Java Solutions for Seamless AWS Deployments
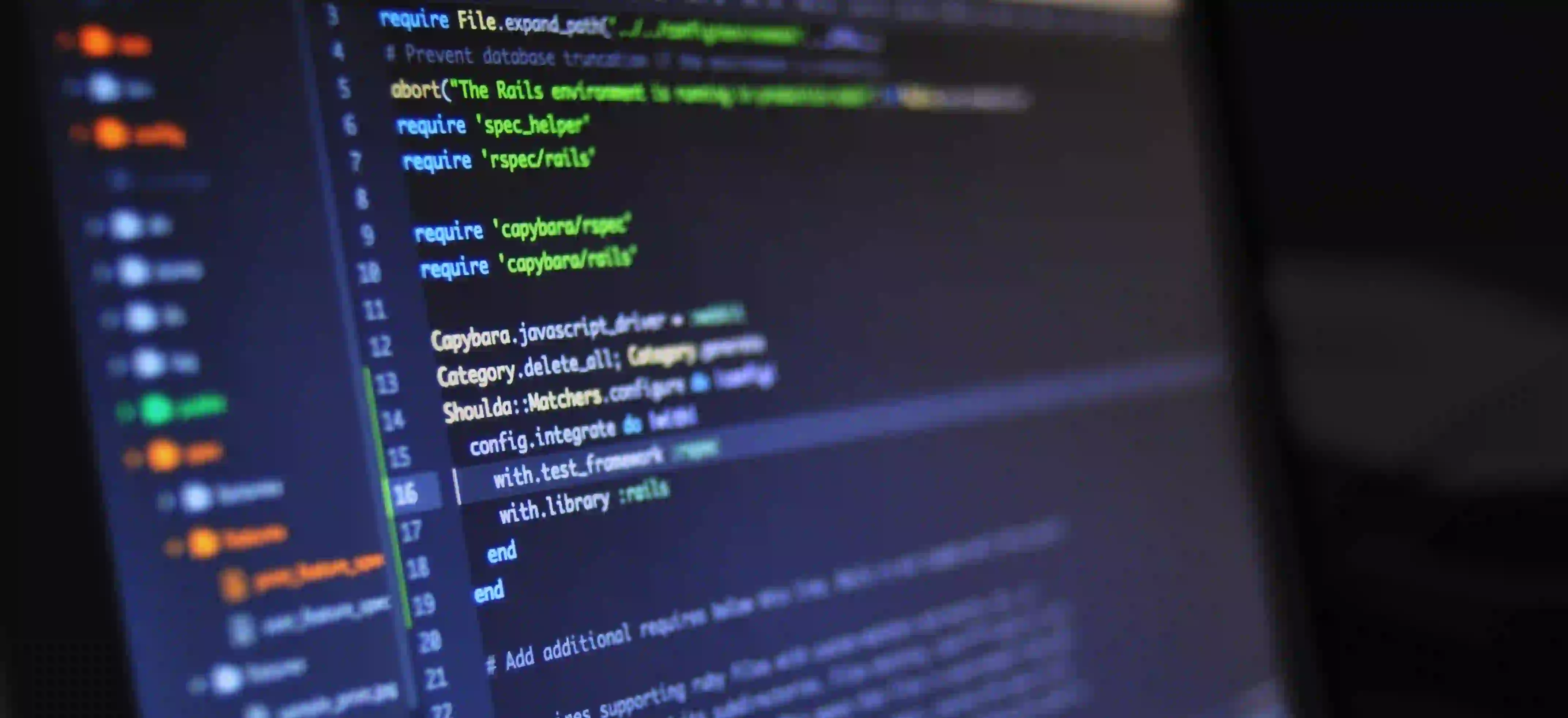
Java Solutions for Seamless AWS Deployments
Deploying Java applications in the cloud has become commonplace thanks to the robust features provided by Amazon Web Services (AWS). However, ensuring smooth and efficient deployments can be a significant challenge. In this blog post, we'll explore practical Java solutions for seamless AWS deployments, leveraging Java's extensive capabilities alongside AWS services.
Why Use AWS for Java Deployments?
AWS is a leading cloud platform that offers scalable, reliable, and low-latency infrastructure. Java, a widely-used programming language, complements AWS with its platform independence and ease of development. The combination of these two technologies can accelerate application deployment, development, and management processes.
Understanding AWS Services for Java Applications
When deploying Java applications on AWS, it’s essential to understand the core services that can enhance your deployment strategy. Some of these include:
- Amazon EC2: Provisioning virtual servers for hosting Java applications.
- AWS Lambda: Running Java code without needing to provision servers.
- Amazon RDS: Managing databases for your Java applications effortlessly.
- Amazon Elastic Beanstalk: Simplifies deploying and scaling Java applications in the cloud.
By understanding these services, Java developers can deploy their applications more efficiently.
Coding with AWS SDK for Java
To interact with AWS services, we need to use the AWS SDK for Java. This SDK simplifies the process of building applications on AWS. Here’s how to get started:
Step 1: Including the AWS SDK
First, ensure you have included the AWS SDK in your project. If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>com.amazonaws</groupId>
<artifactId>aws-java-sdk</artifactId>
<version>1.12.X</version> <!-- Replace with the latest version -->
</dependency>
Step 2: Configuring AWS Credentials
Next, set up your AWS credentials. You can do this by creating a credentials file at ~/.aws/credentials
and adding your access key and secret key:
[default]
aws_access_key_id = YOUR_ACCESS_KEY
aws_secret_access_key = YOUR_SECRET_KEY
Step 3: Interacting with S3 Service
As an example of how to leverage AWS services, let’s look at how to upload files to Amazon S3 using the SDK.
import com.amazonaws.auth.AWSStaticCredentialsProvider;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.amazonaws.services.s3.model.PutObjectRequest;
import java.io.File;
public class S3Uploader {
private final AmazonS3 s3client;
public S3Uploader(String accessKey, String secretKey, String region) {
BasicAWSCredentials awsCredentials = new BasicAWSCredentials(accessKey, secretKey);
s3client = AmazonS3ClientBuilder.standard()
.withRegion(region)
.withCredentials(new AWSStaticCredentialsProvider(awsCredentials))
.build();
}
public void uploadFile(String bucketName, String keyName, File file) {
PutObjectRequest request = new PutObjectRequest(bucketName, keyName, file);
s3client.putObject(request);
System.out.println("File uploaded: " + keyName);
}
}
Why the Code Works
-
Initialization: We create an instance of the AmazonS3 client, specifying our credentials and the desired AWS region. This establishes a connection to AWS resources.
-
Upload Method: The
uploadFile
method takes in a bucket name, key name, and file. The PutObjectRequest is used to perform the upload operation.
By encapsulating this functionality into a class, we conform to good software design practices, promoting reusability and maintainability.
Using AWS Lambda for Java Applications
AWS Lambda allows you to run Java code in response to events. For instance, you could trigger a Lambda function when a file is uploaded to S3. Here's a code snippet for a simple AWS Lambda function in Java:
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class HelloWorldHandler implements RequestHandler<String, String> {
@Override
public String handleRequest(String name, Context context) {
return "Hello, " + name;
}
}
The Advantages of Using AWS Lambda
- Cost-Effective: You pay only for execution time, unlike traditional server-hosted applications.
- Scalability: AWS manages the scaling automatically, allowing for seamless enhancements in workload.
- Event-Driven: Lambda functions can be triggered by other AWS services, making it an excellent solution for microservices architecture.
Learn more about deploying services with AWS Lambda here.
Managing Dependencies with AWS CodeBuild
When working with Java applications, dependency management can be cumbersome. AWS CodeBuild allows you to automate the build process. Setting up CodeBuild with a buildspec.yml
file simplifies this task:
version: 0.2
phases:
install:
runtime-versions:
java: openjdk11
commands:
- mvn install
build:
commands:
- mvn package
artifacts:
files:
- target/*.jar
Explanation of the buildspec.yml
- Install Phase: Sets the Java runtime version and runs the Maven install command to download dependencies.
- Build Phase: Packages the application into a JAR file.
- Artifacts: Defines the output files that will be stored after the build is complete.
Monitoring Your Deployments
Once your Java application is deployed on AWS, it’s crucial to monitor its performance. AWS CloudWatch provides a comprehensive solution for monitoring applications, collecting metrics, and setting alarms.
You can integrate CloudWatch into your Java applications using the AWS SDK, allowing you to log specific metrics for analysis.
import com.amazonaws.services.cloudwatch.AmazonCloudWatch;
import com.amazonaws.services.cloudwatch.AmazonCloudWatchClientBuilder;
import com.amazonaws.services.cloudwatch.model.PutMetricDataRequest;
import com.amazonaws.services.cloudwatch.model.MetricDatum;
import java.util.Collections;
public class CloudWatchSender {
private final AmazonCloudWatch cloudWatch;
public CloudWatchSender() {
cloudWatch = AmazonCloudWatchClientBuilder.defaultClient();
}
public void sendData(String namespace, String metricName, double value) {
MetricDatum datum = new MetricDatum()
.withMetricName(metricName)
.withValue(value)
.withUnit(StandardUnit.Count);
PutMetricDataRequest request = new PutMetricDataRequest()
.withNamespace(namespace)
.withMetricData(Collections.singletonList(datum));
cloudWatch.putMetricData(request);
System.out.println("Data sent: " + metricName + " - " + value);
}
}
Why Monitoring Matters
- Insight and Analysis: Monitoring allows for real-time insights into application performance, helping to identify issues before they escalate.
- Cost Management: By tracking resource usage, you can optimize and reduce unnecessary costs incurred during deployment.
Closing Remarks
Deploying Java applications on AWS offers numerous benefits, especially when using the right services and practices. From simplifying file uploads to automating builds, we've covered essential Java solutions that enhance deployment efficiency.
For specific challenges like deployment failures, you may want to check out the article titled Troubleshooting Laravel Deployment in AWS, which provides insight into common issues and their resolutions.
With these best practices and solutions, you can embark on your Java deployment journey on AWS, maximizing the capability of both technologies. Remember, the key to a seamless deployment lies in understanding and leveraging the tools at your disposal. Happy coding!