Java Simplified: Tips to Overcome Code Comprehension Hurdles
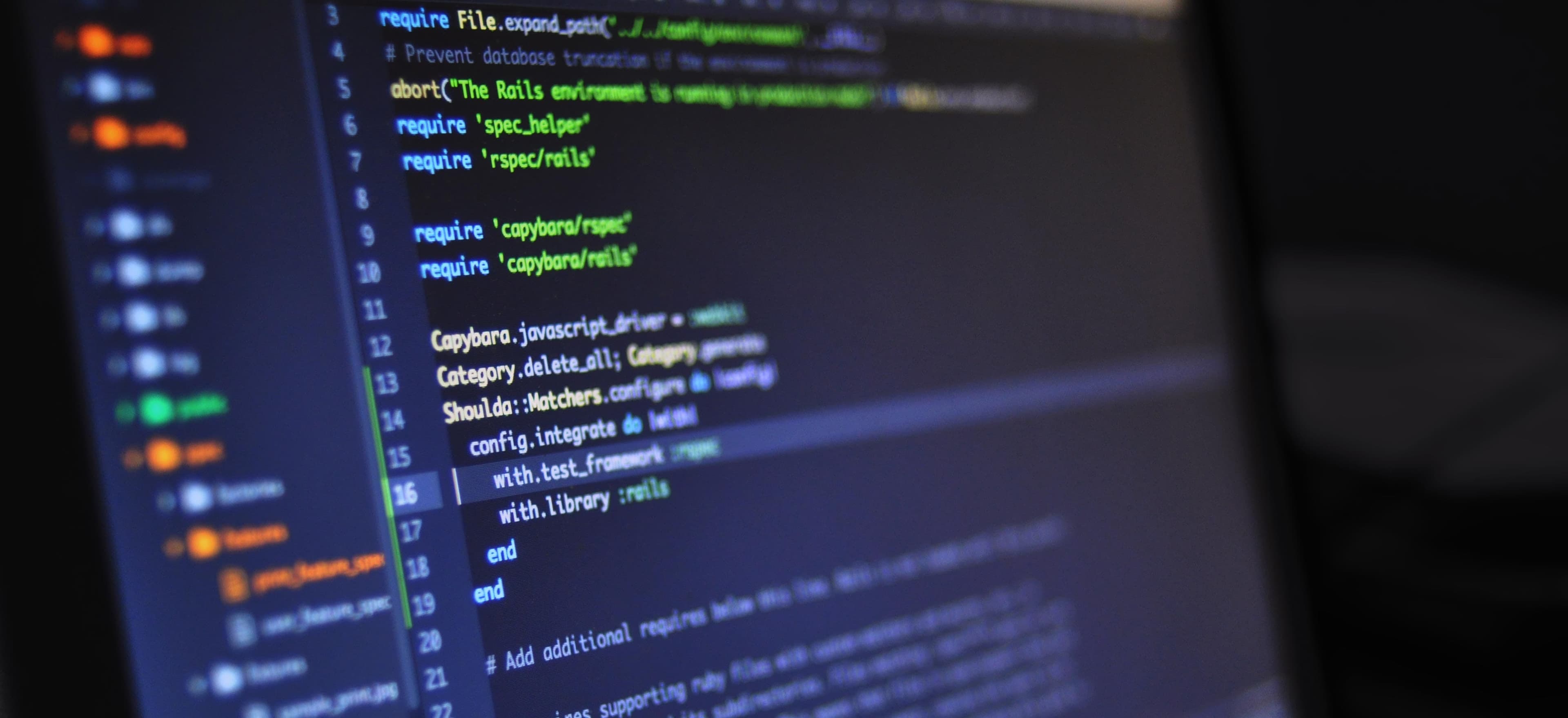
- Published on
Java Simplified: Tips to Overcome Code Comprehension Hurdles
Java programming may sometimes seem daunting, especially for beginners. Yet, like any intricate field, breaking it down into manageable components can turn complexity into clarity. This article serves as a guide to help developers navigate the multifaceted world of Java by providing insights and strategies for overcoming common comprehension challenges. This is akin to how chemistry education tackles abstract concepts, as discussed in Chemie leicht gemacht: Tipps gegen Verständnisprobleme.
Understanding Java doesn’t require you to master every nuance immediately. Instead, it’s about building a strong foundation, recognizing patterns, and employing practical strategies.
Understanding the Basics: Java Syntax and Structure
Before diving deep into complex code snippets, it is essential to grasp the basic syntax and structure of Java. Java is an object-oriented programming language with a straightforward structure. A simple Java program will look something like this:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why This Code?
- Class Declaration: The
public class HelloWorld
line defines a class namedHelloWorld
. Java is class-based, emphasizing the encapsulation of data and behavior. - Main Method:
public static void main(String[] args)
serves as the entry point for any Java application. No matter how complex your program is, it always starts executing from the main method. - Output Statement:
System.out.println("Hello, World!");
is used to print messages to the console. Understanding how to leverageSystem.out
is fundamental in debugging and providing feedback.
Familiarizing yourself with such basic concepts creates a comfort zone, making it easier to tackle more advanced topics.
Approach Code in Chunks
When faced with lengthy codes, it can be helpful to break them down into smaller, manageable sections. This technique is particularly useful to make sense of complex Java applications. Consider this complete example:
Full Java Example
import java.util.ArrayList;
public class Student {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
public class School {
private ArrayList<Student> students;
public School() {
students = new ArrayList<>();
}
public void addStudent(Student student) {
students.add(student);
}
public void displayStudents() {
for (Student student : students) {
System.out.println("Student Name: " + student.getName() + ", Age: " + student.getAge());
}
}
public static void main(String[] args) {
School school = new School();
school.addStudent(new Student("Alice", 10));
school.addStudent(new Student("Bob", 12));
school.displayStudents();
}
}
Why This Breakdown?
- Class Definitions: Notice how we defined two classes:
Student
andSchool
. This separation follows the object-oriented principle of encapsulation. - Constructor and Methods: In
Student
, we utilize a constructor to initialize object state and provide getter methods to access private variables. - ArrayList Usage: The
School
class uses anArrayList
to storeStudent
objects dynamically. UnderstandingArrayList
's functionality is crucial for managing collections in Java. - Displaying Information: The
displayStudents
method iterates through the list of students and outputs their details, which is ideal for debugging and ensuring that the program executes as expected.
By dissecting code into logical segments, it becomes more manageable to grasp its functionality.
Leverage Comments and Documentation
One of the best practices in programming is to document your code using comments. As systems grow in complexity, so does the importance of clear comments.
Sample Code with Comments
public class Calculator {
// Adds two integers and returns the result
public int add(int a, int b) {
return a + b; // Returning the sum of a and b
}
// Subtracts second integer from the first
public int subtract(int a, int b) {
return a - b; // Returning the difference of a and b
}
}
Why Commenting Matters?
- Code Clarity: Comments explain the purpose of methods and significantly enhance code readability.
- Future Reference: As you revisit your code after some time, comments provide context that may not be immediately obvious.
- Collaboration: They also aid others in understanding your work, making collaborative projects more seamless.
Embrace the Power of Resources
There are countless resources available to assist Java developers. From official documentation to community forums like Stack Overflow, tapping into the wealth of shared knowledge can propel your learning.
Recommended Resources
- Oracle Java Documentation
- Java Code Geeks - Offers tutorials, examples, and insights.
- GeeksforGeeks Java Programming Language - A comprehensive resource for understanding Java.
Engaging with these resources enables you to approach problems from different angles, deepening your understanding.
Practice Regularly
In programming, practice is key. Regularly engaging in coding challenges, contributing to open-source projects, or building personal projects can solidify your knowledge.
Suggested Practice Platforms
- LeetCode - Engage with coding challenges.
- HackerRank - Participate in Java-specific challenges.
- Codecademy - Interactive courses designed to teach Java concepts.
Key Takeaways
Understanding Java programming isn't an insurmountable challenge. By breaking down code, utilizing comments, leveraging resources, and practicing regularly, you can build your confidence and comprehension. Much like the strategies discussed for overcoming barriers in understanding chemistry as covered in Chemie leicht gemacht: Tipps gegen Verständnisprobleme, applying a structured approach in programming can go a long way.
With these strategies, you are now equipped to face Java challenges head-on. Continue to explore, practice, and engage with the community—your journey into Java is just beginning!
Checkout our other articles