Tackling Color Conversion Issues in Java Graphics
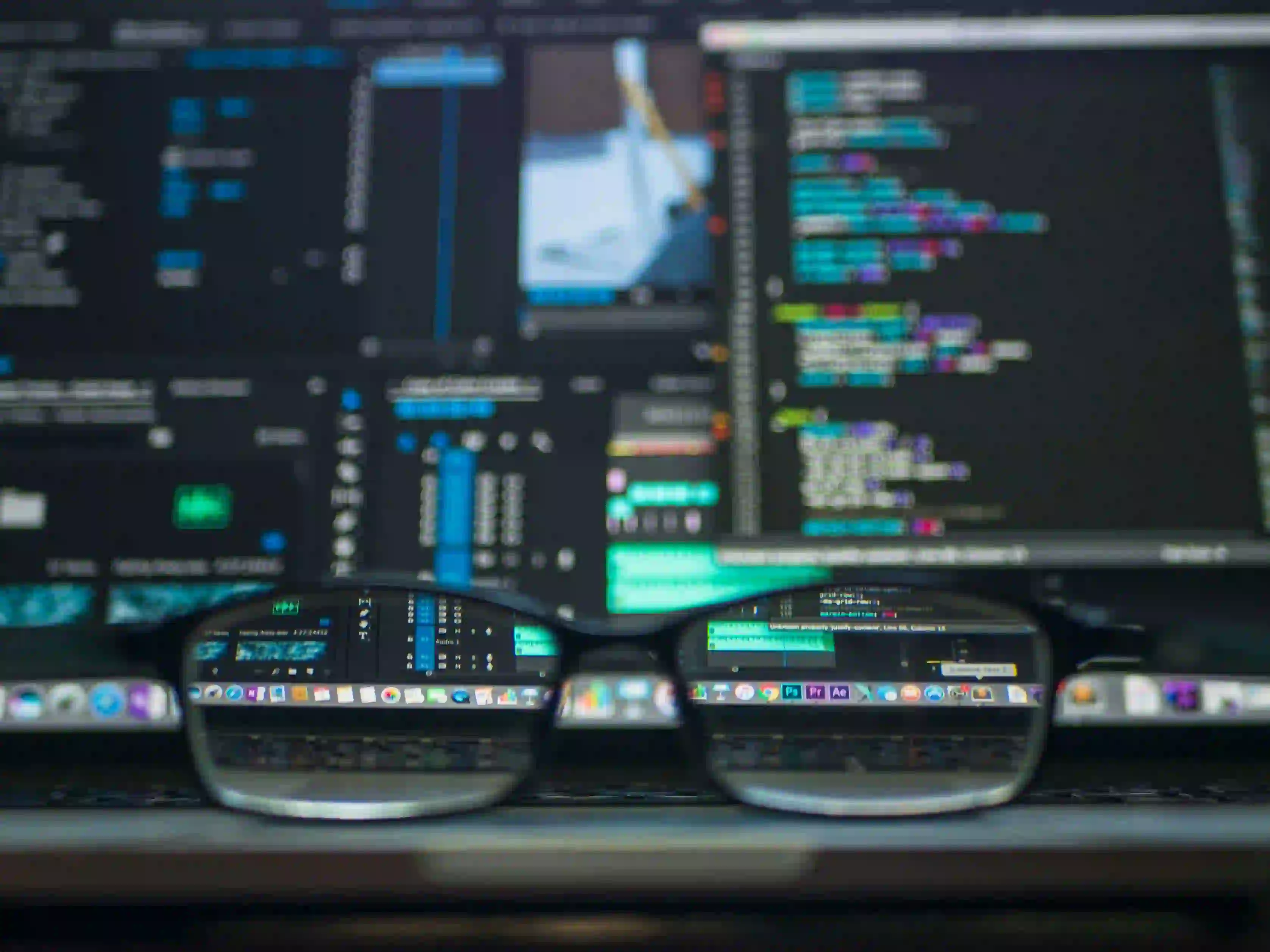
Tackling Color Conversion Issues in Java Graphics
Java developers working in the field of graphics often encounter the challenging task of color conversion. Whether you're developing a game, a graphical user interface, or processing images, understanding how to manipulate colors accurately can be essential to achieving the desired visual output.
Many developers might feel overwhelmed by RGB, CMYK, HSL, and other color spaces. However, by mastering color conversion in Java, you can ensure your applications exhibit true to life colors.
In this blog post, we'll explore the intricacies of color conversion in Java, with a particular focus on RGB conversions, potential pitfalls, and strategies to mitigate them. For additional context on a related topic, you may refer to the article titled Mastering Color Matching: Solving RGB Component Loss.
Understanding Color Models
Before we delve into the remedies for color conversion issues, let’s briefly outline the common color models.
-
RGB (Red, Green, Blue): This additive color model combines red, green, and blue light in various ways to reproduce a broad spectrum of colors. It is widely used in digital screens.
-
CMYK (Cyan, Magenta, Yellow, Black): This subtractive color model is primarily utilized in color printing. It works by masking colors on a white background.
-
HSL (Hue, Saturation, Lightness): This model represents colors in a cylindrical format, making it easier to adjust colors in terms of human perception.
Understanding these models sets the groundwork for managing color conversion effectively.
Common Pitfalls in Color Conversion
1. RGB Component Loss
One of the primary challenges developers face during color conversion is RGB component loss. When converting RGB values to another color model (or when storing them), it's easy to drop or inaccurately transform the components, leading to undesirable visual outcomes.
In the article referenced earlier, Mastering Color Matching: Solving RGB Component Loss, the author discusses practical examples highlighting this issue.
2. Color Range Compatibility
Different color spaces may have varying ranges. For instance, while RGB can represent 256 levels per channel (0-255), other spaces like HSL may not necessarily align with those values well. Color values exceeding the valid range can yield inaccurate results and visual artifacts.
Java Color Conversion Techniques
Now let's roll up our sleeves and look at how to effectively tackle these issues within Java.
RGB to HSL Conversion
Let's start with converting RGB values to HSL. Here's a function that accomplishes this task.
public class ColorConverter {
public static float[] rgbToHsl(int r, int g, int b) {
// Normalize RGB values to the range [0, 1]
float rNorm = r / 255f;
float gNorm = g / 255f;
float bNorm = b / 255f;
// Compute the maximum and minimum RGB values
float max = Math.max(rNorm, Math.max(gNorm, bNorm));
float min = Math.min(rNorm, Math.min(gNorm, bNorm));
float h, s, l = (max + min) / 2;
// Calculate Saturation
if (max == min) {
h = s = 0; // achromatic
} else {
float d = max - min;
s = l > 0.5 ? d / (2 - max - min) : d / (max + min);
// Calculate Hue
if (max == rNorm) {
h = (gNorm - bNorm) / d + (gNorm < bNorm ? 6 : 0);
} else if (max == gNorm) {
h = (bNorm - rNorm) / d + 2;
} else {
h = (rNorm - gNorm) / d + 4;
}
h /= 6;
}
return new float[]{h, s, l};
}
}
Commentary
- In this method, we normalize the RGB values to the range of [0, 1] to perform calculations seamlessly.
- We compute the maximum and minimum values among the RGB components to derive the
hue
,saturation
, andlightness
. - This implementation is crucial for applications that require color adjustment based on user input or design requirements.
HSL to RGB Conversion
Following the conversion from RGB to HSL, we can also convert HSL back to RGB.
public static int[] hslToRgb(float h, float s, float l) {
float r, g, b;
if (s == 0) {
r = g = b = l; // achromatic
} else {
float q = l < 0.5 ? l * (1 + s) : l + s - (l * s);
float p = 2 * l - q;
r = hueToRgb(p, q, h + 1f / 3);
g = hueToRgb(p, q, h);
b = hueToRgb(p, q, h - 1f / 3);
}
return new int[]{(int)(r * 255), (int)(g * 255), (int)(b * 255)};
}
private static float hueToRgb(float p, float q, float t) {
if (t < 0) t += 1;
if (t > 1) t -= 1;
if (t < 1f / 6) return p + (q - p) * 6 * t;
if (t < 1f / 2) return q;
if (t < 2f / 3) return p + (q - p) * (2f / 3 - t) * 6;
return p;
}
Commentary
- This function operates similarly but in reverse, taking calculated HSL values and converting them back into RGB values.
- The helper function
hueToRgb
assists in computing the RGB components based on the parameters derived from HSL values. - This two-way conversion is beneficial when you need to switch between different color models, particularly in design applications where user input can vary across different color representations.
Handling Component Loss
As we've noted earlier, RGB component loss can occur during conversions. To mitigate this, it's essential to ensure that your color values are within the acceptable ranges at all times.
Verification Function
We can incorporate a verification step after conversion to ensure our values remain accurate.
public static int clamp(int value) {
return Math.max(0, Math.min(255, value));
}
You can use this clamp function after color conversions to prevent any undesired outputs.
Example of Full Cycle
Here's how we can use these conversions together in a full cycle, demonstrating value integrity:
public static void main(String[] args) {
int r = 150, g = 75, b = 200; // Sample RGB values
float[] hsl = rgbToHsl(r, g, b);
System.out.printf("Converted HSL: H: %.2f, S: %.2f, L: %.2f%n", hsl[0], hsl[1], hsl[2]);
int[] rgbBack = hslToRgb(hsl[0], hsl[1], hsl[2]);
System.out.printf("Converted back RGB: R: %d, G: %d, B: %d%n", clamp(rgbBack[0]), clamp(rgbBack[1]), clamp(rgbBack[2]));
}
Commentary
- The above code demonstrates the entire cycle of conversion and reconversion, ensuring that at each step, values remain within prescribed limits.
- This can serve as a foundation for building more complex color handling functionalities in your applications.
Wrapping Up
Tackling color conversion issues in Java graphics can seem challenging at first glance. However, by understanding color models and utilizing effective conversion methods, you can manage colors with greater precision. The importance of color integrity cannot be overstated, as it directly impacts the quality and usability of graphical applications.
For further insights into RGB component loss and color matching strategies, do check out the article Mastering Color Matching: Solving RGB Component Loss.
With careful handling and a bit of practice, you'll soon be adept at converting colors and ensuring that your Java applications shine with vivid accuracy. Happy coding!
Feel free to follow this structure and modify the sample code to better fit your needs!