Troubleshooting Common Issues in File Polling with Spring Integration
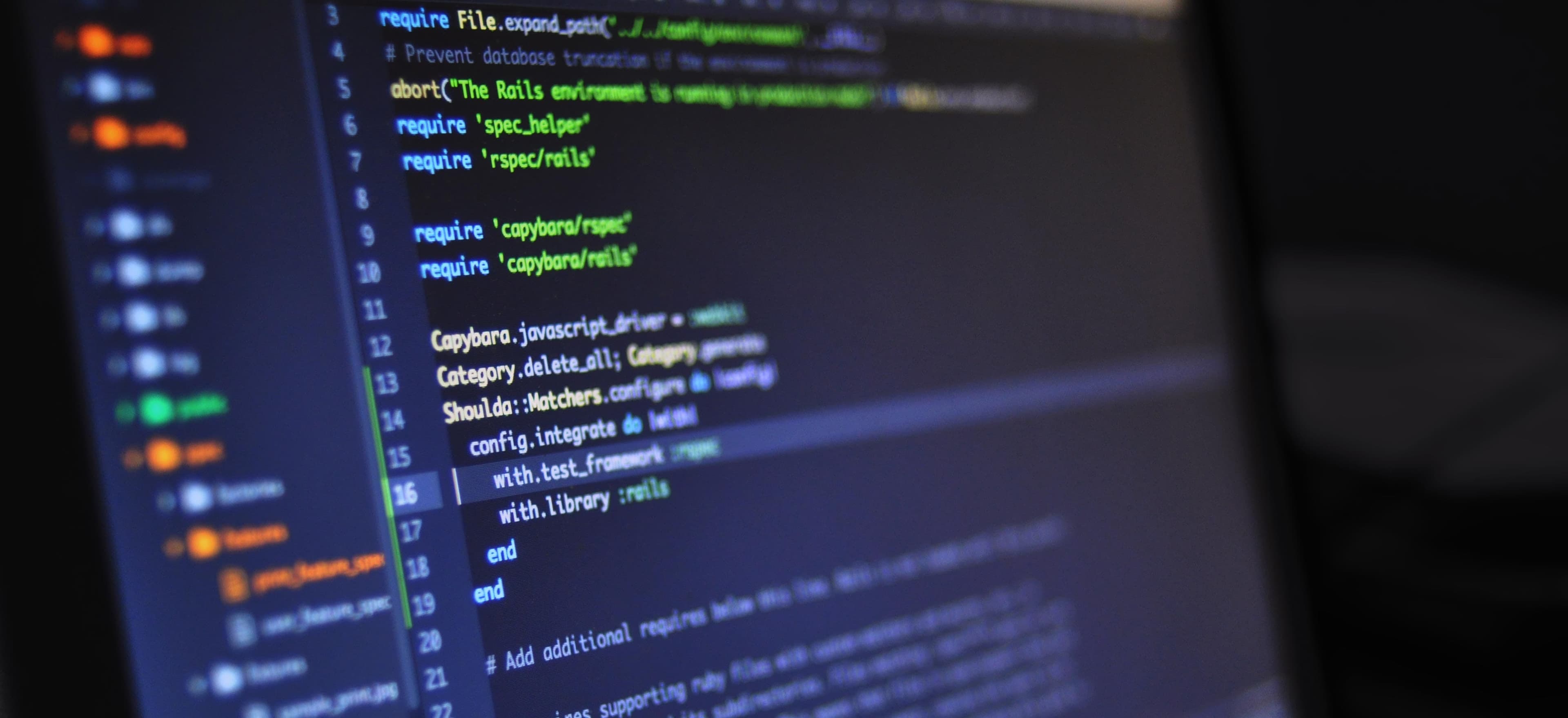
- Published on
Troubleshooting Common Issues in File Polling with Spring Integration
Spring Integration is a powerful framework that allows developers to build complex event-driven applications. One of its frequently utilized features is file polling, which helps applications monitor directories for new files and process them as soon as they appear. While Spring Integration simplifies many tasks, challenges can still arise, especially for newcomers. In this blog post, we will explore common issues encountered in file polling using Spring Integration, their solutions, and how to implement a robust file polling mechanism.
What is File Polling in Spring Integration?
File polling refers to the process where an application regularly checks a specified directory for new files. In Spring Integration, this is typically implemented using poller
and file
components that work together to automate file processing.
Basic Setup of File Polling
Before diving into troubleshooting, let's establish a basic file polling configuration using Spring Integration. You'll need to set up your Maven or Gradle project with the appropriate dependencies.
Maven Dependency:
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-file</artifactId>
</dependency>
Java Configuration:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.integration.annotation.EnableIntegration;
import org.springframework.integration.config.EnableIntegration;
import org.springframework.integration.file.FileReadingMessageSource;
import org.springframework.integration.file.FileWritingMessageHandler;
import org.springframework.integration.scheduling.PollerMetadata;
import org.springframework.integration.dsl.IntegrationFlow;
import org.springframework.integration.dsl.IntegrationFlows;
import org.springframework.messaging.MessageHandler;
import org.springframework.scheduling.concurrent.ThreadPoolTaskScheduler;
import java.io.File;
@Configuration
@EnableIntegration
public class FilePollingConfig {
@Bean
public FileReadingMessageSource fileReadingMessageSource() {
FileReadingMessageSource source = new FileReadingMessageSource();
source.setDirectory(new File("input-directory"));
return source;
}
@Bean
public MessageHandler fileWritingMessageHandler() {
FileWritingMessageHandler handler = new FileWritingMessageHandler(new File("output-directory"));
handler.setExpectReply(false);
return handler;
}
@Bean
public IntegrationFlow fileIntegrationFlow() {
return IntegrationFlows.from(fileReadingMessageSource(), c -> c.poller(poller()))
.handle(fileWritingMessageHandler())
.get();
}
@Bean
public PollerMetadata poller() {
return Pollers.fixedDelay(5000).get();
}
}
In the example above, we configure a file reading message source to poll an input directory every 5 seconds and write the files to an output directory. Understanding this basic structure will help in troubleshooting issues.
Common Issues in File Polling
Now, let’s examine frequent problems developers encounter when implementing file polling with Spring Integration, along with their solutions.
1. Files Not Being Detected
Possible Causes:
- Polling interval too long.
- Files not appearing in the configured directory.
Solution:
First, double-check the input directory path in your configuration. Ensure that the specified directory is correct and accessible. Additionally, consider modifying the polling interval to a shorter duration for testing purposes.
Example:
@Bean
public PollerMetadata poller() {
// Reduce the interval for faster testing
return Pollers.fixedDelay(1000).get();
}
2. Duplicate File Processing
Possible Causes:
- Lack of a file lock mechanism.
- Files not being deleted or moved after processing.
Solution:
Implement a FileLock
or use unique identifiers for processed files to avoid reprocessing. Also, configure the FileWritingMessageHandler
to rename or delete the processed files after successfully handling them.
Example:
handler.setOutputChannelName("nullChannel"); // Prevent further processing
handler.setFileExistsMode(FileExistsMode.REPLACE); // Replace existing files
3. File Format Issues
Possible Causes:
- The application assumes a specific file format that may differ from the actual files.
Solution:
Implement error handling and logging mechanisms to track the type of incoming files. You can extend your integration flow to validate formats before processing.
Example:
.handle((payload) -> {
if (!isValidFormat(payload)) {
throw new IllegalArgumentException("Invalid file format.");
}
return payload;
})
4. Error Handling During Processing
Possible Causes:
- Unhandled exceptions during file processing.
Solution:
Spring Integration provides robust error handling, allowing you to define an error channel in case of failures. Use this to route error messages and log exceptions accordingly.
Example of configuring an error channel:
@Bean
public IntegrationFlow errorHandlingFlow() {
return IntegrationFlows.from("errorChannel")
.handle(message -> {
// Handle error
System.err.println(message.getPayload());
})
.get();
}
5. Memory Issues
Possible Causes:
- Too many files being processed simultaneously.
Solution:
Control the concurrency limit in your integration flow to prevent high memory consumption. Use Executor
parameters to manage thread pools.
Example:
@Bean
public IntegrationFlow fileIntegrationFlow() {
return IntegrationFlows.from(fileReadingMessageSource(), c -> c.poller(poller().maxMessagesPerPoll(5)))
.handle(fileWritingMessageHandler())
.get();
}
6. Polling “Stuck”
Possible Causes:
- Polling thread might be blocked due to long processing time or heavy load.
Solution:
Always ensure that file processing is quick and efficient. If processing takes longer, consider moving the processing to a separate thread or microservice. Use Spring’s asynchronous support or message queues such as RabbitMQ or Kafka for heavy workloads, allowing the poller to continue working on new files.
Final Considerations
File polling in Spring Integration is a powerful feature that simplifies file processing tasks. However, common issues can arise that may hinder its effectiveness. By understanding potential challenges and implementing the suggested solutions outlined in this post, developers can create a reliable and efficient file polling system.
Feel free to explore the Spring Integration documentation for more detailed insights and examples. Consistent testing and monitoring are key to maintaining an effective application. Happy coding!
Additional Resources
For further reading and details, here are some relevant resources:
By integrating these practices, you can enhance your file polling setups and troubleshoot effectively.
Checkout our other articles