Java Developers: Avoid Shell Script Pitfalls in CI/CD
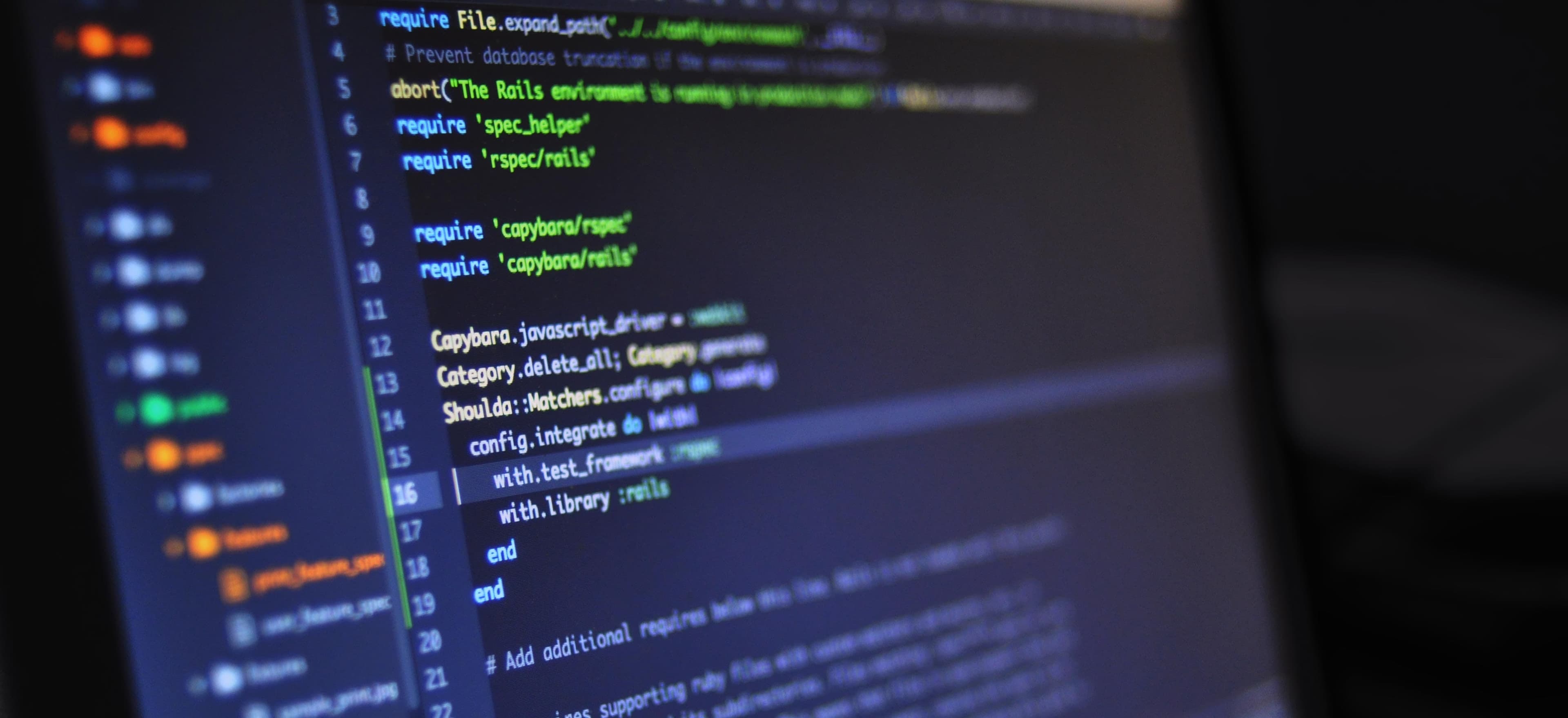
- Published on
Java Developers: Avoid Shell Script Pitfalls in CI/CD
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential in modern software development practices. They automate the process of testing and deploying applications, offering rapid feedback and saving release time. However, as a Java developer, you may find yourself entangled in the twists and turns of shell scripts to manage these workflows. While shell scripts can be powerful tools, they are fraught with pitfalls that can lead to frustrating roadblocks, especially for developers who are more accustomed to Java than to command-line interfaces.
In this article, we will explore some common mistakes Java developers make when working with shell scripts in a CI/CD environment and how to avoid them. Additionally, we'll provide examples to illustrate better practices.
Understanding Shell Scripts in CI/CD
Before we delve into the mistakes, let’s first clarify why shell scripts are commonly used in CI/CD pipelines. Shell scripts enable developers to automate tasks like building, testing, and deploying applications. They can orchestrate several commands to be executed in sequence, making them indispensable for automating complex workflows.
For example, a simple shell script might be used to compile Java code, run tests, and package the application. Here’s a minimal script for such a process:
#!/bin/bash
# Compile the Java application
javac -d bin src/com/example/Main.java
# Navigate to the bin directory
cd bin
# Run the tests
java -cp . com.example.TestRunner
# Package the application
jar -cvf MyApplication.jar com/example/*.class
In this script, each command has a specific role in the CI/CD pipeline. The javac
command compiles the Java code, followed by the test runner execution using Java, and finally, it packages the compiled classes into a JAR file.
Common Shell Script Mistakes
Let's break down some pitfalls Java developers often encounter when leveraging shell scripts in their CI/CD processes.
1. Not Handling Errors Gracefully
One common mistake is neglecting error handling in shell scripts. If a command fails, the script continues executing, generating more errors.
Correct Approach:
#!/bin/bash
# Stop execution on errors
set -e
# Compile the application
javac -d bin src/com/example/Main.java
# If the compilation fails, the script will exit
Adding set -e
at the beginning of the script tells the shell to exit immediately if any command exits with a non-zero status. This approach keeps your CI/CD pipeline robust and mitigates cascading failures.
2. Using Hardcoded Paths
Hardcoding paths is another pitfall that Java developers might overlook. Paths can vary depending on the environment, leading to broken scripts when moving from local development to CI environments.
Best Practice:
#!/bin/bash
# Define a base directory dynamically
BASE_DIR="$(dirname "$(realpath "$0")")"
# Use the base directory for file paths
javac -d "$BASE_DIR/bin" "$BASE_DIR/src/com/example/Main.java"
Using realpath
allows you to dynamically resolve the script's location, ensuring it works regardless of where it gets executed.
3. Lack of Comments and Documentation
While Java often benefits from verbose comments, shell scripts often get overlooked in this department. A lack of comments can lead to confusion for whoever reads the script later, including yourself.
Example:
#!/bin/bash
# Compile the Java application
javac -d bin src/com/example/Main.java # Build the application classes
# Run unit tests
java -cp bin com.example.TestRunner # Execute tests in the bin directory
Incorporating comments enhances code readability and aids in understanding the purpose and function of each command.
Additional Resources
To further enhance your scripting skills, consider reading our article titled Mastering Shell Scripts: Overcoming Common Beginner Mistakes at configzen.com/blog/mastering-shell-scripts-beginner-mistakes. It provides valuable insights that will assist you in avoiding many common scripting errors.
4. Forgetting Shell Compatibility
Errors may arise from using shell-specific features without considering compatibility across different systems. For example, bash
may not behave the same way in sh
or other shell environments.
Solution:
Whenever possible, use POSIX-compliant features:
#!/bin/sh
# Use POSIX-compliant syntax
if [ -f "config.txt" ]; then
echo "Config file exists."
else
echo "Config file missing, aborting."
exit 1
fi
This approach increases the adaptability of your scripts across various environments.
5. Not Testing Scripts Independently
Java applications might feel natural to test within an IDE, but shell scripts often don’t get the same consideration. Failing to test scripts independently may lead to broken builds.
Testing Example:
Use a testing framework designed for shell scripts, such as Bats or shunit2.
# Example of a Bats test
@test "Compiles successfully" {
run bash script.sh
[ "$status" -eq 0 ]
}
This ensures your scripts behave as expected before integrating them into your CI/CD process.
The Bottom Line
With CI/CD becoming a staple in software development, Java developers must overcome the pitfalls associated with shell scripts. By adopting best practices like effective error handling, dynamic paths, adequate documentation, ensuring compatibility, and independent testing, you can enhance the reliability of your CI/CD processes.
As you work with shell scripts, keep these points in mind. You'll bolster not only your scripting skills but also the overall efficiency and reliability of your development workflow. Be sure to check out Mastering Shell Scripts: Overcoming Common Beginner Mistakes at configzen.com/blog/mastering-shell-scripts-beginner-mistakes for further guidance.
Happy coding!
Checkout our other articles