Unlocking Apache APISIX: When to Build a Custom Plugin
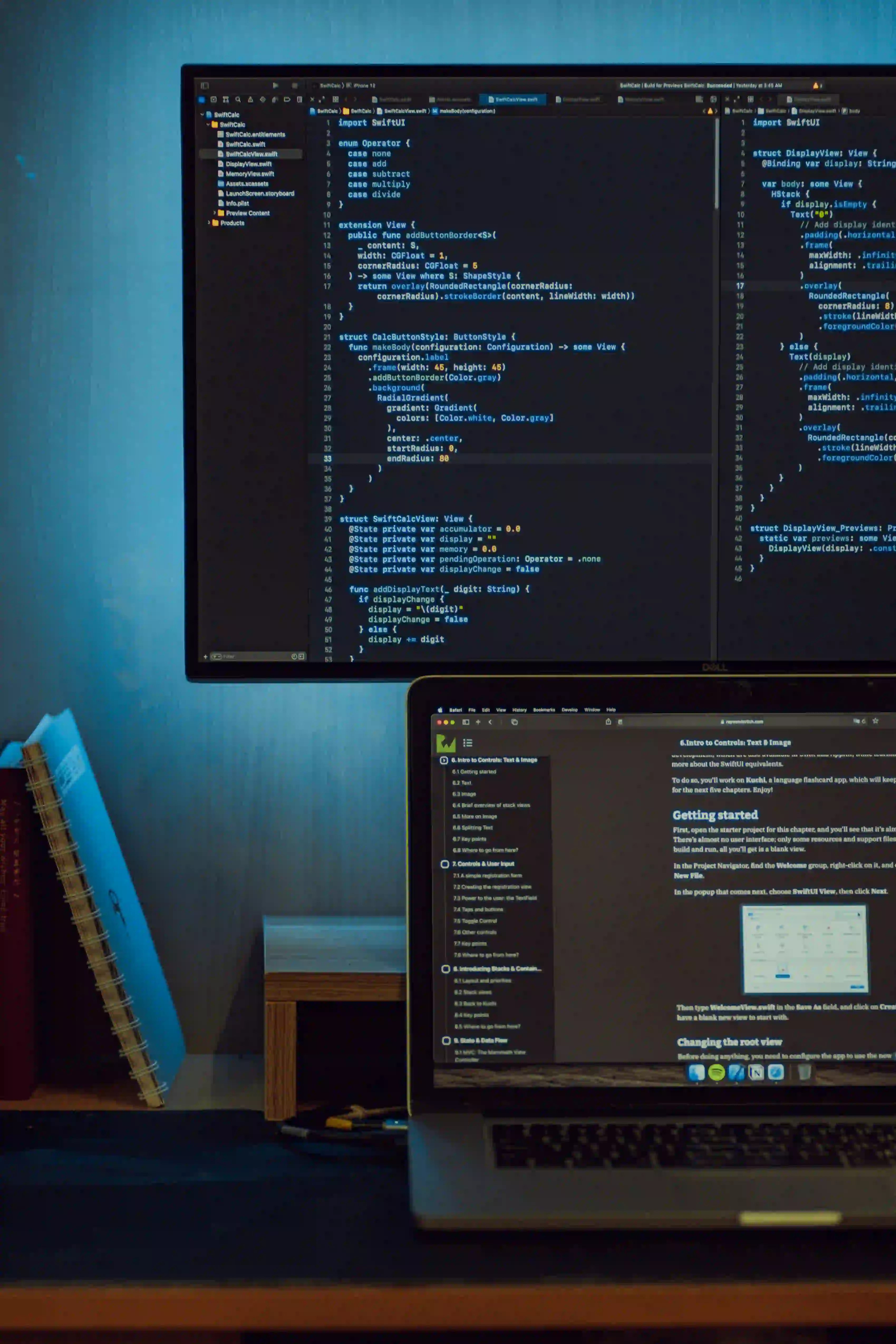
Unlocking Apache APISIX: When to Build a Custom Plugin
In the world of microservices and API management, Apache APISIX stands out as a dynamic and flexible gateway solution. Built on top of NGINX, it provides an abundant set of out-of-the-box features that simplify API management. However, sometimes these built-in capabilities may not meet your specific needs. This is where building a custom plugin comes into play. In this blog post, we will explore the scenarios in which you might want to build a custom plugin for Apache APISIX, how to do it, and the benefits of taking this approach.
Understanding Apache APISIX Architecture
Before diving into plugins, itβs essential to understand how Apache APISIX works. Built on a modular architecture, APISIX handles incoming API requests, routing them to various services based on specified rules. The key components of APISIX include:
- Load Balancing: Efficient request distribution across multiple service instances.
- Traffic Control: Rate limiting, request / response transformation, and more.
- Security: Authentication, authorization, and IP whitelisting/blacklisting.
With these features built-in, you can easily manage API traffic without modifying your core application. But what if you have unique requirements?
When Should You Build a Custom Plugin?
While APISIX boasts a rich set of plugins, there are specific scenarios where developing a custom plugin becomes indispensable:
1. Unique Business Logic
If your API demands specific business rules or workflows that are not addressed by the existing plugins, customizing one can help encapsulate this logic, ensuring that it executes seamlessly with your API requests.
Example: You might have a custom authentication mechanism based on OAuth2 tokens that existing authentication plugins do not cover.
2. Specialized Metrics and Monitoring
If you want to track specific metrics that aren't supported by standard monitoring plugins, creating a custom plugin enables you to gather, store, or report this specialized data.
Example: Custom latency measurements or A/B testing tracking that needs to be collected alongside regular metrics.
3. Customized Request/Response Manipulation
In many cases, you may want to alter requests and responses in a way that existing plugins do not easily allow.
Example: Adding specific headers or modifying payload structures before forwarding requests to your backend services.
4. Integration with External Systems
Sometimes, your custom business processes integrate external systems that arenβt compatible with existing APISIX features or plugins.
Example: When interfacing with bespoke CRM or third-party services that require specific interaction patterns.
5. Compliance and Security Requirements
If your organization adheres to strict regulatory standards, you may need a plugin that implements compliance features tailored to your policies.
Example: Data masking according to GDPR standards for specific fields in your request and response.
Building a Custom Plugin
Creating a custom plugin in Apache APISIX is more straightforward than it sounds. Letβs break down the process into actionable steps.
Step 1: Understand the Plugin Architecture
APISIX plugins are Lua scripts that extend the functionality of the gateway. They can hook into various phases of request processing. The main lifecycle phases are:
- Access: Triggered when a request hits APISIX.
- Rewrite: Modify the request before it is sent to the backend service.
- Pre-Processing: Additional analysis or actions.
- Response: Modify response data before returning to the client.
Step 2: Create the Plugin Structure
Assume you're building a custom plugin called custom-auth
. The structure would look like this:
apisix/
βββ lua/
βββ apisix/
βββ plugin/
βββ custom-auth.lua
Step 3: Write the Lua Code
Here is a basic code snippet for a custom authentication plugin:
local core = require("apisix.core")
local _M = {}
_M.version = 0.1
function _M.access(config, ctx)
local token = ctx.req.headers["Authorization"]
-- Check if the Authorization header exists
if not token or not validate_token(token) then
return 401, "Unauthorized"
end
-- Continue processing if the token is valid
return 200, "Authorized"
end
function validate_token(token)
-- Add your custom authentication logic here.
-- For the sake of example, we will just check for a placeholder token.
return token == "valid-token"
end
return _M
Commentary on the Code:
- Access Function: This function is key to validating incoming requests. It checks for the
Authorization
header and verifies the token.- A successful validation proceeds, while failure results in a
401 Unauthorized
response.
- A successful validation proceeds, while failure results in a
- Custom Validation Logic: The modular design allows you to replace the
validate_token
function with any sophisticated algorithm, including calls to external systems, databases, or token introspection endpoints.
Step 4: Deploy Your Plugin
Once you write your plugin, you need to integrate and enable it within APISIX by configuring it in your APISIX configuration files (usually config.yaml
).
plugins:
- custom-auth
After modifying the configuration, ensure you restart APISIX for the changes to take effect.
Step 5: Test Your Plugin
Conduct thorough testing to ensure your plugin behaves as expected. You can use tools like Postman or cURL to test API requests against your custom authentication middleware:
curl -H "Authorization: valid-token" http://localhost:9080/your-api-endpoint
# Should return 200 Authorized
Closing Remarks
Building a custom plugin for Apache APISIX can be a game-changer for organizations with unique API requirements. By leveraging the extensibility offered by APISIX, you can ensure your APIs are aligned perfectly with your business needs. For more detailed guidelines, review the official APISIX documentation and explore more about Lua programming if youβre new to it.
Embrace the power of customization, and unlock the full potential of Apache APISIX for enhanced API management!