Understanding Java's Biological Data Processing Capabilities
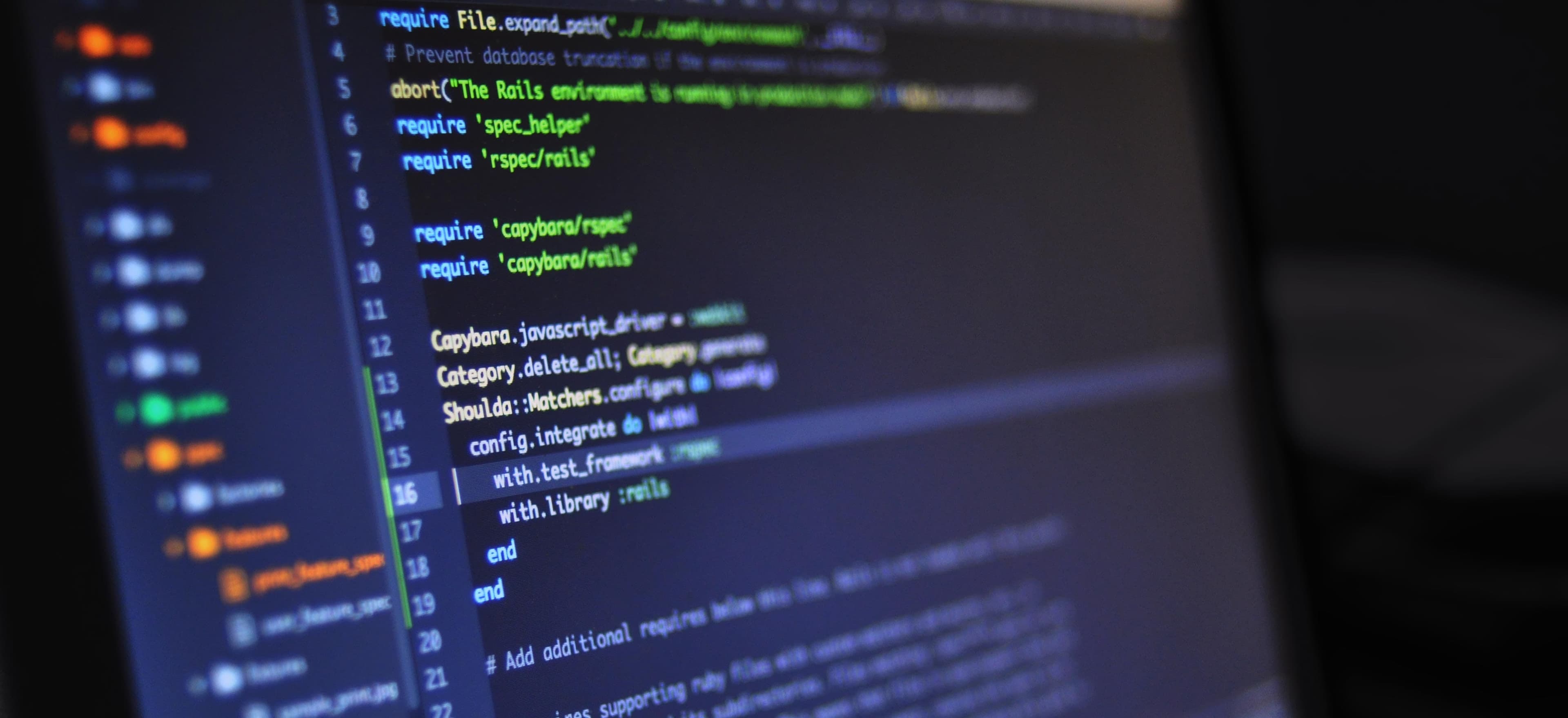
- Published on
Understanding Java's Biological Data Processing Capabilities
Java has become a staple in many areas of software development, and its significance spans various fields, including bioinformatics. The ability to process and analyze biological data efficiently is crucial for ongoing scientific research and understanding complex biological systems. In this blog post, we will explore how Java can be utilized for biological data processing, its advantages, and a few practical examples.
Why Java for Biological Data Processing?
Java’s elegance stems not only from its portability but also from its robust libraries and frameworks. Here are a few reasons why Java is an ideal choice:
-
Cross-platform Compatibility: Java runs on any machine that has the Java Virtual Machine (JVM) installed. This flexibility is crucial when dealing with diverse computing environments often found in biological research.
-
Rich Ecosystem: Java has a wealth of libraries such as Apache Commons Math, BioJava, and JFreeChart that facilitate complex mathematical computations, data visualization, and handling of biological data formats.
-
Object-Oriented Programming: This paradigm allows scientists to model biological entities more naturally, leading to code that is easier to understand and maintain.
-
Concurrency Support: Java's built-in support for multithreading can significantly speed up data processing tasks, which is particularly important when dealing with large datasets typical in bioinformatics.
Getting Started with Bioinformatics in Java
To delve deeper into bioinformatics applications in Java, let us review some code snippets that illustrate how data can be processed. We will focus on DNA sequence analysis, which is a common task in biological information processing.
Analyzing DNA Sequences
Below is a simple Java program that reads a DNA sequence and counts the occurrences of each nucleotide type (A, T, C, G).
import java.util.HashMap;
public class DNAAnalyzer {
public static void main(String[] args) {
String dnaSequence = "ATGCTAGCTAGCATCGATGCTAGCTAGCTAGC";
HashMap<Character, Integer> nucleotideCount = analyzeDNA(dnaSequence);
System.out.println(nucleotideCount);
}
public static HashMap<Character, Integer> analyzeDNA(String dna) {
HashMap<Character, Integer> counts = new HashMap<>();
for (char nucleotide : dna.toCharArray()) {
counts.put(nucleotide, counts.getOrDefault(nucleotide, 0) + 1);
}
return counts;
}
}
Explanation of the Code:
- Input DNA Sequence: The DNA sequence is represented as a string.
- HashMap: A HashMap is employed to store the count of each nucleotide. The keys are the nucleotide characters ('A', 'T', 'G', 'C'), while the values represent their counts.
- Iterating Through the Sequence: The program converts the DNA string into a character array and iterates through it, incrementing the count for each nucleotide found.
This simple analysis can be expanded to include functionalities like GC content calculation, reverse complement generation, or even searching for specific motifs, reflecting the modularity of Java.
Functionalities to Implement
Given the simplicity of the example above, we can build upon this foundation to craft more advanced functionalities that could be useful in biological research.
GC Content Calculation
The GC content is often an essential parameter in genomics as it can affect the properties of a segment of DNA:
public static double calculateGCContent(String dna) {
int gCount = 0;
int cCount = 0;
for (char nucleotide : dna.toCharArray()) {
if (nucleotide == 'G') gCount++;
if (nucleotide == 'C') cCount++;
}
return (double)(gCount + cCount) / dna.length() * 100.0; // Return GC content as percentage.
}
Why GC Content Matters
GC content has implications for the stability of DNA molecules due to the presence of three hydrogen bonds between G and C, as opposed to the two bonds between A and T. It can influence melting temperature, gene expression, and more.
Visualizing Bioinformatics Data
Data visualization is a fundamental aspect of bioinformatics, allowing researchers to interpret and communicate their findings effectively. Java provides various libraries for charting, among which JFreeChart is a popular choice. Here’s a simple example of how you could visualize the nucleotide counts.
A Basic Bar Chart of Nucleotide Counts
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.data.category.DefaultCategoryDataset;
import javax.swing.*;
public class NucleotideChart extends JFrame {
public NucleotideChart(HashMap<Character, Integer> nucleotideCount) {
DefaultCategoryDataset dataset = new DefaultCategoryDataset();
for (Character nucleotide : nucleotideCount.keySet()) {
dataset.addValue(nucleotideCount.get(nucleotide), "Nucleotides", nucleotide.toString());
}
JFreeChart chart = ChartFactory.createBarChart(
"Nucleotide Counts",
"Nucleotide",
"Count",
dataset
);
ChartPanel chartPanel = new ChartPanel(chart);
setContentPane(chartPanel);
}
public static void main(String[] args) {
HashMap<Character, Integer> counts = analyzeDNA("ATGCTAGCTAGCATCGATGCTAGCTAGCTAGC");
NucleotideChart chart = new NucleotideChart(counts);
chart.setSize(800, 600);
chart.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
chart.setVisible(true);
}
}
What This Code Does
- Dataset Creation: A
DefaultCategoryDataset
is utilized to hold the nucleotide counts for easy plotting. - Chart Creation: We create a bar chart using JFreeChart to represent the nucleotide counts visually.
- Displaying the Chart: Utilizing a JFrame, the chart is displayed, allowing for immediate visual feedback.
A Final Look
Java’s capabilities for biological data processing are vast and versatile. From simple nucleotide counting to complex statistical analysis, the language provides a strong foundation for bioinformatics applications. The use of Java libraries can greatly improve the efficiency and readability of code, which is invaluable in research settings.
For those interested in a deeper dive into biochemical reactions, check out the article titled Das Wunderwerk Leben: Biochemische Reaktionen entschlüsselt. This article sheds light on biochemical processes that can further enhance your understanding of biological systems and algorithms.
In the ever-evolving realm of bioinformatics, Java remains a dependable ally for scientists and developers alike, enhancing our understanding of the biological world through data.