Overcoming Common Pitfalls in Java 8 TDD with JUnit
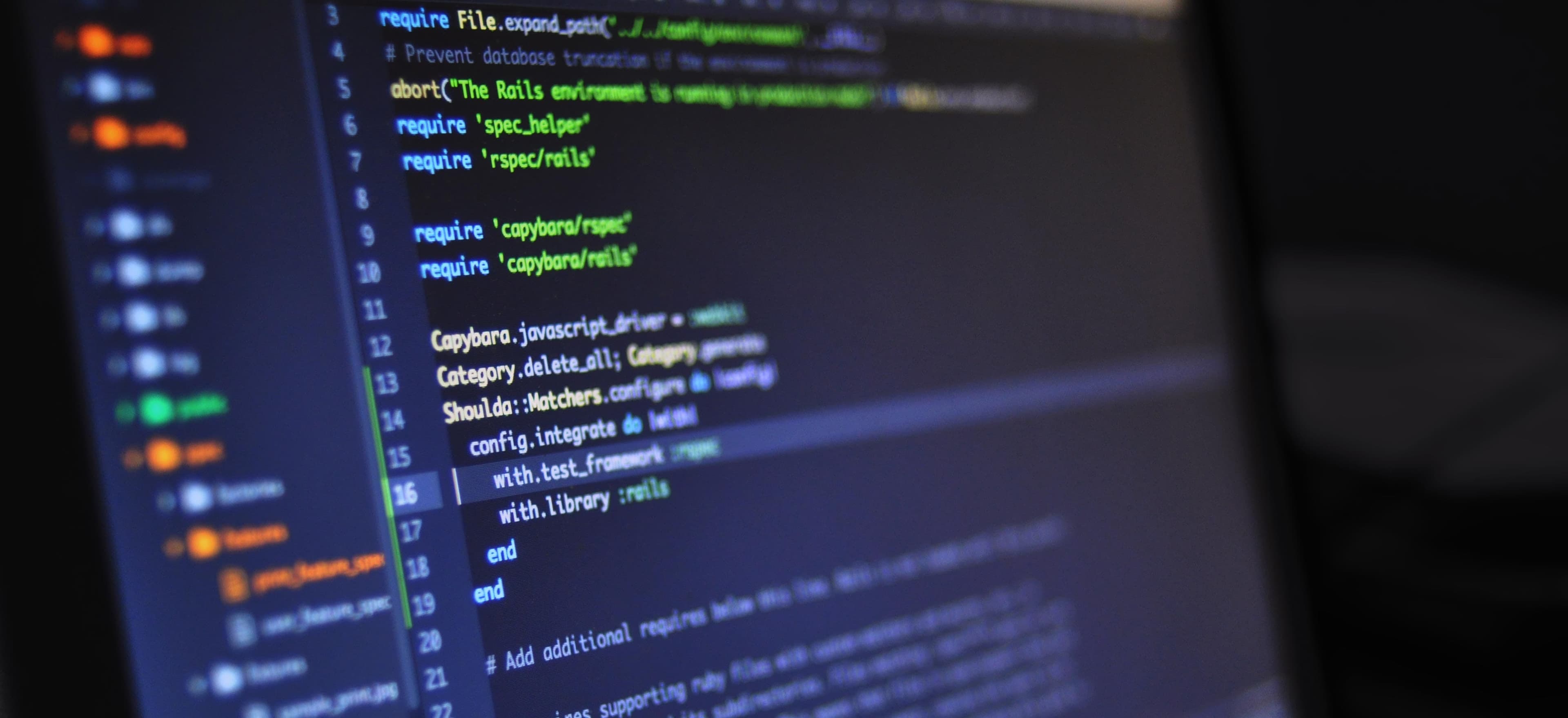
- Published on
Overcoming Common Pitfalls in Java 8 TDD with JUnit
Test-Driven Development (TDD) transforms the way developers approach coding. While TDD has numerous benefits, mastering its implementation, especially in Java with JUnit, can be challenging. In this article, we'll explore some common pitfalls that developers encounter when adopting TDD in Java 8 and provide practical solutions to help you improve your workflow.
What is Test-Driven Development (TDD)?
TDD is an agile development practice in which you write tests before you write the corresponding code. The TDD cycle is often summarized by the mantra "Red, Green, Refactor." Here's how it works:
- Red: Write a failing test.
- Green: Write enough code to pass the test.
- Refactor: Clean up the code while keeping the tests green.
This approach ensures that you are continuously validating your code and helps prevent regression bugs.
Common Pitfalls in Java 8 TDD
1. Inadequate Test Coverage
One of the biggest pitfalls in TDD is writing insufficient tests. Developers may write only a few tests, missing critical components of their code, leading to inadequate test coverage.
Solution:
Aim for 100% coverage of your critical paths. Use tools like JaCoCo or Cobertura to analyze which parts of your code are not covered by tests.
Here’s an example of a simple Java class and its corresponding JUnit test:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
The test might look like this:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
assertEquals(0, calculator.add(-2, 2));
}
}
By testing both positive and negative scenarios, we ensure better coverage.
2. Overly Complex Tests
Another pitfall is writing overly complex tests. When tests become complicated, they are harder to read and maintain.
Solution:
Keep your tests simple and focused. Each test should verify one aspect of your code.
Consider enhancing the above test with helper methods for clarity:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
assertEquals(5, add(2, 3));
assertEquals(0, add(-2, 2));
}
private int add(int a, int b) {
Calculator calculator = new Calculator();
return calculator.add(a, b);
}
}
3. Ignoring Edge Cases
Edge cases are the scenarios that can cause an application to behave unexpectedly. Ignoring these can lead to catastrophic failures in production.
Solution:
Identify and test edge cases. Every method should have corresponding tests for boundary values.
For example, when testing a method that divides two numbers, consider edge cases like division by zero:
public class Divider {
public double divide(int a, int b) {
if (b == 0) {
throw new ArithmeticException("Division by zero");
}
return (double) a / b;
}
}
The test should include division by zero:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class DividerTest {
@Test(expected = ArithmeticException.class)
public void testDivisionByZero() {
Divider d = new Divider();
d.divide(1, 0);
}
}
4. Not Writing Tests for Refactored Code
Developers often forget to write tests after refactoring their code, mistakenly believing that the previous tests are sufficient.
Solution:
Always add or adjust tests when you refactor code. This practice ensures that the integrity of the code is not compromised.
When reasons for refactoring arise (such as optimizing the method), maintain and enhance your test cases simultaneously.
public class OptimizedCalculator {
public int add(int... numbers) {
return Arrays.stream(numbers).sum();
}
}
You should adjust your tests accordingly:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class OptimizedCalculatorTest {
@Test
public void testAddition() {
OptimizedCalculator calculator = new OptimizedCalculator();
assertEquals(5, calculator.add(2, 3));
assertEquals(0, calculator.add(-2, 2));
assertEquals(10, calculator.add(1, 2, 3, 4));
}
}
5. Misunderstanding Testing Frameworks
JUnit, the most commonly used testing framework in Java, has features that many developers may not fully understand, leading to improper usage.
Solution:
Don't hesitate to explore the full capabilities of JUnit. Familiarize yourself with annotations like @Before
, @After
, @BeforeClass
, and @AfterClass
.
Here's how to use JUnit effectively:
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
public class MyTest {
@Before
public void setUp() {
// Code to set up test environment
}
@Test
public void testSomething() {
// Test code
}
@After
public void tearDown() {
// Clean up after tests
}
}
6. Treating Tests as Afterthoughts
Sometimes developers write tests only when they have time or when they think of them. Treating tests as afterthoughts can create a huge gap in the quality of code.
Solution:
Ingrain testing into the development process. Make it consistent by integrating testing into your regular workflow.
Design tasks with TDD principles in mind from the outset. Always ask: "What scenarios need testing for this feature?"
My Closing Thoughts on the Matter
Adopting TDD with JUnit in Java 8 can significantly enhance the reliability and quality of your code. By overcoming common pitfalls — inadequate test coverage, complex tests, ignoring edge cases, neglecting tests during refactoring, misunderstanding testing frameworks, and treating tests as afterthoughts — you position yourself to foster a development environment focused on quality.
Empowering yourself with robust testing practices not only boosts your confidence as a developer but also leads to software that meets user needs and stands the test of time.
For deeper insights into Java testing and TDD, consider exploring JUnit documentation and resources on best practices in testing.
Happy coding!