Java Command Line Tools: Solving Common Issues Effectively
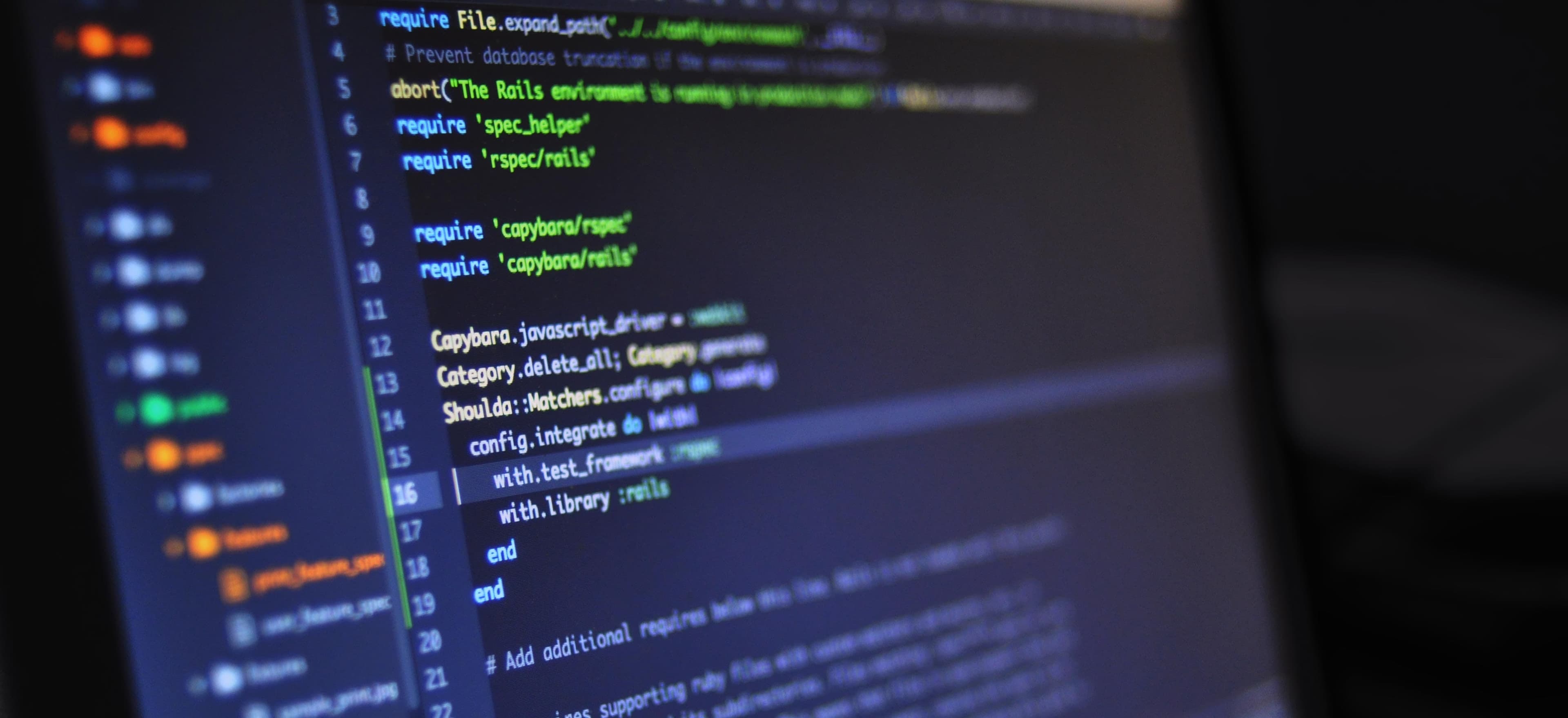
- Published on
Java Command Line Tools: Solving Common Issues Effectively
Java is a powerhouse in the world of programming, powering everything from mobile apps to enterprise systems. One of the most powerful aspects of Java is its suite of command line tools that can help developers manage their applications more effectively. However, even seasoned developers can run into issues. In this blog post, we will dive deep into some common pitfalls associated with Java command line tools and how to resolve them, ensuring you can navigate the command line landscape with confidence.
Understanding Java Command Line Tools
Java provides several command line tools that assist developers in compiling, running, and managing applications. Some of the most commonly used tools include:
- javac: The Java compiler, used to convert Java code into bytecode.
- java: The Java Runtime Environment (JRE) launcher, responsible for running Java applications.
- javadoc: A tool that generates documentation from Java source code.
- jar: The tool used to create Java Archive files, packaging multiple Java classes and resources into a single file.
Understanding how to utilize these tools properly is essential. Let’s explore common issues and their resolutions in depth.
1. Compilation Errors with javac
Issue: Missing or Incorrect Classpath
One of the most frequently encountered issues during Java development is related to the javac
command, especially concerning the classpath.
Example Command:
javac -cp . MyClass.java
Problem: If your class files are located in a different directory or if you're using external libraries, your command might fail due to a missing classpath.
Solution: Always ensure that you specify the correct classpath. Use the -cp
or -classpath
option followed by a list of directories or JAR files that your application depends on.
Why is This Important?
Defining the classpath correctly allows the Java compiler to locate the necessary classes and resources, preventing compilation failures.
2. Runtime Errors with java
Issue: ClassNotFoundException
When running your Java application using the java
command, encountering a ClassNotFoundException
is common.
Example Command:
java -cp . MyClass
Problem: This typically happens when the specified class (in this case, MyClass
) is not in the specified classpath.
Solution: Just like with javac
, ensure your classpath is correctly set. Include all directories and JAR files.
Corrected Command:
java -cp .:lib/somelibrary.jar MyClass
Why it Matters
Properly setting the classpath allows the Java Virtual Machine (JVM) to find the classes it needs to execute, which is fundamental for your application to run successfully.
3. Packaging Issues with jar
Issue: Improper Manifest File
When creating JAR files with the jar
command, an improperly formatted MANIFEST.MF
file can lead to issues, especially if you're trying to run the JAR as an executable.
Example Command:
jar cfm MyApplication.jar MANIFEST.MF MyClass.class
Problem: If the manifest file does not correctly specify the Main-Class attribute, you won't be able to execute the JAR file.
Solution: Ensure your MANIFEST.MF
file includes the right format.
Manifest-Version: 1.0
Main-Class: MyClass
Importance of Proper Packaging
A well-defined manifest file is essential for guiding the JVM to the entry point of your application, making execution smooth and error-free.
4. Documentation with javadoc
Issue: Incomplete or Missing Documentation
While generating documentation with javadoc
, you might find that not all your classes or methods are documented as expected.
Example Command:
javadoc -d docs MyClass.java
Problem: This can occur if you haven’t used the proper Javadoc comments in your code.
Solution: Ensure you use /** ... */
comment blocks for documenting your classes, methods, and fields.
Example Code:
/**
* This class represents a simple example.
*/
public class MyClass {
/**
* This method performs a simple task.
*/
public void myMethod() {
// Implementation goes here
}
}
Why This Matters
Comprehensive documentation not only improves code readability but also helps other developers (and future you) understand the purpose and functionality of your code, significantly reducing onboarding time.
5. Environment Issues
Issue: JAVA_HOME Not Set
The JAVA_HOME
environment variable is crucial for Java applications and tools. If it’s not set correctly, you may run into numerous issues.
Problem: Commands fail because the tools cannot find the Java installation.
Solution: Set the JAVA_HOME
variable to point to your JDK installation. On Unix/Linux, you can add the following line to your .bash_profile
or .bashrc
file:
export JAVA_HOME=/path/to/your/jdk
Why is JAVA_HOME
Necessary?
Properly setting the JAVA_HOME
variable allows other applications and tools to locate your JDK installation, ensuring smooth execution.
Bonus: Referencing Additional Resources
If you're looking to enhance your skills further, I highly recommend checking out Mastering Bash: Overcoming Common Command Line Challenges. This article provides valuable insights into command line usage that can also be applied when working with Java command line tools.
The Last Word
Navigating the command line can sometimes be daunting, especially when working with powerful languages like Java. By understanding common issues and their solutions related to Java command line tools, you can enhance your efficiency and confidence as a developer. Remember, the key to mastering these tools lies in correct configuration and understanding their functionality.
Take some time to experiment with these commands, and always make sure to refer back to your project’s requirements and libraries. With practice, you'll find that the command line is not just a tool but an extension of your programming capabilities. Happy coding!