Fixing Groovy SQL's Invalid Column Index Exception in Oracle
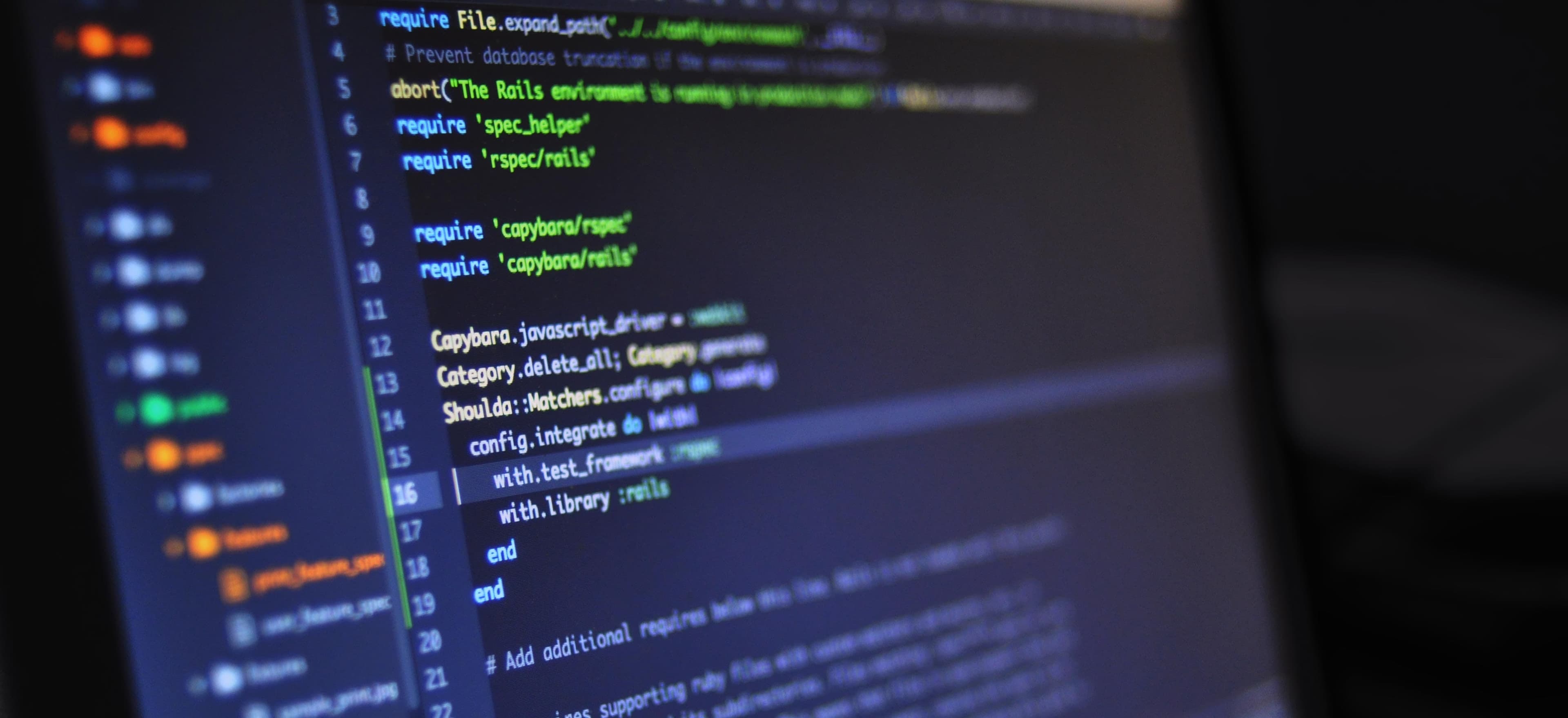
- Published on
Fixing Groovy SQL's Invalid Column Index Exception in Oracle
When working with databases in Java, you might have encountered Groovy SQL's Invalid Column Index
exception while connecting to an Oracle database. This issue can disrupt the flow of your application, and finding the root cause often feels like looking for a needle in a haystack. In this post, we will delve into the reasons for this exception and how to mitigate it with practical examples.
What is Groovy SQL?
Groovy SQL is a powerful extension that allows developers to interact seamlessly with SQL databases using Groovy code. By simplifying many tasks, such as executing queries and managing connections, Groovy SQL enables developers to write cleaner and more maintainable code.
Understanding the Invalid Column Index Exception
Before jumping into the solution, let's understand what an Invalid Column Index
exception is. This exception typically occurs when you attempt to access a column in a result set that does not exist. Common causes include:
- Incorrect column index
- Mismatched data types
- Faulty SQL query
To address this issue effectively, one must identify which of the above factors is causing the exception.
Example Scenario
Imagine you have the following code snippet using Groovy SQL to query an Oracle database:
import groovy.sql.Sql
def dbUrl = 'jdbc:oracle:thin:@localhost:1521:yourdb'
def user = 'your_user'
def password = 'your_password'
def sql = Sql.newInstance(dbUrl, user, password, 'oracle.jdbc.OracleDriver')
def query = 'SELECT id, name FROM users WHERE id = ?'
def userId = 1
sql.eachRow(query, [userId]) { row ->
println "User ID: ${row[0]}, Name: ${row[1]}"
}
In this code, we are trying to fetch a user's ID and name. However, if the column names are incorrect or if user ID does not exist, you may end up with the Invalid Column Index
exception.
Debugging the Exception
-
Check Column Names: Always ensure that your SQL query is correct. If the column names "id" and "name" are incorrect or do not exist in the table, it will throw the exception.
-
Confirm Data Types: Data types should be compatible. If you are supplying a value that does not match the expected type (for instance, passing a String instead of an Integer), it can lead to issues.
-
Validating Indexes: In Groovy, the index of a result set starts from zero. However, if you are confused with one-based indexing from the SQL perspective, it might lead to incorrect index access.
Finalizing the Code Example
Here’s an improved and more robust version of the previous code snippet with error handling and comments explaining each part:
import groovy.sql.Sql
def dbUrl = 'jdbc:oracle:thin:@localhost:1521:yourdb'
def user = 'your_user'
def password = 'your_password'
def sql = Sql.newInstance(dbUrl, user, password, 'oracle.jdbc.OracleDriver')
// Correct SQL Query
def query = 'SELECT id, name FROM users WHERE id = ?'
def userId = 1
try {
sql.eachRow(query, [userId]) { row ->
// Accessing using index; ensure zero-based indexing
println "User ID: ${row[0]}, Name: ${row[1]}"
}
} catch (groovy.sql.SqlException e) {
// Catch specific SQL exceptions
println "SQL Error: ${e.message}"
} catch (Exception e) {
// Handle potential general exceptions
println "An unexpected error occurred: ${e.message}"
} finally {
// Always close the SQL connection
sql.close()
}
Key Improvements
- Error Handling: Use try-catch blocks for better error management.
- Closing Connections: Always remember to close database connections to avoid resource leaks.
- Comments for Clarity: Adding comments helps both others and your future self understand the rationale behind your code decisions.
Performance Best Practices
While fixing the exception is one part of the solution, we should continuously strive for optimizing our database interaction:
- Use Connection Pooling: If your application makes frequent database calls, employing a connection pool can enhance performance by reusing existing connections.
- Prepared Statements: As used in the example, prepared statements help prevent SQL injection and can improve efficiency for repeated query executions.
Further Reading
To deepen your understanding of database interactions in Java and Groovy, consider the following resources:
- Groovy SQL Documentation
- JDBC Best Practices
Key Takeaways
Dealing with database exceptions, such as the Groovy SQL Invalid Column Index
, can be challenging. By understanding the underlying causes and implementing robust code practices, you can resolve the issue effectively and enhance the resilience of your application. Happy coding!
Feel free to reach out in the comments if you need further clarification or assistance with your specific use cases!
Checkout our other articles