Java DevOps: Mastering Ansible for Java Deployments
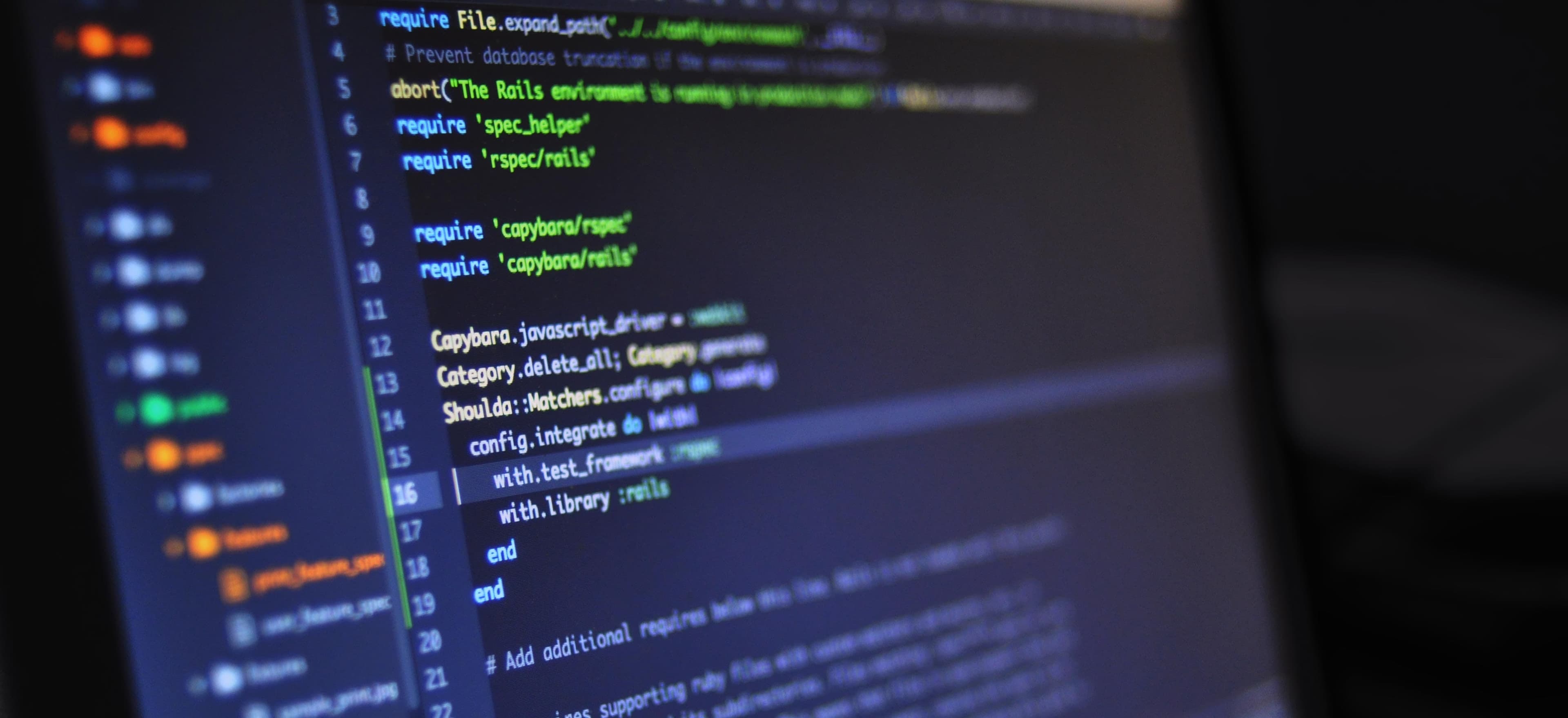
- Published on
Java DevOps: Mastering Ansible for Java Deployments
In today's fast-paced software development landscape, integrating DevOps practices is paramount for successful and efficient deployments. As we venture into the intersection of Java applications and Ansible—an open-source automation tool—we uncover the nuanced yet powerful synergy that enhances the deployment process.
Table of Contents
- Understanding Ansible Basics
- Setting Up Your Environment
- Ansible Playbooks for Java Deployments
- Managing Dependencies with Maven
- Continuous Integration and Delivery (CI/CD) Pipeline
- Conclusion
Understanding Ansible Basics
Ansible is an open-source tool that automates software provisioning, configuration management, and application deployment. What makes Ansible truly special is its simplicity and ease of use. With Ansible, you can define a configuration once and reuse it multiple times, streamlining the entire deployment process.
Ansible uses Playbooks written in YAML format, which describe the desired state of your system. For Java developers, employing Ansible can significantly reduce the complexity of application deployment tasks.
Setting Up Your Environment
Before diving into the intricacies of Ansible and its application in Java deployments, let’s set up our environment:
-
Install Ansible: You can install Ansible on various platforms. If you're using Ubuntu, execute the following command:
sudo apt update sudo apt install ansible
-
Install Java and Maven: Ensure that Java Development Kit (JDK) and Maven are installed on your machine. Use these commands:
sudo apt install openjdk-11-jdk sudo apt install maven
-
Verify Installations: Check the installations by running these commands:
java -version mvn -version ansible --version
Ansible Playbooks for Java Deployments
Ansible playbooks form the backbone of your automation scripts. Let's look at writing a simple playbook to deploy a Java application.
- name: Deploy Java Application
hosts: java_servers
become: yes
vars:
app_name: my-application
app_version: 1.0.0
app_directory: /opt/{{ app_name }}
tasks:
- name: Install Java
apt:
name: openjdk-11-jdk
state: present
- name: Create application directory
file:
path: "{{ app_directory }}"
state: directory
- name: Copy application files
copy:
src: /local/path/to/{{ app_name }}-{{ app_version }}.jar
dest: "{{ app_directory }}/{{ app_name }}.jar"
- name: Start the Java application
java:
jar: "{{ app_directory }}/{{ app_name }}.jar"
state: started
Commentary on the Code Snippet
- Hosts Definition: We specify
hosts: java_servers
, indicating that the playbook will run on a group of servers defined in our inventory. - Package Installation: The
apt
module ensures that Java is installed on our target machine. This prevents instances where the Java runtime is missing. - Directory Creation: The
file
module creates our application directory, ensuring that there is a place to deploy our artifact. - File Transfer: The
copy
module transfers the JAR file from the local machine to the server—an essential step, as it enables us to deploy the necessary code. - Application Startup: By using the
java
module, we can directly run our JAR file.
Managing Dependencies with Maven
Maven plays a pivotal role in building Java applications. It manages dependencies and handles the build process seamlessly. Here's how you can integrate Maven into your Ansible playbook.
Add a task to download Maven dependencies:
- name: Download dependencies with Maven
shell: mvn clean install
args:
chdir: "{{ app_directory }}"
Commentary on the Maven Task
- Shell Command: We are executing a shell command within a specified directory. The
chdir
argument ensures that Maven operates in the correct context. This responsible set up allows you to build your Java project without any manual intervention, promoting reliability.
Continuous Integration and Delivery (CI/CD) Pipeline
Integrating Ansible with a CI/CD pipeline can drastically enhance your deployment efficiency. Tools like Jenkins, GitLab CI/CD, or GitHub Actions can trigger Ansible playbooks upon successful builds.
For instance, you can configure a Jenkins pipeline to run your Ansible playbook as a post-build step:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
ansiblePlaybook playbook: 'deploy.yml'
}
}
}
}
The Bottom Line
Mastering Ansible for Java deployments not only simplifies your workflows but also empowers your team to deliver applications faster and with fewer errors. As you implement these practices, remember that automating your deployment process is not just about writing scripts but about making your team more efficient and responsive to changes.
For those preparing for a deeper dive into technological skillsets, consider reviewing the article How to Ace Ansible Interview Questions?, which could provide you with valuable insights into mastering Ansible.
Further Reading
- Ansible Documentation: Comprehensive guide to all Ansible features.
- Java Official Documentation: To keep up with the latest Java developments.
By embracing the collaboration of Java and Ansible, you're set on the path towards a more automated, efficient, and scalable deployment process. Happy coding and automating!
Checkout our other articles