification Fails: Common Pitfalls in Testing
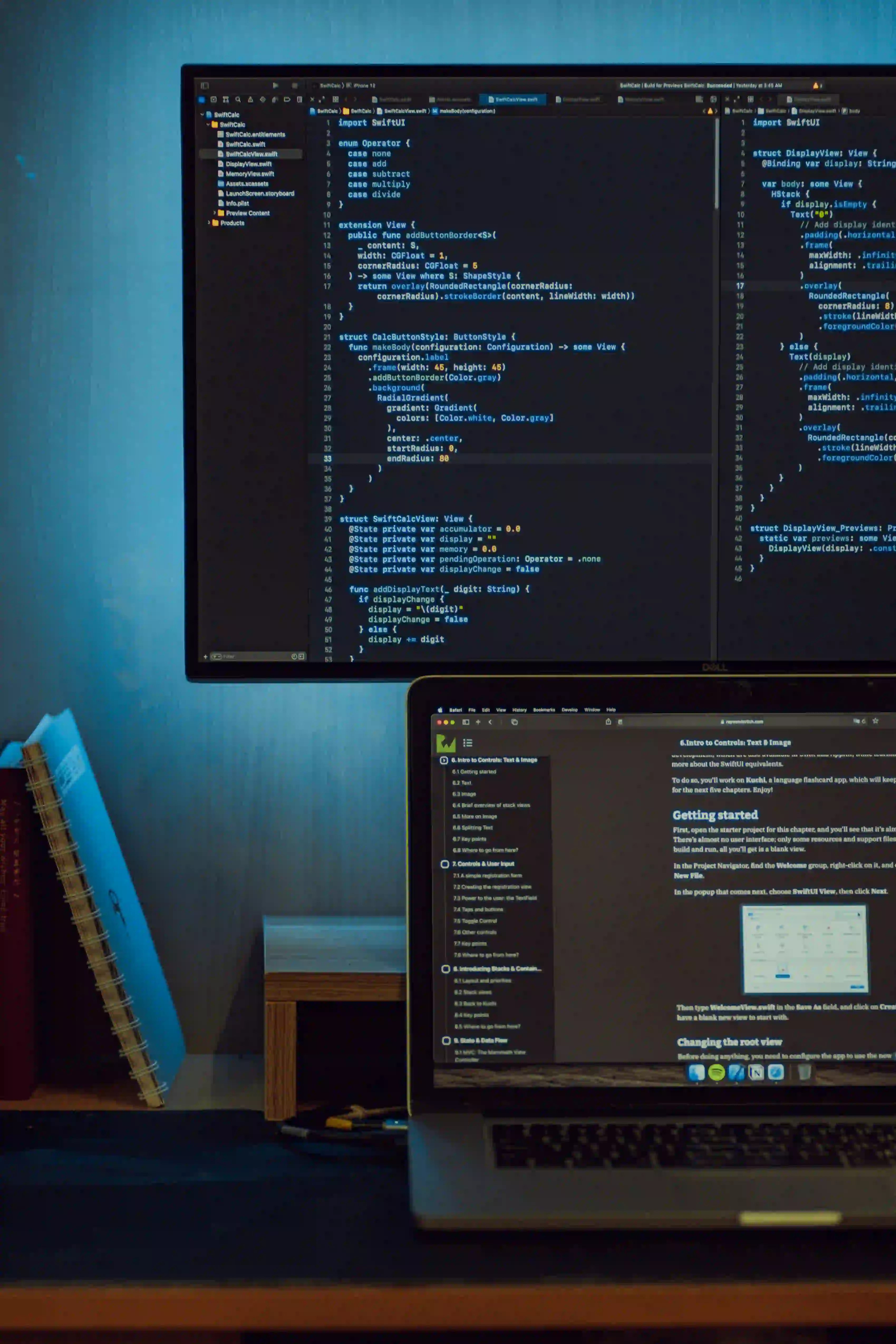
Why Verification Fails: Common Pitfalls in Testing
Software testing is a critical stage within the software development lifecycle. It ensures that the software product is functional, reliable, and of high quality before reaching the user. However, despite the best intentions and rigorous methodologies, verification often fails. Understanding the common pitfalls in testing allows developers to avoid these mistakes, resulting in more robust software.
In this blog post, we’ll discuss the reasons why verification fails, the importance of proper testing strategies, and practical ways to enhance your testing processes.
Understanding Software Verification and Validation
Before discussing where verification falls short, let's clarify what verification and validation mean in software testing.
- Verification: Ensures the product is built correctly according to specifications. It answers the question, "Did we build the product right?"
- Validation: Confirms the product meets user requirements and expectations. It answers, "Did we build the right product?"
Both processes are crucial, but verification often encounters specific challenges that can lead to failures.
Common Pitfalls in Verification
1. Inadequate Test Coverage
One of the most critical pitfalls is having insufficient test coverage. If your tests do not cover all code paths, you risk missing bugs. This oversight can lead to verifying only parts of the application.
Solution:
- Use Code Coverage Tools: Tools like JaCoCo or Cobertura can help analyze test coverage.
Example of a simple test using JaCoCo:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In this example, if you notice that the add
method tests only a favorable scenario, you still need to test edge cases like adding negative numbers.
2. Lack of Clear Requirements
When requirements are muddled or ambiguous, verifying against them becomes an impossible task. If the team has unclear understanding of what they are testing, the likelihood of falling short increases.
Solution:
- Collaborative Requirement Gathering: Engage stakeholders in the requirement definition process. This creates a shared understanding and forms a clear benchmark for verification.
You can employ user stories in Agile methodologies to achieve clear and concise requirements. For example:
As a user, I want to log in using my email and password, so that I can access my account securely.
This specifies precisely what the functionality should accomplish, aiding in verification.
3. Failure to Automate Tests
In an era where continuous integration and delivery (CI/CD) are the norms, relying on manual testing can lead to human error and sluggish testing cycles. Manual testing can also miss out on corner cases, leading to failures in verification.
Solution:
- Implement Test Automation: Leverage frameworks such as JUnit for unit tests or Selenium for UI testing to automate verification processes.
Here’s a quick example using Selenium to check if a login page displays correctly:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class LoginPageTest {
private WebDriver driver;
@Before
public void setup() {
driver = new ChromeDriver();
driver.get("http://example.com/login");
}
@Test
public void testLoginPageTitle() {
String title = driver.getTitle();
assertEquals("Login Page", title);
}
@After
public void teardown() {
driver.quit();
}
}
Automating the test not only reduces human error but also provides quick feedback on verification.
4. Underestimating Testing Time
Many teams underestimate the time required for thorough testing. Rushing through this phase can result in incomplete testing and poorly verified software.
Solution:
- Allocate Adequate Time for Testing: Include realistic estimates for testing within project timelines. Engage in discussions about testing during sprint planning and retrospectives.
For instance, in Agile development, consider separating testing sprints or using pairs of developers and testers working collaboratively.
5. Ignoring Regression Testing
Regression testing ensures that new changes do not adversely affect existing features. Ignoring this process can lead to previously verified functionalities breaking down in future releases.
Solution:
- Create a Regression Test Suite: After every major feature addition, conduct regression tests systematically.
Here’s a code snippet that employs JUnit for regression tests:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class FeatureRegressionTest {
@Test
public void testPreviousFunctionality() {
// Assume original functionality is to return 5 on the method call
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
// New feature testing
assertEquals(4, calculator.subtract(7, 3));
}
}
This verifies that updates do not disrupt established functionality.
6. Lack of Test Data Management
For an effective testing process, it is essential to have a well-defined and managed test data set. If the team executes tests without proper data, verification can yield misleading results.
Solution:
- Utilize Test Data Management Tools: Tools such as Test Data Management (TDM) will secure reliable data for testing purposes.
By maintaining a repository of data states, testers can efficiently execute different scenarios.
7. Poor Communication Among Team Members
Good communication between developers, testers, and stakeholders is vital for effective verification. Misunderstandings can lead to incorrect assumptions and flawed tests.
Solution:
- Encourage Cross-Functional Team Collaboration: Hold regular meetings and adopt collaboration tools such as JIRA or Confluence to enhance transparency.
Having a collaborative culture facilitates shared understanding and clearer verification.
Lessons Learned
Verification is a fundamental part of software testing that can suffer from several pitfalls. Awareness of these common mistakes and proactively addressing them can significantly improve the effectiveness of your testing process. From employing automation to ensuring team collaboration, organizations can better their verification practices.
Understanding your verification processes can lead to producing quality software, minimizing bugs, and achieving user satisfaction.
For more extensive reading, refer to ISTQB - International Software Testing Qualifications Board.
By being vigilant and proactive in your testing strategy, you can navigate the verification minefield, ensuring your software meets both specification and user expectations flawlessly. Happy testing!