Avoiding Shell Script Pitfalls: Enhance Your Java Deployment
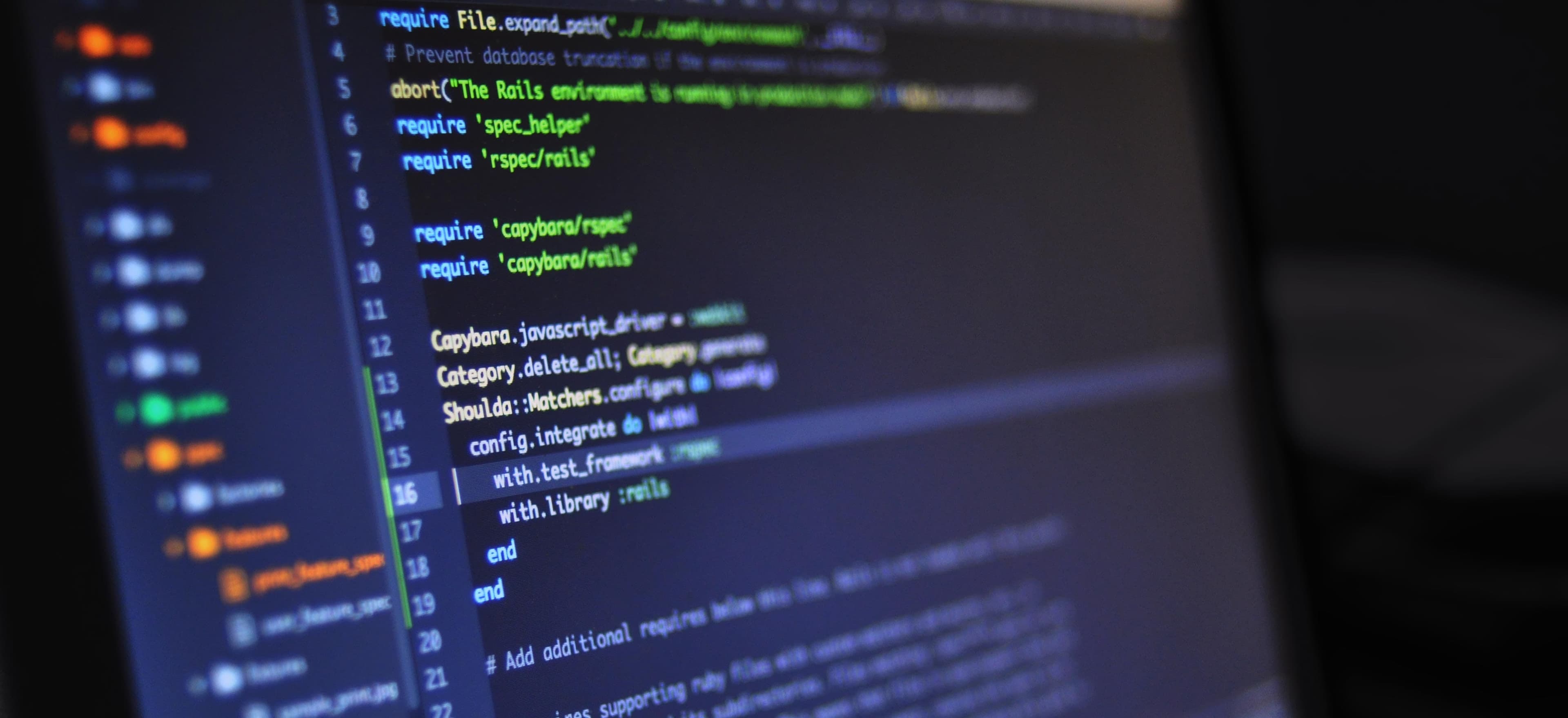
- Published on
Avoiding Shell Script Pitfalls: Enhance Your Java Deployment
In today's fast-paced development environment, deploying Java applications requires not only solid coding skills but also an understanding of the underlying deployment processes. Java developers often rely on shell scripts to automate deployment tasks. However, these scripts can present challenges, especially for those new to shell scripting. In this article, we will explore common pitfalls in shell scripting and provide best practices for enhancing your Java deployment processes. We will also reference an insightful article titled Mastering Shell Scripts: Overcoming Common Beginner Mistakes to further support our discussion.
The Importance of Shell Scripts in Java Deployment
Shell scripts help automate repetitive tasks involved in deploying Java applications, such as:
- Building the Application: Compiling Java code and packaging it into JAR files.
- Environment Setup: Configuring required environment variables and paths.
- Deployment: Copying JAR files to target servers and starting necessary services.
A well-written shell script can save time and enhance the reliability of the deployment process.
Common Pitfalls in Shell Scripts
While shell scripts are powerful tools, they can lead to issues if not written carefully. Here are some common pitfalls and how to avoid them:
1. Ignoring Exit Codes
One of the most frequent mistakes is neglecting to check exit codes. Each command in a shell script returns an exit code indicating whether the command succeeded or failed. This can lead to continued execution of subsequent commands even when an earlier command has failed.
Solution: Always check the exit status of commands.
#!/bin/bash
# Build the application
mvn clean install
if [ $? -ne 0 ]; then
echo "Build failed! Exiting."
exit 1
fi
# Deploy the application
scp target/myapp.jar user@server:/path/to/deploy/
if [ $? -ne 0 ]; then
echo "Deployment failed! Exiting."
exit 1
fi
echo "Deployment successful!"
In this example, the script checks if the mvn
command and scp
command fail, exiting the script to prevent further actions.
2. Using Unquoted Variables
Shell scripting can behave unexpectedly when variables contain spaces or special characters. Forgetting to quote variables can result in syntax errors or security vulnerabilities (such as command injection).
Solution: Always quote your variables.
#!/bin/bash
app_name="My Java App"
# Unquoted variable may fail
echo $app_name # Could break if there are spaces in the variable
# Quoted variable avoids issues
echo "$app_name"
By quoting the variable, we prevent any potential problems that might arise from unexpected spaces or characters.
3. Hardcoding Paths and Settings
Another common mistake is hardcoding file paths, configurations, or other settings directly in the script. If these values change, the script becomes brittle and requires modification.
Solution: Use configuration files or environment variables.
#!/bin/bash
# Load configurations from an external file
source ./config.sh
# Use environment variables
java -jar "$APP_HOME/myapp.jar"
Using a configuration file allows for easier modification without altering the shell script.
4. Neglecting Logging and Debugging
Many developers overlook the importance of logging and debugging in their shell scripts. If a script fails, it can be difficult to trace the problem without proper logs.
Solution: Implement logging.
#!/bin/bash
LOG_FILE="/var/log/my-deployment.log"
exec > >(tee -a "$LOG_FILE") 2>&1 # Redirect output to log file
echo "$(date) - Starting deployment."
# Build and log the build process
if ! mvn clean install; then
echo "Build failed."
exit 1
fi
echo "Build successful. Proceeding to deployment."
In this approach, all output, including errors, is logged to a designated log file. This makes debugging much more manageable.
5. Forgetting to Handle Signals
Sometimes, shell scripts can be interrupted due to various reasons (like a user pressing Ctrl+C). If not handled, this can leave your system in an inconsistent state.
Solution: Use trap to handle signals.
#!/bin/bash
cleanup() {
echo "Cleaning up temporary files..."
rm -rf /tmp/my_temp_files
}
trap cleanup SIGINT SIGTERM
# Main deployment steps here
echo "Deploying..."
# Simulate long-running process
sleep 60
In this example, if the user interrupts the script, the cleanup
function will run to remove temporary files.
Enhancing Your Java Deployment Workflow
By avoiding these common pitfalls, your Java deployment workflow can become smoother and more reliable. Here are some additional tips:
-
Version Control: Always use a version control system (such as Git) to manage changes to your shell scripts. This allows you to revert to previous versions if something goes wrong.
-
Testing: Test your scripts in a staging environment before deploying them to production. This can help catch errors early.
-
Automation Tools: Consider integrating tools like Jenkins for continuous integration and deployment (CI/CD). This can help manage the deployment process more efficiently and reliably.
-
Documentation: Document your shell scripts thoroughly, explaining the purpose of each section and any dependencies. This is crucial for maintaining code quality, especially when the script is shared among multiple team members.
-
Referring to Resources: For those looking to deepen their understanding of shell scripting, the article Mastering Shell Scripts: Overcoming Common Beginner Mistakes provides valuable insights and solutions to common challenges faced by beginners.
A Final Look
Shell scripts are indispensable tools in the Java developer's toolkit for automating deployment tasks. However, it is crucial to be aware of common pitfalls and implement best practices to ensure smooth operations. By focusing on error handling, quoting variables, avoiding hardcoded values, ensuring proper logging, and managing signals effectively, you can enhance your Java deployment workflow.
By taking the time to understand and improve your shell scripts, you will not only streamline your deployment process but also build a more robust and resilient Java application environment. Happy coding!
Checkout our other articles