Common Jenkins Configuration Pitfalls for Spring Boot CI/CD
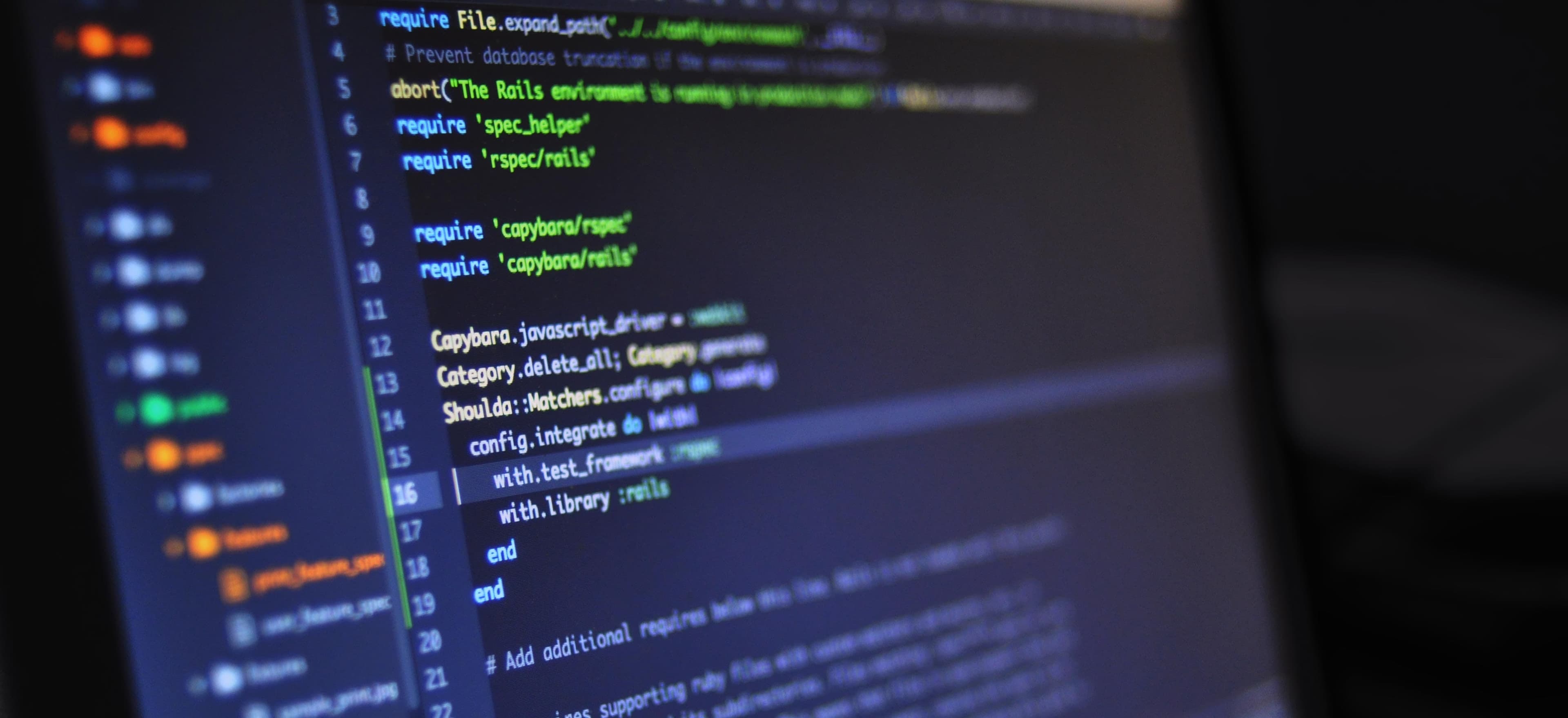
- Published on
Common Jenkins Configuration Pitfalls for Spring Boot CI/CD
Setting up Continuous Integration and Continuous Deployment (CI/CD) pipelines in Jenkins for your Spring Boot applications can be straightforward. However, several common pitfalls can derail even the most well-planned deployments. In this blog post, we will explore these pitfalls, providing insight, solutions, and code snippets to help you establish a robust Jenkins configuration for your Spring Boot projects.
Table of Contents
- Understanding CI/CD
- Pitfall 1: Inadequate Environment Variables
- Pitfall 2: Misconfigured Build Triggers
- Pitfall 3: Ignoring Dependency Management
- Pitfall 4: Inconsistent Artifact Management
- Pitfall 5: Insufficient Testing
- Conclusion
Understanding CI/CD
Before diving into pitfalls, let’s briefly discuss what CI/CD means. Continuous Integration is the practice of automatically building and testing your code every time a change is made, ensuring that integration issues are caught early. Continuous Deployment, on the other hand, extends CI by automatically deploying code changes to production after passing tests. Jenkins is one of the most popular CI/CD tools widely used for automating these processes.
Pitfall 1: Inadequate Environment Variables
An often-overlooked aspect of Jenkins job configuration is the setup of environment variables. Spring Boot applications frequently rely on various environment configurations to operate correctly. Missing or incorrectly set environment variables can cause unexpected behavior.
Solution
Ensure that you define all necessary environment variables either in Jenkins’ global configurations or per job as needed. You can set them in the Jenkins interface or script them in the pipeline.
Here’s how to define environment variables in a Jenkins Pipeline script:
pipeline {
agent any
environment {
SPRING_PROFILE = 'production'
MYSQL_HOST = 'localhost'
MYSQL_PORT = '3306'
}
stages {
stage('Build') {
steps {
sh 'echo $SPRING_PROFILE'
// Compile and package your Spring Boot application
sh './mvnw clean package'
}
}
}
}
Why
Using environment variables allows you to modify behavior without changing your source code. For example, switching between profiles or databases becomes seamless.
Pitfall 2: Misconfigured Build Triggers
Another common pitfall occurs when build triggers are not configured properly. Insufficient triggers can lead to missed builds, and overly aggressive triggers can cause unnecessary builds, wasting resources and time.
Solution
Configure triggers carefully. A popular option is to use Git hooks or webhooks that trigger builds on pull requests or merges. Here’s an example of a simple trigger for Git in a Jenkins Pipeline:
pipeline {
agent any
triggers {
pollSCM('H/5 * * * *') // Polls SCM every 5 minutes
}
stages {
...
}
}
Why
Configuring triggers helps manage resources efficiently, ensuring that builds happen only under relevant conditions, improving your CI/CD workflow.
Pitfall 3: Ignoring Dependency Management
Ignoring proper dependency management can lead to build failures or runtime errors. Missing dependencies or conflicting versions often cause builds to fail or applications to crash.
Solution
Utilize tools like Maven or Gradle effectively. Always define your dependencies correctly in your pom.xml
or build.gradle
file.
For example, in a Maven project:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.5.4</version>
</dependency>
...
</dependencies>
Why
Correctly managing dependencies ensures that the application has everything it needs to run smoothly and helps avoid version conflicts and build errors.
Pitfall 4: Inconsistent Artifact Management
Poor artifact management can lead to confusion and errors during deployment. Consistently managing artifacts across builds is crucial for traceability and rollback capabilities.
Solution
Make use of artifact repositories like Nexus or Artifactory to store your built applications. Here’s how you might push an artifact to Nexus:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh './mvnw clean package'
}
}
stage('Publish') {
steps {
sh '''
mvn deploy:deploy-file -DgroupId=com.example \
-DartifactId=myapp \
-Dversion=1.0.0 \
-Dpackaging=jar \
-Dfile=target/myapp-1.0.0.jar \
-DrepositoryId=nexus-repo \
-Durl=http://your-nexus-repo-url
'''
}
}
}
}
Why
Using an artifact repository centralizes your artifacts in a secure location, making it easier for teams to find and use the correct versions, thus avoiding deployment errors.
Pitfall 5: Insufficient Testing
One of the most critical phases in CI/CD is testing. Rushing through this phase can lead to undetected issues, which may result in production failures.
Solution
Implement comprehensive testing strategies within your pipeline. Include unit tests, integration tests, and functional tests. Use tools such as JUnit and Mockito for unit testing.
Here’s an example of integrating a test stage in your Jenkins Pipeline:
pipeline {
agent any
stages {
...
stage('Test') {
steps {
sh './mvnw test'
}
}
}
}
Why
Automating testing in your Jenkins pipeline ensures that each build maintains integrity and quality. It allows for fast feedback loops, helping you catch and resolve issues early in the development lifecycle.
Final Thoughts
Setting up a Jenkins CI/CD pipeline for your Spring Boot application can be a smooth process if you are aware of the common pitfalls. By paying attention to environment variables, build triggers, dependency management, artifact management, and comprehensive testing, you can avoid many issues that may arise.
Implementing these steps will enhance the reliability of your CI/CD process, ultimately leading to smoother deployments and a better development experience.
For further reading on continuous integration with Jenkins, consider visiting the official Jenkins documentation. You'll find additional features and advanced configurations that can take your CI/CD process to the next level.
Happy coding!
Checkout our other articles