Mastering IntentService for Smooth Web Service Calls in Android
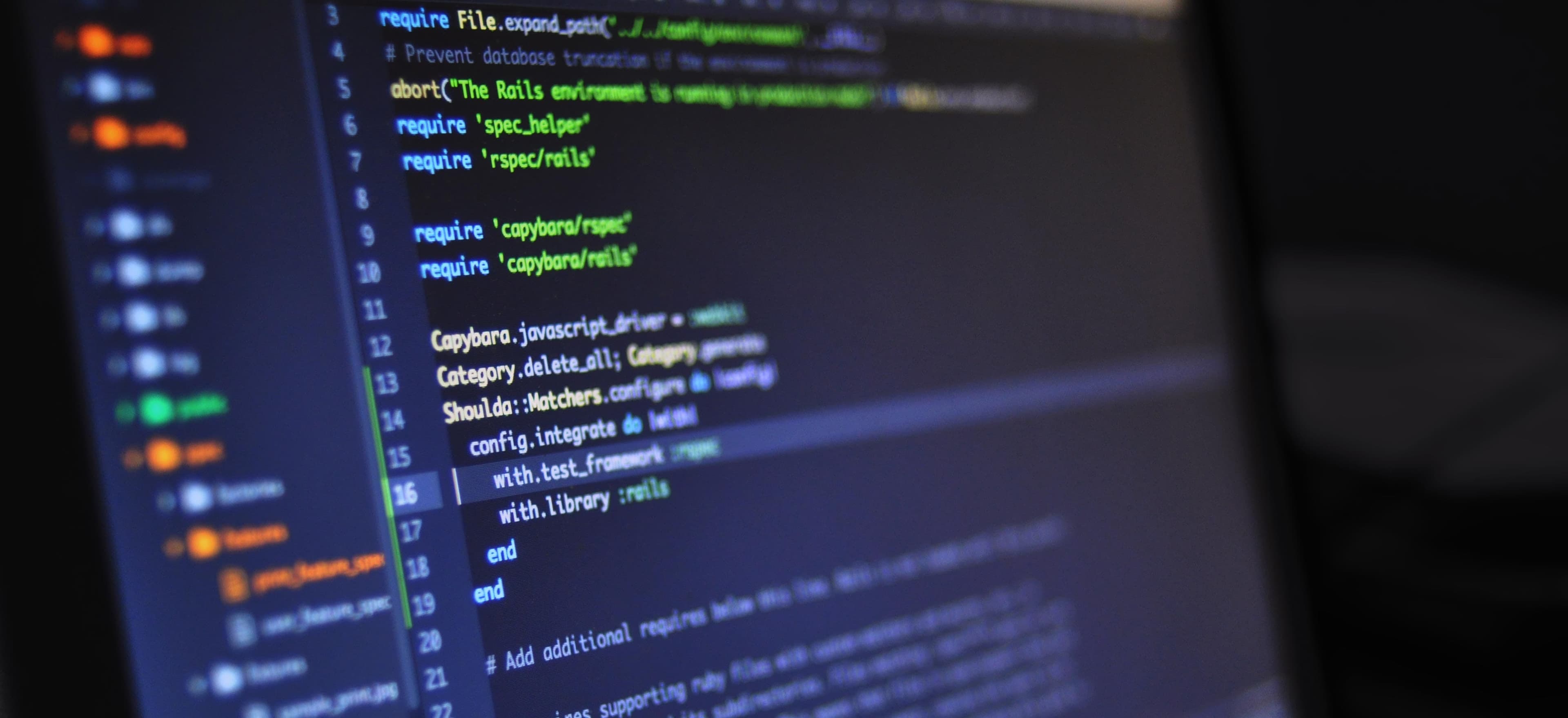
- Published on
Mastering IntentService for Smooth Web Service Calls in Android
In the Android realm, smooth UI performance is paramount. One of the underlying principles for achieving this is by performing long-running operations outside the main thread. For web service calls, the use of IntentService can be particularly advantageous. In this blog post, we will explore how to effectively utilize IntentService for smooth web service calls in Android applications.
What is IntentService?
An IntentService
is a specialized type of Service
. It provides functionalities that make it simple for you to perform background operations in a more efficient manner. When you implement an IntentService, it handles threading for you, which means you don’t have to manage background threads manually.
Key Features of IntentService:
- Specialized for Work Queues: It processes intents sequentially, which means it executes one at a time ensuring that each intent is processed to completion.
- Automatic Termination: It automatically stops itself when the work is done.
- Built-in Threading: It runs on a separate thread which simplifies concurrent work management.
Understanding these features sets the stage for our discussions on implementing web service calls.
Setting Up Your Project
Before we dive deeper, let’s ensure your Android project is ready to use IntentService.
- Create a new Android project using Android Studio or open an existing one.
- Add Internet permissions in your
AndroidManifest.xml
:
<uses-permission android:name="android.permission.INTERNET"/>
- Create a new Java class for your IntentService.
Create a Custom IntentService
Let’s implement a basic IntentService
for making a web service call. Here’s how you can create your MyIntentService
class:
public class MyIntentService extends IntentService {
public MyIntentService() {
super("MyIntentService");
}
@Override
protected void onHandleIntent(Intent intent) {
// Your main logic goes here.
fetchWebServiceData();
}
private void fetchWebServiceData() {
String urlString = "https://api.example.com/data"; // Your API endpoint
try {
URL url = new URL(urlString);
HttpURLConnection urlConnection = (HttpURLConnection) url.openConnection();
try {
InputStream in = new BufferedInputStream(urlConnection.getInputStream());
String jsonResponse = readStream(in);
// Handle the JSON response as needed
} finally {
urlConnection.disconnect();
}
} catch (IOException e) {
e.printStackTrace();
}
}
private String readStream(InputStream inputStream) {
StringBuilder result = new StringBuilder();
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream));
String line;
try {
while ((line = bufferedReader.readLine()) != null) {
result.append(line);
}
} catch (IOException e) {
e.printStackTrace();
}
return result.toString();
}
}
Explanation of the Code
-
IntentService Constructor:
- The constructor invokes
super()
with a name for the worker thread.
- The constructor invokes
-
onHandleIntent:
- This method is invoked when the service is started. It functions similarly to a
doInBackground
method found inAsyncTask
.
- This method is invoked when the service is started. It functions similarly to a
-
fetchWebServiceData:
- The actual web service call is performed here. We make use of
HttpURLConnection
to initiate a connection to the provided URL, read the response stream, and manage exceptions carefully.
- The actual web service call is performed here. We make use of
-
readStream:
- This method handles converting the InputStream into a String. It effectively encapsulates the reading logic separate from network calls.
Starting the IntentService
You can start your IntentService from an Activity
or Fragment
as follows:
Intent serviceIntent = new Intent(this, MyIntentService.class);
startService(serviceIntent);
Why Use IntentService Instead of AsyncTask?
While AsyncTask
is often used for short background tasks, it has limitations on lifecycle management and can lead to memory leaks if not properly handled. IntentService, on the other hand, automatically manages threads and is more suitable for operations that can take a long time, such as networking.
For a comparison of using AsyncTask vs. IntentService
, you can check out the documentation on AsyncTasks.
Handling Service Responses
Upon successfully fetching data from a web service, you might want to update the UI or save the data. Here’s how you can handle that:
Send a Broadcast
You can communicate results back to the main thread using a LocalBroadcastManager
:
private void sendResponseBroadcast(String jsonResponse) {
Intent intent = new Intent("com.example.ACTION_RESPONSE");
intent.putExtra("response", jsonResponse);
LocalBroadcastManager.getInstance(this).sendBroadcast(intent);
}
In your main activity, register a receiver:
private BroadcastReceiver responseReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String response = intent.getStringExtra("response");
// Update your UI with this response
}
};
@Override
protected void onStart() {
super.onStart();
LocalBroadcastManager.getInstance(this).registerReceiver(responseReceiver, new IntentFilter("com.example.ACTION_RESPONSE"));
}
@Override
protected void onStop() {
super.onStop();
LocalBroadcastManager.getInstance(this).unregisterReceiver(responseReceiver);
}
Robust Error Handling
Since network operations can frequently result in exceptions, robust error handling is crucial. Here are some best practices:
- Use Try-Catch: Wrap your network calls with try-catch statements to catch
IOException
. - Check Network Connectivity: Before making a web service call, ensure the device is connected to the Internet.
- Handle HTTP Response Codes: Always check response codes from the server. For example, handle
404 (Not Found)
or500 (Server Error)
appropriately.
Lessons Learned
In summary, using IntentService
for web service calls allows for efficient, manageable, and seamless background operations in your Android applications. This service is especially beneficial for ensuring that your UI remains smooth and responsive.
Take advantage of this approach for making web service calls while keeping your app's performance optimal. For more in-depth exploration of Android Services, visit the official Android Developers page.
If you have any experiments or additional features you'd like to implement with IntentService, feel free to share them in the comments section below! Happy coding!