Understanding Java Annotations: Why Retention Matters
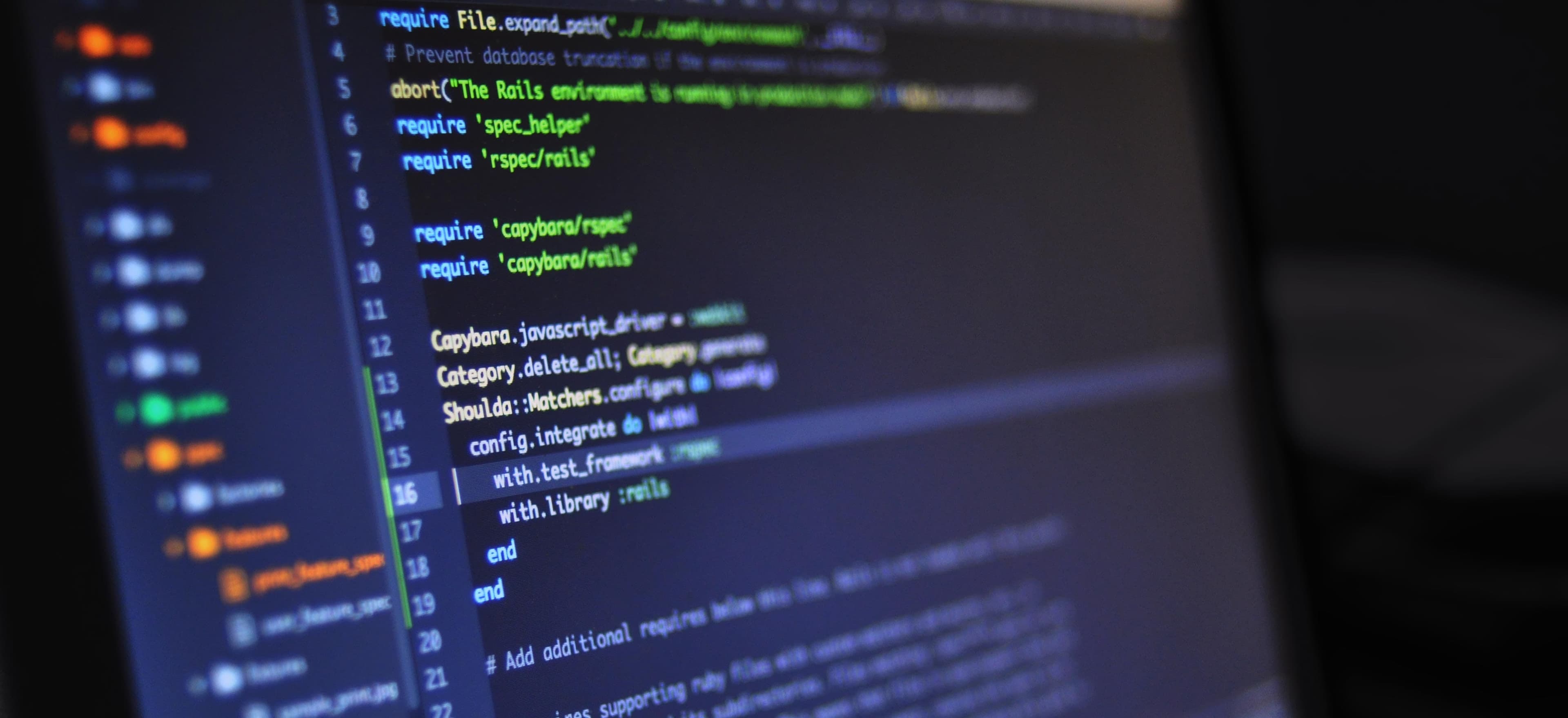
- Published on
Understanding Java Annotations: Why Retention Matters
Java annotations are a powerful feature that allows developers to include metadata about their code. They can offer important information regarding programming elements, from classes to methods and fields. In this post, we will explore Java annotations, their significance, and particularly focus on the concept of retention policy. By the end of this post, you will have a clearer understanding of how annotations work and why retention is crucial for their functionality.
What are Java Annotations?
Java annotations are a form of metadata. They provide data about a program but are not part of the program itself. The Java programming language (since version 5) introduced annotations to allow developers to add information to their code. Popular use cases of annotations include:
- Code documentation: Annotations can enhance readability by providing details about the intended use.
- Framework-driven development: Frameworks like Spring and Hibernate use annotations extensively for configuration purposes.
- Compile-time checks: Annotations can trigger errors at compile-time through tools like the Java Annotation Processing Tool (APT).
Basic Structure of Annotations
Annotations are defined using the @interface
keyword in Java. Here's a simple example of defining an annotation:
public @interface MyCustomAnnotation {
String value();
}
In this code:
@interface
denotes that we are defining a new annotation calledMyCustomAnnotation
.- The method
value()
serves as an attribute that can hold information when the annotation is used.
You can apply this annotation, for example:
@MyCustomAnnotation(value = "This is a demo annotation")
public class DemoClass {
// Class implementation
}
In the above example, MyCustomAnnotation
is applied to DemoClass
, storing the string "This is a demo annotation".
Understanding Retention Policy
Retention policy determines how long annotations are kept and whether they are available at runtime. It is crucial for enabling or restricting features based on when you target these annotations. The retention policy is specified using @Retention
, which can take one of three values:
- SOURCE
- CLASS
- RUNTIME
Let's discuss each retention policy in detail.
1. SOURCE
Annotations with SOURCE
retention are discarded by the compiler. They are not incorporated into the compiled class files.
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.SOURCE)
public @interface SourceOnly {
String value();
}
In the example above, the SourceOnly
annotation will only exist in the source code and won't be available during the compilation or at runtime. This can be useful for annotations intended solely for documentation.
2. CLASS
An annotation with CLASS
retention is recorded in the class files but is not available for reflection at runtime.
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.CLASS)
public @interface ClassRetention {
String value();
}
Unlike SOURCE
, class retention preserves the annotation in the .class
file. However, you can’t access it using reflection in the running program. This is useful for annotations meant for processing by tools but don’t have a presence during runtime.
3. RUNTIME
Annotations marked with RUNTIME
retention are accessible during the execution of the program through reflection.
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
@Retention(RetentionPolicy.RUNTIME)
public @interface RuntimeRetention {
String value();
}
This is the most commonly used retention policy, especially in frameworks like Spring or JPA, where annotations determine behavior at runtime. You can read these annotations programmatically using reflection:
import java.lang.reflect.Method;
public class AnnotationDemo {
@RuntimeRetention(value = "Example Method")
public void annotatedMethod() {
// Method implementation
}
public static void main(String[] args) {
Method[] methods = AnnotationDemo.class.getDeclaredMethods();
for (Method method : methods) {
if (method.isAnnotationPresent(RuntimeRetention.class)) {
RuntimeRetention annotation = method.getAnnotation(RuntimeRetention.class);
System.out.println("Annotation value: " + annotation.value());
}
}
}
}
In this code snippet:
- We define a method
annotatedMethod
with@RuntimeRetention
. - In the
main
method, we use reflection to check ifannotatedMethod
has theRuntimeRetention
annotation and print its value.
Why Retention Matters
The retention policy of annotations can turn your code into a more dynamic system or simply necessitate code cleanliness. The choice of retention can significantly impact how, when, and where your annotations will be applied.
-
Performance: Using
SOURCE
orCLASS
retention can help in compiling cleaner bytecode with minimal margins of error when reflective capabilities are not required. -
Framework Compatibility: Libraries like Spring depend heavily on runtime retention. Choosing the right retention policy is crucial for frameworks to inspect annotations at runtime.
-
Tooling and Documentation: If you are developing tools to analyze code, source or class retention might suffice as they save memory and avoid the overhead associated with runtime reflection.
Wrapping Up: Choosing the Right Retention Policy
Java annotations provide immense flexibility and power in the architecture of your applications. Understanding the implications of the retention policy plays a pivotal role in ensuring that annotations serve their intended purpose effectively.
Choose SOURCE
for annotations not needed beyond code-level documentation, CLASS
for compile-time checks only, and RUNTIME
when proper execution context is required.
For further reading on annotations, be sure to explore Java's official documentation and the various frameworks that extensively utilize annotations like Spring and Hibernate.
By owning this knowledge, you can leverage annotations smartly and create highly manageable, readable, and efficient Java applications. Remember, the style of coding you adopt today will set the foundation for scalable and maintainable code tomorrow. Happy coding!
Checkout our other articles