Unlocking the Myth: Double-Checked Locking Flaws in Java
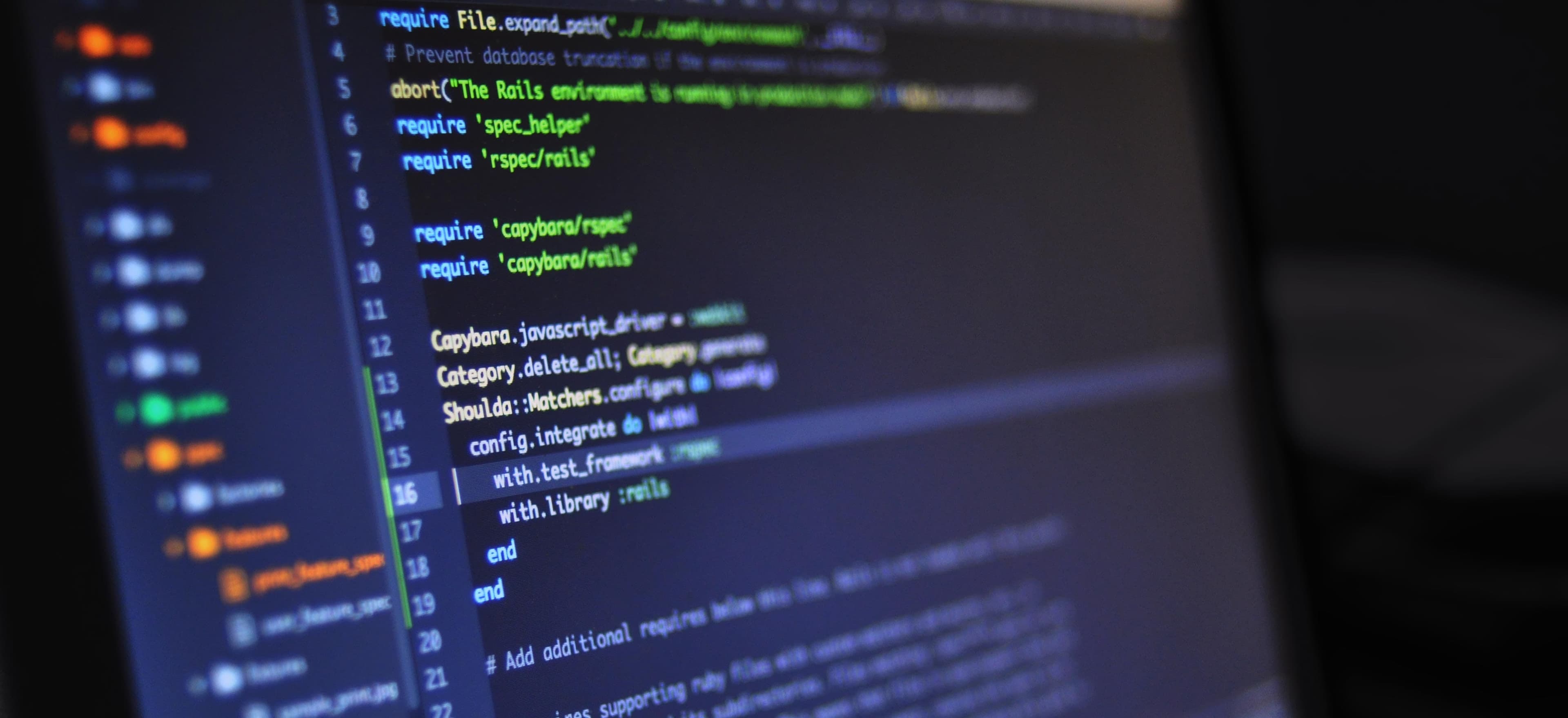
- Published on
Unlocking the Myth: Double-Checked Locking Flaws in Java
Concurrency in Java can be tricky. Among the many synchronization techniques, double-checked locking is often cited as a reliable way to improve performance when using lazy initialization in a multithreaded environment. However, this pattern has its pitfalls. In this blog post, we will dive deep into double-checked locking, exposing its flaws and offering alternative solutions.
What is Double-Checked Locking?
Double-checked locking is a design pattern that reduces the overhead of acquiring a lock by first checking the locking criterion (e.g., whether or not the object is initialized) without actually acquiring the lock. Ideally, if the object is initialized, acquiring the lock for the subsequent checks can be avoided.
Consider the following classic example:
public class Singleton {
private static Singleton instance;
private Singleton() {
// private constructor to prevent instantiation
}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
Breakdown of the Code
-
Instance Variable:
private static Singleton instance;
declares the variable where the singleton instance will be stored. -
Private Constructor:
private Singleton()
prevents instantiation from outside this class. -
First Check:
if (instance == null)
checks if the instance is uninitialized. -
Synchronized Block:
synchronized (Singleton.class)
ensures that only one thread can execute this block at a time, which prevents multiple instances from being created. -
Second Check: Once inside the synchronized block, we perform the check again with
if (instance == null)
before creating the instance.
The Flaws of Double-Checked Locking
While this pattern seems efficient, it has a significant flaw related to the Java Memory Model (JMM). Specifically, it can lead to the creation of multiple instances when accessed by multiple threads. This occurs due to memory visibility issues, which can happen when an instance is partially constructed.
Example of Potential Flaw
Consider the following scenario:
- Thread A executes
if (instance == null)
, which evaluates totrue
. - Thread A enters the synchronized block and starts constructing the Singleton instance.
- Before Thread A assigns the newly created instance to the variable, Thread B executes
if (instance == null)
and also evaluates totrue
. - Thread B enters the synchronized block and may create a new Singleton instance, resulting in two instances.
Why This Happens
The issue arises from how the Java Virtual Machine (JVM) handles memory allocation and initialization. During the construction of an object, the constructor is not completed until the reference is assigned. Consequently, there is a brief moment when instance
is not yet fully constructed but is accessible.
Alternatives to Double-Checked Locking
Knowing the pitfalls of double-checked locking, you may wonder: what alternatives exist? Fortunately, Java provides several strategies to implement singleton patterns safely and efficiently.
1. Eager Initialization
One of the simplest ways to ensure a single instance is to create it at class loading time:
public class EagerSingleton {
private static final EagerSingleton instance = new EagerSingleton();
private EagerSingleton() {
// private constructor
}
public static EagerSingleton getInstance() {
return instance;
}
}
Why Choose Eager Initialization?
- Thread-Safe: Since the instance is created when the class is loaded, you do not have to worry about synchronization.
- Simplicity: The implementation is straightforward, making it easier to maintain.
2. Static Inner Class Initialization
Another effective technique leverages Java’s lazy initialization feature through a static inner class:
public class InnerClassSingleton {
private InnerClassSingleton() {
// private constructor
}
private static class Holder {
private static final InnerClassSingleton INSTANCE = new InnerClassSingleton();
}
public static InnerClassSingleton getInstance() {
return Holder.INSTANCE;
}
}
Benefits of the Static Inner Class Approach
- Lazy Loaded: This implementation delays the instantiation of the Singleton until the
Holder
class is accessed. - Thread Safety: The JVM handles the class loading mechanism, ensuring that only one instance is created, thus providing inherent thread safety.
3. Enum Singleton
Java also supports the Singleton pattern through enumeration, which is inherently thread-safe:
public enum EnumSingleton {
INSTANCE;
public void someMethod() {
// some logic
}
}
Advantages of Using Enum for Singleton
- Built-In Serialization Support: Enums in Java are serializable by default.
- Protection against Reflection: An enum instance cannot be instantiated through reflection, preventing the creation of duplicate instances.
Bringing It All Together
Understanding the flaws of double-checked locking in Java is essential for developers handling concurrency. This pattern, while seemingly efficient, can lead to multiple instances being created under certain conditions due to the inadequacies of memory visibility.
By employing alternatives such as eager initialization, static inner classes, or enumeration, you can avoid the pitfalls associated with double-checked locking while achieving your design goals.
For further reading on concurrency in Java, feel free to check out Java Concurrency in Practice or dive into Java's Memory Model for a more detailed understanding.
Choosing the right implementation can provide not just safety but also performance benefits in your Java applications. Always remember to assess the requirements of your project and choose a pattern that aligns with those needs. Happy coding!
Checkout our other articles