Common Pitfalls in Simple Security Rules Implementation
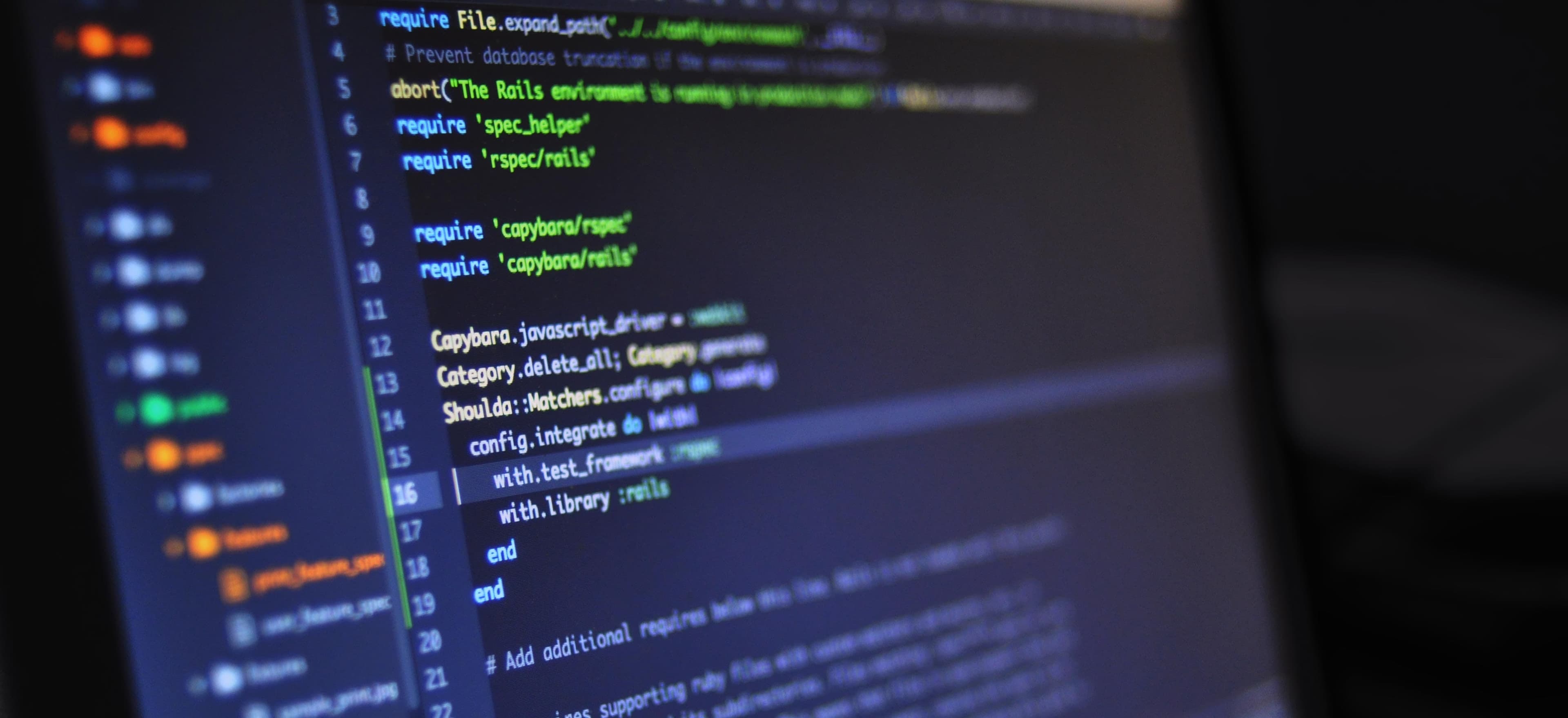
- Published on
Common Pitfalls in Simple Security Rules Implementation
In today's rapidly evolving digital landscape, security remains a paramount concern for developers and organizations alike. While implementing security measures, it's essential to start with simple security rules. However, even straightforward implementations can lead to significant vulnerabilities if not handled correctly. In this post, we will explore common pitfalls one might encounter in the implementation of simple security rules, alongside best practices to mitigate these risks.
Why Simple Security Rules Matter
Simple security rules are often the first line of defense for applications, especially in larger systems. They ensure that basic security principles are in place, providing a foundation for more complex security measures. However, simple doesn’t mean easy. Flaws in their implementation can expose applications to various threats, such as unauthorized access, data breaches, and compliance risks.
1. Lack of Understanding of Security Principles
One of the most critical pitfalls in security rule implementation is the lack of understanding of fundamental security principles. Developers may implement rules without grasping the "why" behind them.
Best Practice: Invest time in understanding security fundamentals. Resources such as the OWASP Top Ten offer excellent guidance on the most common security vulnerabilities and how to address them.
2. Overlooking Input Validation
Input validation is a crucial aspect of security. Developers sometimes assume that inputs are safe without proper validation. Failure to clean and validate user input can lead to SQL injection, cross-site scripting (XSS), and other vulnerabilities.
Code Example: Basic Input Validation in Java
public class UserInput {
public static boolean isValidEmail(String email) {
String emailRegex = "^[a-zA-Z0-9_+&*-]+(?:\\.[a-zA-Z0-9_+&*-]+)*@(?:[a-zA-Z0-9-]+\\.)+[a-zA-Z]{2,7}$";
return email.matches(emailRegex);
}
}
Why This Matters: This regex pattern checks for a standard format of an email address, preventing malformed inputs from sneaking into your application. Always validate and sanitize input to ensure that your application remains robust against injection attacks.
3. Hardcoding Credentials
It's tempting to hardcode sensitive information such as API keys, passwords, or database connection strings directly in code for convenience. However, this practice poses a significant risk if the code is ever leaked or extracted.
Best Practice: Use environment variables or secure vaults like AWS Secrets Manager to manage sensitive information.
4. Implementing Insecure Default Configurations
Security misconfigurations are rampant, especially when defaults are left unchanged. For example, a database's or API's default settings often favor usability over security.
Best Practice: Always review and modify default configurations. For instance, with databases, ensure that root access is restricted, and appropriate roles are assigned.
5. Ignoring Exception Handling
Poorly handled exceptions can reveal sensitive information about your application’s architecture or expose pointers to potential vulnerabilities.
Code Example: Exception Handling in Java
public class UserService {
public User getUserById(String userId) {
try {
// Code to fetch user from the database
} catch (SQLException e) {
log.error("Database error occurred.", e); // Log technical error
throw new CustomException("Could not retrieve user. Please try again."); // User-friendly message
}
}
}
Why This Matters: Here, the SQL exception is logged for internal review, while a generic and user-friendly message is presented to the end user. This ensures sensitive implementation details are not exposed.
6. Insufficient Access Controls
Implementing security rules without stringent access control can lead to unauthorized access to critical functions. This often happens in the haste to develop features quickly.
Best Practice: Implement role-based access control (RBAC) to regulate user access appropriately. Tools like Spring Security can be instrumental in managing authentication and authorization.
7. Failing to Use Encryption
Encryption is often overlooked or underutilized in simple security rule implementations. Data at rest and in transit should always be encrypted to prevent unauthorized access.
Code Example: Encrypting Data in Java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class EncryptionUtil {
public static byte[] encrypt(String data) throws Exception {
SecretKey key = KeyGenerator.getInstance("AES").generateKey();
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(data.getBytes());
}
}
Why This Matters: In this example, we demonstrate the AES encryption algorithm to secure data. Always use industry-standard encryption algorithms to protect sensitive information.
8. Ignoring Logging and Monitoring
Monitoring for unusual activities or potential breaches can mitigate security risks. Not implementing comprehensive logging can leave a blind spot in detecting attacks.
Best Practice: Incorporate centralized logging and monitoring solutions. Platforms like ELK Stack can help maintain logs and identify unusual patterns.
9. Lack of Security Testing
Finally, after implementing security rules, it's critical to test these measures thoroughly. Simple security rules should be periodically reviewed and tested in various scenarios to identify potential weaknesses.
Tools for Security Testing:
- OWASP ZAP (Zed Attack Proxy)
- Burp Suite
Bringing It All Together
Security is an ongoing process that requires continual assessment and enhancement. While simple security rules are the first step, one must be vigilant about the pitfalls outlined above to ensure robust implementation. Remember that understanding the "why" behind security measures leads to more thoughtful coding practices and, ultimately, a more secure application.
By adhering to best practices and leveraging available tools, developers can fortify their applications against common vulnerabilities and contribute to a safer digital environment.
For more insights into security best practices and emerging threats, consider visiting The Hacker News or Krebs on Security.
This blog post provides a comprehensive overview of common pitfalls in security rule implementations, featuring practical coding examples and best practices. Share your thoughts in the comments below, and let's create a more secure coding culture together!