Efficiently Process JSON Arrays in Java with MySQL Integration
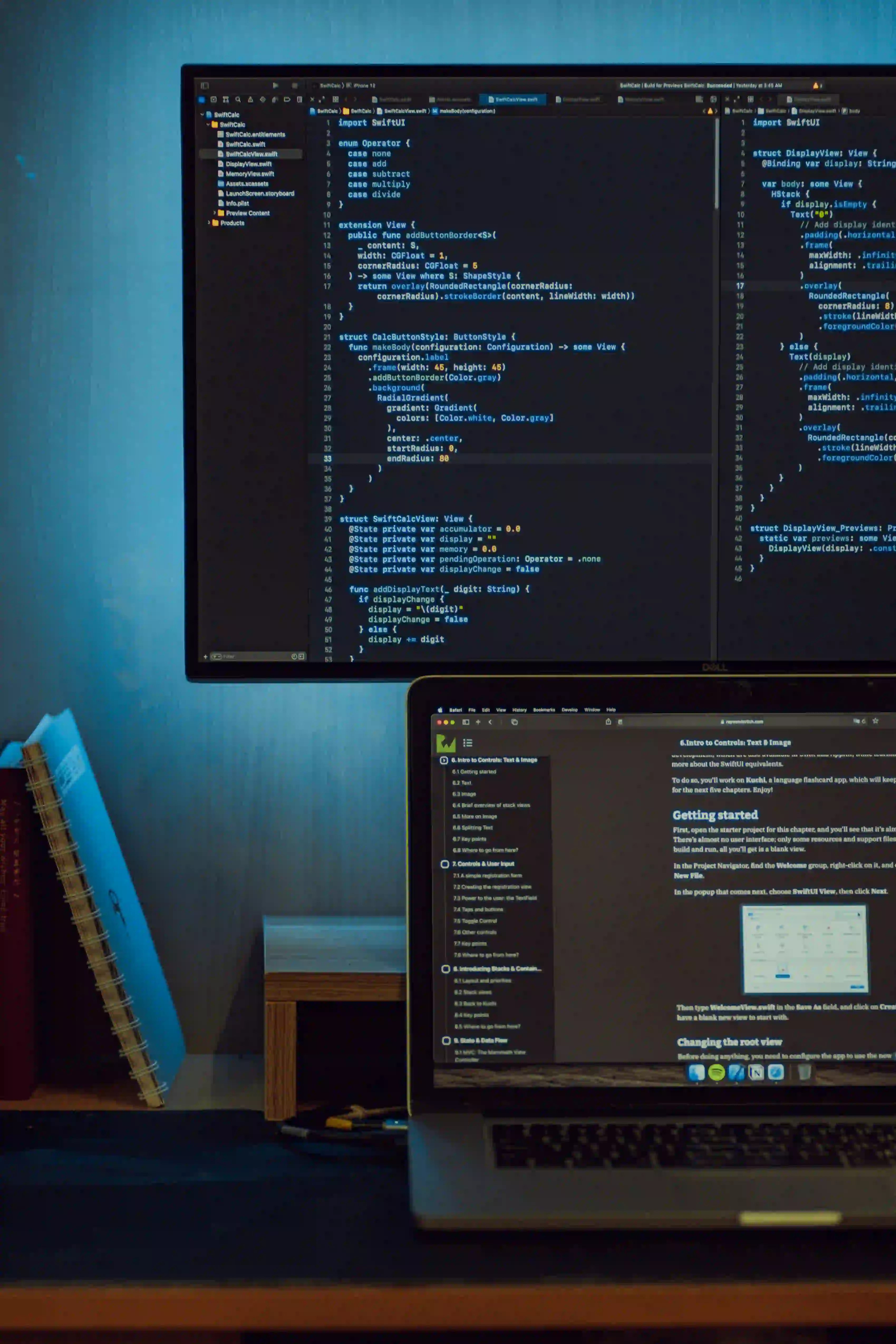
Efficiently Process JSON Arrays in Java with MySQL Integration
In today's data-driven world, JSON (JavaScript Object Notation) has become the de facto standard for data interchange. When combined with Java, the ability to manipulate these structures becomes even more powerful, especially when integrating with MySQL databases. This blog post will explore efficient ways to process JSON arrays in Java and how to integrate these operations with MySQL to unleash the full potential of your applications.
The Opening Bytes to JSON and MySQL
JSON's lightweight data-interchange format is celebrated for its simplicity and ease of use. It represents data as key-value pairs and arrays, making it intuitive for both humans and machines. MySQL, a robust relational database management system, has started supporting JSON as a native data type since version 5.7. This integration allows developers to store, query, and manipulate JSON data efficiently.
Why Use JSON with MySQL?
- Flexibility: JSON data is schema-less, meaning you can store different structures and types of data in the same column.
- Nested Structures: JSON allows for nested data, making it ideal for representing complex data hierarchies.
- Integration: Many APIs and web services return data in JSON format, making it convenient for applications.
By understanding how to effectively manipulate JSON in Java and MySQL, developers can enhance their applications and provide richer user experiences.
Getting Started
Before we dive into the implementation, you will need the following:
- Java Development Kit (JDK): Ensure you have Java installed on your system.
- MySQL Server: Set up a MySQL server to work with. Make sure it is version 5.7 or later to support JSON data types.
- JDBC Driver: Include the MySQL JDBC driver in your project to enable connectivity.
Setting Up Your MySQL Database
Let’s begin by creating a simple table that can hold JSON data:
CREATE TABLE user_data (
id INT AUTO_INCREMENT PRIMARY KEY,
user_info JSON
);
In this table, user_info
will hold JSON data representing user-related information.
Inserting JSON Data into MySQL Using Java
Now that your table is ready, let's see how to insert JSON data into the user_data
table using Java:
Example Code Snippet
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import org.json.JSONObject;
public class InsertJsonData {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static void main(String[] args) {
JSONObject userInfo = new JSONObject();
userInfo.put("name", "John Doe");
userInfo.put("age", 30);
userInfo.put("email", "john.doe@example.com");
try (Connection connection = DriverManager.getConnection(URL, USER, PASSWORD)) {
String sql = "INSERT INTO user_data (user_info) VALUES (?)";
try (PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setString(1, userInfo.toString());
statement.executeUpdate();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
In this snippet, we create a JSONObject
to hold user information. Using JDBC (Java Database Connectivity), we prepare an SQL statement to insert the JSON object into the user_data
table. This straightforward approach allows us to seamlessly store complex data structures directly.
Querying JSON Data
Once you've inserted JSON data, the next step is to retrieve it efficiently. MySQL provides various functions to access and manipulate JSON data.
Retrieving and Parsing JSON Data
Here's how you can retrieve and parse JSON data in Java:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class RetrieveJsonData {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static void main(String[] args) {
String sql = "SELECT user_info FROM user_data";
try (Connection connection = DriverManager.getConnection(URL, USER, PASSWORD);
PreparedStatement statement = connection.prepareStatement(sql);
ResultSet resultSet = statement.executeQuery()) {
while (resultSet.next()) {
String jsonString = resultSet.getString("user_info");
JSONObject userInfo = new JSONObject(jsonString);
System.out.println("Name: " + userInfo.getString("name"));
System.out.println("Age: " + userInfo.getInt("age"));
System.out.println("Email: " + userInfo.getString("email"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
The RetrieveJsonData
class connects to the MySQL database and executes a SQL query to retrieve user information. The JSON strings returned from the database are then parsed back into JSONObject
for easy access. This duality allows us to work with JSON quickly and efficiently.
Manipulating JSON Arrays
Sometimes, you need to handle JSON arrays. In the existing article, "Manipulating JSON Arrays in MySQL Tables", you will find detailed techniques on how to structure and manage JSON arrays within your database. Here, let's explore how to update JSON arrays with Java.
Updating JSON Arrays Example
Consider a scenario where you want to add a new phone number to an existing user’s information:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import org.json.JSONArray;
public class UpdateJsonArray {
private static final String URL = "jdbc:mysql://localhost:3306/your_database";
private static final String USER = "your_username";
private static final String PASSWORD = "your_password";
public static void main(String[] args) {
String newPhoneNumber = "123-456-7890";
String sql = "UPDATE user_data SET user_info = JSON_ARRAY_APPEND(user_info, '$.phoneNumbers', ?) WHERE id = ?";
try (Connection connection = DriverManager.getConnection(URL, USER, PASSWORD);
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setString(1, newPhoneNumber);
statement.setInt(2, 1); // Assuming we are updating user with ID 1
statement.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Explanation
In this example, we use MySQL's JSON_ARRAY_APPEND
function to add a new phone number to an existing JSON array stored in the user_info
field. The use of JSON functions in SQL enhances data manipulation directly within the database layer, reducing overhead in the application code.
Efficient JSON Queries
To leverage the full power of JSON in MySQL, it's essential to understand how to use JSON functions to filter and retrieve specific data.
Example of Efficient Queries
For instance, you might want to find all users over a certain age. This can be executed using the following SQL query:
SELECT * FROM user_data
WHERE JSON_UNQUOTE(JSON_EXTRACT(user_info, '$.age')) > 25;
This SQL statement uses JSON_EXTRACT
to access the age attribute in the JSON structure and returns users who meet the conditions. Integrating these queries into your Java application is seamless, providing a robust solution for handling complex data structures.
Bringing It All Together
By effectively integrating JSON with MySQL and Java, you can create applications that handle complex data structures with ease. Using JSON for data storage allows for flexibility and simplicity.
The code snippets provided illustrate how easy it is to insert, retrieve, and manipulate JSON data within a MySQL table using Java. Additionally, understanding how to use MySQL’s JSON functions maximizes performance and simplifies queries.
For a deeper dive into the intricacies of JSON in MySQL, be sure to check out the article on "Manipulating JSON Arrays in MySQL Tables".
As you continue your journey with Java and MySQL, keep these principles in mind — they will serve you well in creating efficient, data-driven applications.
References
- MySQL JSON Reference Documentation
- JSON in Java