Java Solutions for Managing Medical Data in Emergencies
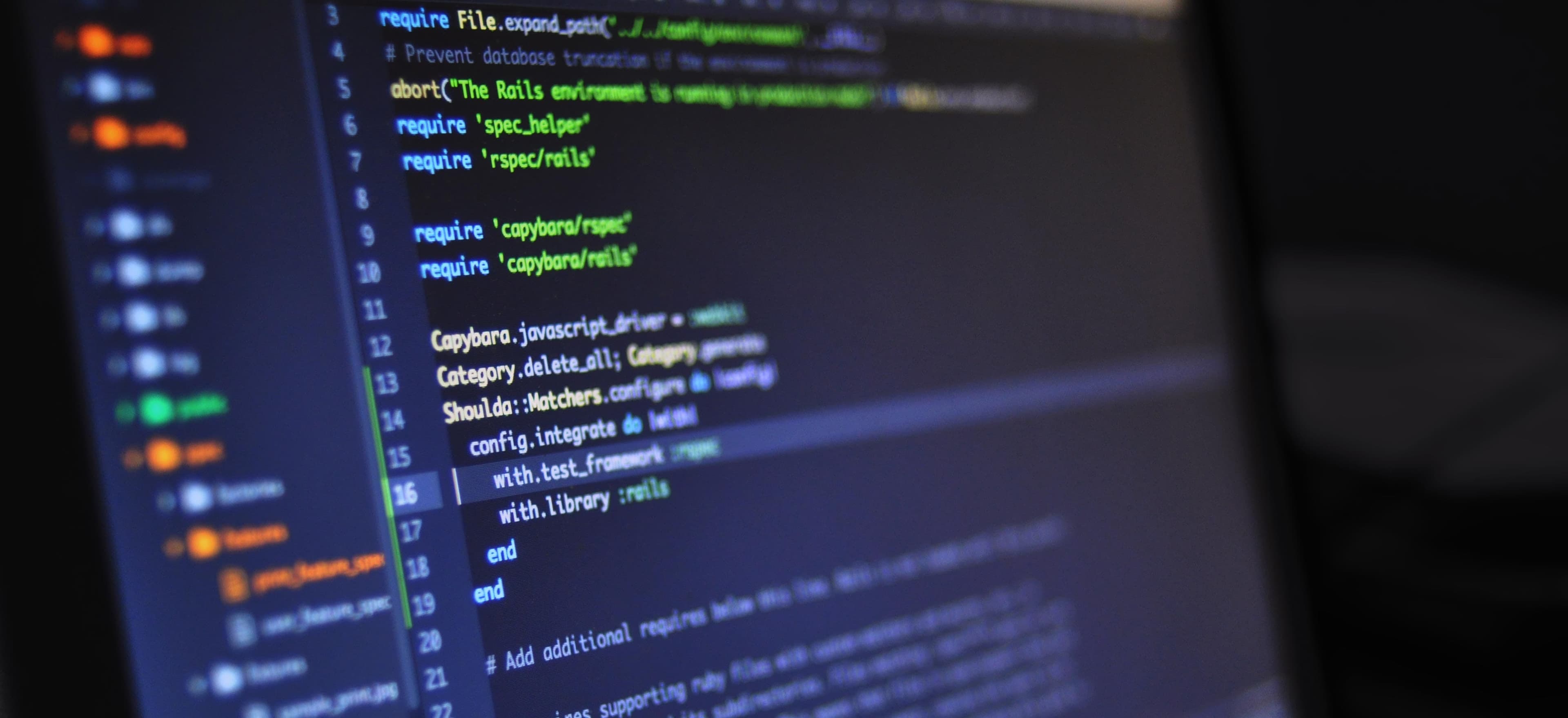
- Published on
Java Solutions for Managing Medical Data in Emergencies
In times of crisis, having reliable and efficient systems to manage medical data can be a lifesaver. Emergency situations demand quick access to accurate information. As more organizations and individuals turn toward learning programming languages like Java to solve these pressing issues, this post will explore how Java can help in managing medical data during emergencies.
Throughout this article, we will delve deep into various Java-based strategies, enabling you to create robust applications that handle medical data efficiently. We will look at code examples that highlight best practices and provide real-world context to each solution.
Why Use Java for Medical Data Management?
Java is a powerful and versatile language that has been around for over two decades. Here are several reasons why Java is particularly suited for managing medical data in emergencies:
- Platform Independence: Java applications can run on any device that has the Java Runtime Environment (JRE) installed, making it easier to deploy on various systems.
- Robust Libraries: Java offers an extensive set of libraries, including powerful data structures and algorithms necessary for managing complex medical data.
- Strong Community Support: With a large community of developers, finding help or resources is easier. Code snippets, libraries, and frameworks can be found with just a quick search.
First Step: Storing Medical Data
A critical component of any medical data management system is effective data storage. Here’s a simple implementation using Java’s ArrayList
to store patient data.
import java.util.ArrayList;
public class Patient {
private String name;
private int age;
private String medicalHistory;
public Patient(String name, int age, String medicalHistory) {
this.name = name;
this.age = age;
this.medicalHistory = medicalHistory;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getMedicalHistory() {
return medicalHistory;
}
}
public class EmergencyDataManagement {
private ArrayList<Patient> patients;
public EmergencyDataManagement() {
patients = new ArrayList<>();
}
public void addPatient(Patient patient) {
patients.add(patient);
}
public ArrayList<Patient> getPatients() {
return patients;
}
}
Commentary on the Code
In the above example, we define a Patient
class, which encapsulates three important attributes: name, age, and medical history. The EmergencyDataManagement
class manages an ArrayList
of patients.
Using an ArrayList
is beneficial for several reasons:
- Dynamic Size: It automatically adjusts its size when adding or removing patients.
- Versatility: Easy iteration capabilities make retrieving patient data straightforward.
This framework sets a foundation for adding more functionalities, such as searching or filtering patients based on certain criteria.
Second Step: Searching for Medical Information
In an emergency, being able to quickly search through medical records is crucial. Consider the following method added to the EmergencyDataManagement
class to allow searching for a patient by name:
public Patient searchPatientByName(String name) {
for (Patient patient : patients) {
if (patient.getName().equalsIgnoreCase(name)) {
return patient;
}
}
return null; // Patient not found
}
Commentary on the Code
The searchPatientByName
method iterates through the list of patients, comparing the input name with the names stored in the Patient
objects.
Why is this important?
- Speed: Quickly find patients when time is of the essence.
- Simplicity: Employs a straightforward linear search, easy to understand for beginners.
While this implementation is simple, you could enhance efficiency by using more advanced data structures like HashMaps for quicker lookups if your patient list expands significantly.
Third Step: Making Medical Data Accessible
In emergency situations, sharing medical data with authorized personnel securely is vital. Here’s an example of how you could handle this using JSON format for data serialization:
import com.google.gson.Gson;
public String serializePatientData() {
Gson gson = new Gson();
return gson.toJson(patients);
}
Commentary on the Code
This code leverages the Gson library to convert an ArrayList
of Patient
objects into a JSON string. JSON is lightweight and easy to parse, making it a preferred format for data interchange between systems.
Why Use JSON?
- Interoperability: Many different systems support JSON, making it easier to share data.
- Simplicity: Human-readable format enhances clarity while maintaining structure.
To use Gson, ensure you include the library in your project dependencies. You may also want to make this JSON serialized data available through a web service for wider access.
Fourth Step: Implementing a Web Service
Speaking of wider access, you can take the next step to make your application even more functional by implementing a simple web service using Spring Boot. Below is an example of creating a RESTful endpoint for medical data:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.*;
@SpringBootApplication
@RestController
@RequestMapping("/patients")
public class MedicalDataApplication {
private EmergencyDataManagement management = new EmergencyDataManagement();
public static void main(String[] args) {
SpringApplication.run(MedicalDataApplication.class, args);
}
@PostMapping("/add")
public String addPatient(@RequestBody Patient patient) {
management.addPatient(patient);
return "Patient added successfully";
}
@GetMapping("/{name}")
public Patient getPatient(@PathVariable String name) {
return management.searchPatientByName(name);
}
}
Commentary on the Code
This code uses Spring Boot to create a RESTful web service for managing patient data. The /patients/add
endpoint allows you to add new patients, while the /{name}
endpoint retrieves a patient by name.
Why Use Spring Boot?
- Ease of Use: Simplifies the setup process for web applications.
- Rapid Development: Provides built-in tools to facilitate quick application development.
Additional Learning Resources
For more comprehensive insights on managing medical scenarios, consider exploring related content. One such resource is the article titled Outfitting Your Kit: Avoiding Common Prepper Medical Mistakes. While it focuses on preparedness, the underlying principles applicable to managing medical data in emergent situations converge closely.
Lessons Learned
In summary, Java provides an excellent platform for developing systems that manage medical data efficiently during emergencies. The importance of rapid data access, organization, and secure transfer cannot be overstated.
From storing patient data to facilitating quick searches and making information accessible via web services, this article has provided a foundation. As you venture into developing solutions using Java, remember that using libraries, frameworks, and best practices can significantly enhance your application's effectiveness.
Take the time to experiment with the code snippets and consider how you might tailor them to fit specific emergency scenarios. With this knowledge, you are now equipped to contribute positively to emergency medical data management.